Generate random numbers and strings in JavaScript
The ability to generate random numbers or alphanumeric strings comes in handy in many situations. You can use it to spawn enemies or food at different locations in the game. You can also use it to suggest random passwords to users or create filenames to save files.
I wrote a tutorial on how to generate random alphanumeric strings in PHP. I said at the beginning of this post that few events are truly random, and the same applies to random number or string generation.
In this tutorial, I will show you how to generate a pseudo-random alphanumeric string in JavaScript.
Generate random numbers in JavaScript
Let's start by generating random numbers. The first method that comes to mind is Math.random()
, which returns a floating point pseudo-random number. The random number will always be greater than or equal to 0 and less than 1.
The distribution of returned numbers in this range is almost uniform, so this method can generate random numbers well without obvious deviation in daily use. The following is the output of ten calls to the Math.random()
method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
Generate a random integer within a range
As you saw in the previous section, Math.random()
will give us a random number in the range of 0 (inclusive) to 1 (exclusive). Suppose we want a random integer in the range 0 (inclusive) to 100 (exclusive). All we need to do here is multiply the original range by 100.
Taking the first output value of the above code snippet as an example, 0.9981169188071801 will become 99.81169188071801 when multiplied by 100. Now, we can use the Math.floor()
method, which will round down and return the largest integer whose value is less than or equal to 99.81169188071801. In other words, it will give us 99.
The following code snippet will loop 10 times to show all these steps applied to different numbers.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
Now that you understand the logic behind multiplication and rounding, we can write a function to generate random integers within the maximum limit.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
What if you want to generate random numbers above a specified minimum but below a maximum?
In this case, you can prepend the minimum value to ensure that the resulting number is at least equal to the minimum value. After that you can simply generate a random number and scale it by max - min
and then add it to the smallest possible value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
Generate cryptographically secure random numbers
TheMath.random()
method is not suitable for generating cryptographically secure random numbers, but the Crypto.getRandomValues()
method can help us here. This method fills the passed array with cryptographically secure pseudo-random numbers. Keep in mind that the algorithm used to generate these random numbers may vary from user agent to user agent.
As I mentioned before, you need to pass an integer-based TypedArray
to the method so that it fills it with random values. The original contents of the array will be replaced. The following code will fill our ten-element array with random integers.
1 2 3 4 5 6 7 |
|
Unit8Array()
The constructor provides us with an array of ten 8-bit unsigned integers. Array values are all initialized to zero.
Once we pass this array to the getRandomValues()
method, the random number values will remain between 0 and 255. You can use other types of arrays to generate different ranges of random numbers. For example, using the Int8Array()
constructor will give us an array of integer values between -128 and 127. Likewise, using Uint16Array()
will give us an array of integer values up to 65,535.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
|
Generate random alphanumeric strings in JavaScript
We will now use the knowledge gained in the previous section to generate a random alphanumeric string in JavaScript.
The concept is very simple. We'll start with a string containing all the required characters. In this example, the string will consist of lowercase letters, uppercase letters, and numbers from 0 to 9. As you may already know, we can access a character at a specific position in a string by passing an index value to the string.
To generate a random alphanumeric string, all we need to do is generate a random number and then access the character at that random index to append it to our random string. The following code snippet wraps it all up in a nice little function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
使用 toString()
生成随机字母数字字符串
我们可以用来生成随机字母数字字符串的另一种方法是对随机生成的数字使用 toString()
方法。 toString()
方法返回一个表示我们指定数值的字符串。此方法接受可选的 radix
参数,该参数指定要表示数字的基数。值为 2 将返回二进制字符串,值为 16 将返回十六进制字符串。该参数默认值为10。最大值可以为36,因为它涵盖了全部26个字母和10个数字。
以下是针对不同 radix
值对此方法进行几次调用的输出:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
您可能已经注意到,随着我们增加 radix
,输出字符串的长度不断减少。在下面的代码片段中,我们将使用上一节中的 max_random_number()
函数来获取随机数。然后,我们将使用 toString()
方法将此随机数转换为字母数字字符串。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
如果您想要更大的字母数字字符串并希望它们具有固定长度(例如 40 个字符或 100 个字符)怎么办?在这种情况下,我们可以创建一个循环,不断附加生成的字符串,直到达到所需的长度。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
最终想法
在本教程中,我们学习了如何在 JavaScript 中生成随机数和字母数字字符串。借助 Math.random()
方法,在 JavaScript 中生成随机整数很容易。我们所要做的就是缩放输出,使其符合我们所需的范围。如果您希望随机数具有加密安全性,您还可以考虑使用 getRandomValues()
方法。
一旦我们知道如何生成随机数,创建随机字母数字字符串就很容易了。我们需要做的就是弄清楚如何将数字转换为字符。我们在这里使用了两种方法。第一个涉及访问预定义字符串中随机数字索引处的字符。如果您想具体了解随机字母数字字符串中应包含的字符,则此技术非常有用。另一种方法涉及使用 toString()
方法将十进制数转换为不同的基数。这减少了对 max_random_number()
函数的调用。
当然还有更多技术可用于生成随机字母数字字符串。这完全取决于您的需求以及您想要的方法的创意程度。
The above is the detailed content of Generate random numbers and strings in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
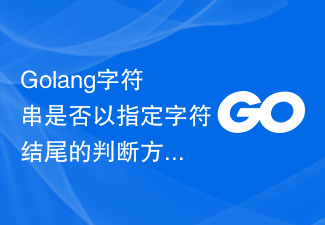
Title: How to determine whether a string ends with a specific character in Golang. In the Go language, sometimes we need to determine whether a string ends with a specific character. This is very common when processing strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference. First, let's take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be
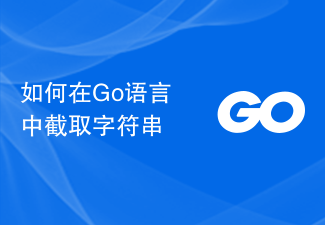
Go language is a powerful and flexible programming language that provides rich string processing functions, including string interception. In the Go language, we can use slices to intercept strings. Next, we will introduce in detail how to intercept strings in Go language, with specific code examples. 1. Use slicing to intercept a string. In the Go language, you can use slicing expressions to intercept a part of a string. The syntax of slice expression is as follows: slice:=str[start:end]where, s
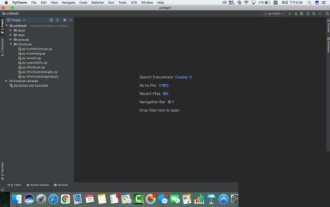
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
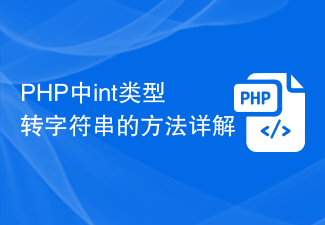
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
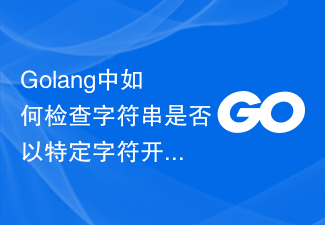
How to check if a string starts with a specific character in Golang? When programming in Golang, you often encounter situations where you need to check whether a string begins with a specific character. To meet this requirement, we can use the functions provided by the strings package in Golang to achieve this. Next, we will introduce in detail how to use Golang to check whether a string starts with a specific character, with specific code examples. In Golang, we can use HasPrefix from the strings package
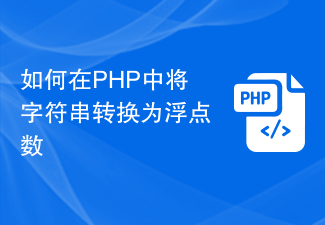
Converting a string to a floating point number is a common operation in PHP and can be accomplished through built-in methods. First make sure that the string is in a legal floating point format before it can be successfully converted to a floating point number. The following will detail how to convert a string to a floating point number in PHP and provide specific code examples. 1. Use (float) cast In PHP, the simplest way to convert a string into a floating point number is to use cast. The way to force conversion is to add (float) before the string, and PHP will automatically convert it
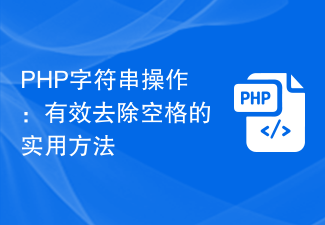
PHP String Operation: A Practical Method to Effectively Remove Spaces In PHP development, you often encounter situations where you need to remove spaces from a string. Removing spaces can make the string cleaner and facilitate subsequent data processing and display. This article will introduce several effective and practical methods for removing spaces, and attach specific code examples. Method 1: Use the PHP built-in function trim(). The PHP built-in function trim() can remove spaces at both ends of the string (including spaces, tabs, newlines, etc.). It is very convenient and easy to use.
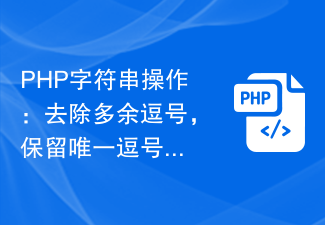
PHP String Operation: Remove Extra Commas and Keep Only Commas Implementation Tips In PHP development, string processing is a very common requirement. Sometimes we need to process the string to remove extra commas and retain the only commas. In this article, I'll introduce an implementation technique and provide concrete code examples. First, let's look at a common requirement: Suppose we have a string containing multiple commas, and we need to remove the extra commas and keep only the unique comma. For example, replace "apple,ba
