What are the ways to generate random numbers in js
The methods for generating random numbers in js are: 1. Use the random function to generate random numbers between 0-1; 2. Use the random function and a specific range to generate random integers; 3. Use the random function and the round function Generate random integers between 0-99; 4. Use the random function and other functions to generate more complex random numbers; 5. Use the random function and other functions to generate random decimals within a range; 6. Use the random function and other functions to generate a range Random integers or decimals within.
#In JavaScript, there are many ways to generate random numbers. The following are some commonly used methods:
Use Math.random() to generate a random number between 0-1:
var random = Math.random(); console.log(random); // 输出一个0到1之间的随机数,包括0但不包括1
Use Math.random( ) and a specific range to generate a random integer:
var min = 1; var max = 100; var random = Math.floor(Math.random() * (max - min + 1)) + min; console.log(random); // 输出1到100之间的随机整数
Here, Math.random() generates a random number between 0 and 1, and then we multiply this random number by max - min 1, Get a random number within the desired range (including min and max). We then use Math.floor() to round this random number down to get an integer. Finally, we add min to this integer to get the final random integer.
Use Math.random() and Math.round() to generate a random integer between 0-99:
var random = Math.round(Math.random() * 99); console.log(random); // 输出0到99之间的随机整数
Use Math.random() and other functions to generate more complex random numbers:
function getRandomInt(min, max) { min = Math.ceil(min); max = Math.floor(max); return Math.floor(Math.random() * (max - min + 1)) + min; } console.log(getRandomInt(10, 50)); // 输出大于等于10且小于等于50的随机整数
This function first ensures that min and max are integers, then generates a random number within the range (including min and max), and finally returns this random number integer.
Use Math.random() and other functions to generate random decimals within a range:
function getRandomFloat(min, max) { return Math.random() * (max - min) + min; } console.log(getRandomFloat(10, 50)); // 输出在10到50之间的随机小数
Use Math.random() and other functions to generate a range Random integers or decimals:
function getRandomIntOrFloat(min, max) { if (min === Math.floor(min) && max === Math.floor(max)) { return Math.floor(Math.random() * (max - min + 1)) + min; } else { return Math.random() * (max - min) + min; } } console.log(getRandomIntOrFloat(10, 50)); // 可以输出大于等于10且小于等于50的随机整数或小数
This function first checks whether min and max are integers. If so, returns a random integer within the range (including min and max). If not, a random decimal number within the range (including min and max) is returned.
The above are some methods of generating random numbers in JavaScript. You can choose the appropriate method according to your needs.
The above is the detailed content of What are the ways to generate random numbers in js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
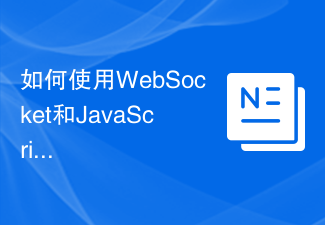
How to use WebSocket and JavaScript to implement an online speech recognition system Introduction: With the continuous development of technology, speech recognition technology has become an important part of the field of artificial intelligence. The online speech recognition system based on WebSocket and JavaScript has the characteristics of low latency, real-time and cross-platform, and has become a widely used solution. This article will introduce how to use WebSocket and JavaScript to implement an online speech recognition system.
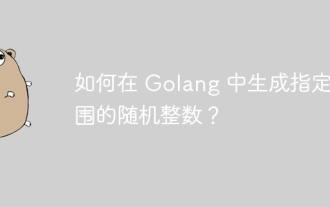
In Golang, use the Intn function in the rand package to generate a random integer within a specified range. The syntax is funcIntn(nint)int, where n is an exclusive random integer upper limit. By setting a random number seed and using Intn(100)+1, you can generate a random integer between 1 and 100 (inclusive). However, it should be noted that the random integers generated by Intn are pseudo-random and cannot generate random integers with a specific probability distribution.
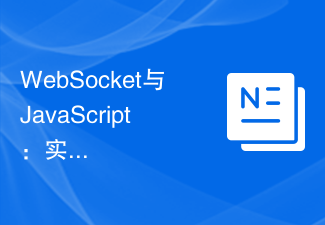
WebSocket and JavaScript: Key technologies for realizing real-time monitoring systems Introduction: With the rapid development of Internet technology, real-time monitoring systems have been widely used in various fields. One of the key technologies to achieve real-time monitoring is the combination of WebSocket and JavaScript. This article will introduce the application of WebSocket and JavaScript in real-time monitoring systems, give code examples, and explain their implementation principles in detail. 1. WebSocket technology
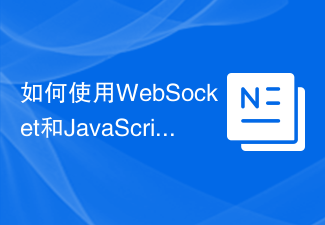
How to use WebSocket and JavaScript to implement an online reservation system. In today's digital era, more and more businesses and services need to provide online reservation functions. It is crucial to implement an efficient and real-time online reservation system. This article will introduce how to use WebSocket and JavaScript to implement an online reservation system, and provide specific code examples. 1. What is WebSocket? WebSocket is a full-duplex method on a single TCP connection.
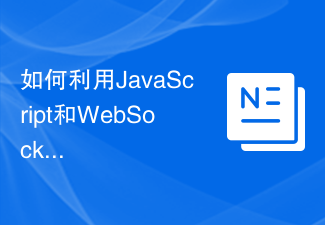
Introduction to how to use JavaScript and WebSocket to implement a real-time online ordering system: With the popularity of the Internet and the advancement of technology, more and more restaurants have begun to provide online ordering services. In order to implement a real-time online ordering system, we can use JavaScript and WebSocket technology. WebSocket is a full-duplex communication protocol based on the TCP protocol, which can realize real-time two-way communication between the client and the server. In the real-time online ordering system, when the user selects dishes and places an order
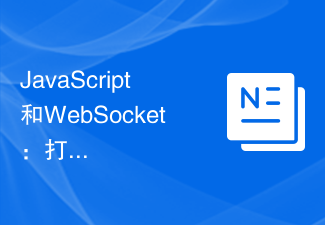
JavaScript and WebSocket: Building an efficient real-time weather forecast system Introduction: Today, the accuracy of weather forecasts is of great significance to daily life and decision-making. As technology develops, we can provide more accurate and reliable weather forecasts by obtaining weather data in real time. In this article, we will learn how to use JavaScript and WebSocket technology to build an efficient real-time weather forecast system. This article will demonstrate the implementation process through specific code examples. We
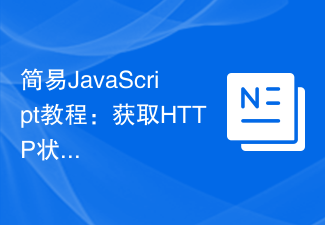
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
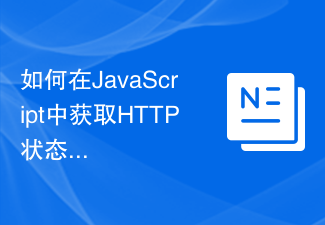
Introduction to the method of obtaining HTTP status code in JavaScript: In front-end development, we often need to deal with the interaction with the back-end interface, and HTTP status code is a very important part of it. Understanding and obtaining HTTP status codes helps us better handle the data returned by the interface. This article will introduce how to use JavaScript to obtain HTTP status codes and provide specific code examples. 1. What is HTTP status code? HTTP status code means that when the browser initiates a request to the server, the service
