


Minimize the number of 0s that need to be removed to maximize the length of the longest consecutive 1 substring
In this article, we will delve into an interesting problem involving C string operations. The problem we are going to study today is how to "minimize the number of 0s that need to be deleted to maximize the length of the longest continuous substring of 1s." This problem is a great way to hone your skills in string manipulation and dynamic programming.
Problem Statement
Given a binary string, the task is to minimize the number of 0s that need to be removed in order to maximize the length of the longest 1 substring.
C Solution
In order to solve this problem, we can use the sliding window method. We will maintain two pointers, left pointer and right pointer. Initially, both pointers point to the first element. Then we'll keep moving the right pointer to the right. If a '0' is encountered, we increment a counter. If the counter becomes larger than the allowed number of zero removals, we move the left pointer right until we encounter a '0' and decrement the counter.
We will also maintain a variable maxLen to store the maximum length of the 1 substring we have seen so far.
Example
This is the C code that solves the problem -
#include <iostream> #include <algorithm> #include <string> using namespace std; int maxSubstring(string str, int k) { int zeroCount = 0; int left = 0; int maxLen = 0; for (int right = 0; right < str.size(); right++) { if (str[right] == '0') { zeroCount++; } while (zeroCount > k) { if (str[left] == '0') { zeroCount--; } left++; } maxLen = max(maxLen, right - left + 1); } return maxLen; } int main() { string str = "110100110"; int k = 2; // number of zeros that can be removed int result = maxSubstring(str, k); cout << "The maximum length of the substring of 1s is: " << result << endl; return 0; }
Output
The maximum length of the substring of 1s is: 5
Test case description
Let's take the binary string "110100110", we can remove 2 zeros.
When we pass this string and the value of k to the maxSubstring function, it starts scanning from the left. Whenever it encounters a '0', it increments zeroCount. When zeroCount exceeds k, it starts moving the left pointer to the right until it encounters a '0' and decrements zeroCount.
In this process, it continuously updates maxLen, which is the maximum substring length of 1s. For the given string, the maximum substring length of 1s without removing at most 2 zeros is 5, i.e. the substring "11111" after removing the second and third '0'.
Therefore, the function will return 5.
in conclusion
This question demonstrates how to effectively use sliding window techniques to solve complex string manipulation problems in C. This is an excellent question for understanding and practicing dynamic programming and string processing techniques. Keep practicing such questions to improve your C coding skills.
The above is the detailed content of Minimize the number of 0s that need to be removed to maximize the length of the longest consecutive 1 substring. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
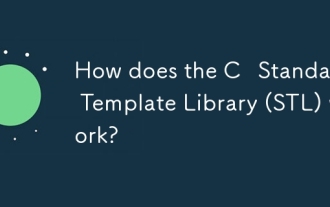
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
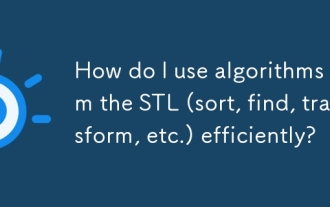
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
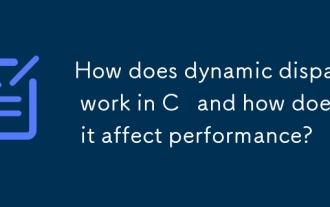
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
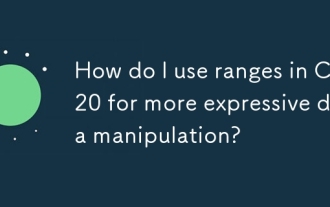
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
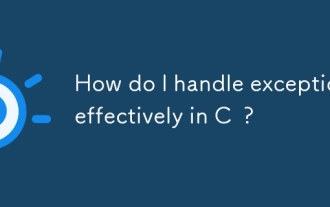
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
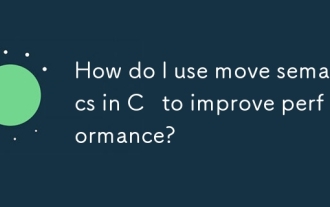
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
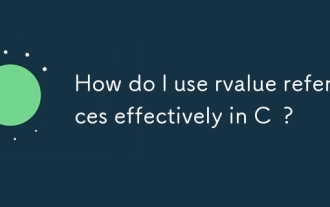
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
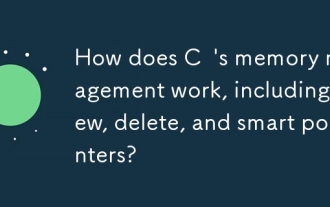
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
