


How to write a function with output parameters (called by reference) in Python?
All parameters (arguments) in the Python language are passed by reference. This means that if you change the reference content of a parameter in a function, that change will also be reflected in the calling function.
Achieve this by -
Return result tuple
Example
In this example we will return a tuple of results -
# Function Definition def demo(val1, val2): val1 = 'new value' val2 = val2 + 1 return val1, val2 x, y = 'old value', 5 # Function call print(demo(x, y))
Output
('new value', 6)
Passing mutable objects
Example
In this example we will pass a mutable object -
# Function Definition def demo2(a): # 'a' references a mutable list a[0] = 'new-value' # This changes a shared object a[1] = a[1] + 1 args = ['old-value', 5] demo2(args) print(args)
Output
['new-value', 6]
Pass a mutated dictionary
Example
In this example we will pass a dictionary -
def demo3(args): # args is a mutable dictionary args['val1'] = 'new-value' args['val2'] = args['val2'] + 1 args = {'val1': 'old-value', 'val2': 5} # Function call demo3(args) print(args)
Output
{'val1': 'new-value', 'val2': 6}
Values in class instances
Example
In this example we will pack the value in the class instance -
class Namespace: def __init__(self, **args): for key, value in args.items(): setattr(self, key, value) def func4(args): # args is a mutable Namespace args.val1 = 'new-value' args.val2 = args.val2 + 1 args = Namespace(val1='old-value', val2=5) # Function Call func4(args) print(vars(args))
Output
{'val1': 'new-value', 'val2': 6}
The above is the detailed content of How to write a function with output parameters (called by reference) in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
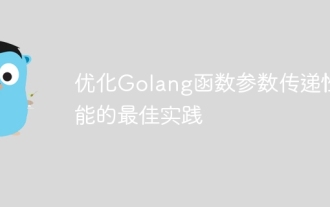
In order to optimize Go function parameter passing performance, best practices include: using value types to avoid copying small value types; using pointers to pass large value types (structures); using value types to pass slices; and using interfaces to pass polymorphic types. In practice, when passing large JSON strings, passing the data parameter pointer can significantly improve deserialization performance.
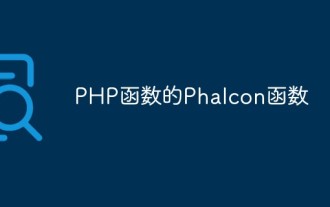
PHP is a powerful programming language that is widely used in web development. Phalcon is a high-performance full-stack framework based on PHP. Its unique feature is that many extension modules are written in C language, which greatly improves the performance of the framework. This article will focus on the function library in Phalcon and explore its application in PHP development. Phalcon function library is a large and comprehensive function library, including many commonly used PHP functions, such as string functions, array functions, date functions, etc. besides,
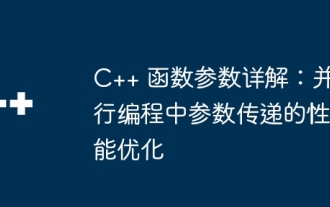
In a multi-threaded environment, function parameter passing methods are different, and the performance difference is significant: passing by value: copying parameter values, safe, but large objects are expensive. Pass by reference: Passing by reference is efficient, but function modifications will affect the caller. Pass by constant reference: Pass by constant reference, safe, but restricts the function's operation on parameters. Pass by pointer: Passing pointers is flexible, but pointer management is complex, and dangling pointers or memory leaks may occur. In parallel summation, passing by reference is more efficient than passing by value, and passing by pointer is the most flexible, but management is complicated.
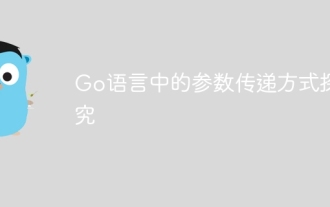
In the Go language, there are two main ways to pass function parameters: value passing: passing a copy of the variable will not affect the original variable in the calling code. Pointer passing: Passing the address of a variable allows the function to directly modify the original variable in the calling code.
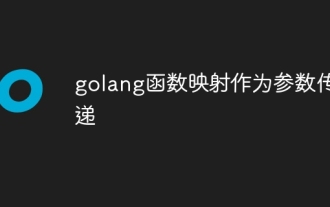
In Go, function maps can be passed as parameters of functions, providing code reuse and customization capabilities: Create a function map: use the map[string]interface{} type, with the function name as the key and the function itself as the value. Pass as parameter: Use the funcMap type in the function parameter list to accept a function map. Execute a function: Retrieve the function from the function map via the reflect package and call it with variable arguments. Practical case: Function mapping can be passed to the template engine to dynamically execute template functions at runtime.
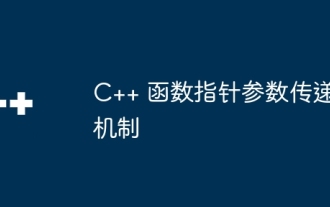
The function pointer is used as a parameter passing mechanism in C++: the function pointer is passed as a constant pointer, a copy is created during the passing process, the received function formal parameter points to the copy, and the dereferenced copy can call the underlying function.
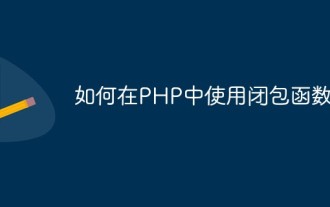
PHP closure function means that the variables used inside the function body defined when declaring the function and the variables in the external environment form a closed scope. This kind of function is also called an anonymous function. Closure functions are widely used in PHP and can be used to implement a series of functions such as event processing and callbacks. This article will introduce how to use closure functions in PHP, as well as some best practices for using closure functions. 1. How to define a closure function. Defining a closure function is very simple. You only need to use the function keyword followedby.
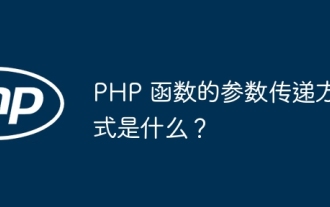
There are two ways to pass parameters in PHP: call by value (the parameter is passed as a copy of the value, modification within the function does not affect the original variable) and passing by reference (the address of the parameter is passed, modification within the function will affect the original variable), when the original variable needs to be modified Use reference passing when calculating the shopping cart total price, which requires reference passing to calculate correctly.
