Minimally modify a string so that all substrings are different
A string is a specific object that represents the sequence and flow of data characters. A string is a data container, always represented in text format. It is also used for conceptual, comparison, split, concatenate, replace, trim, length, internalize, equality, comparison and substring operations. substring() is a data refining process that extracts data between saved data from start to end. substring() does not change the original string. In a dataset, when we have different characters, they can be represented as different data elements. For example: 'a' and 'r' are different, while 'r' and 'r' are the same. So, a string of say, orange contains 6 different characters. Likewise, the string apple contains only 4 distinct characters.
Suppose, "s" is a string, and we need to find the minimum number of changes required for all substrings to make the string different.
The length of the string - 26
The given input − T is the test case in the first line, which is an integer. For each test case, there will only be one line containing 26 characters.
Output - We will get the minimum number of changes for each test case.
-
Constraints of logical method flow
1
1
In today's article, we will learn how to modify a string so that all substrings are different.
Algorithm for making substrings different
This is a possible algorithm for operating on a string such that all substrings are distinct while minimizing changes.
The first step - start.
Step 2 − Use two nested loops to generate substrings.
Step 3 - From i = 0 in the outer loop, the string length is reduced by 1.
Step 4 - Inner loop starts from j = 0, decrementing the string length by 1.
Step 5 − Construct a counting variable using zero value.
Step 6 − Inside the outer loop, create a distinct_character variable.
Step 7 - Create frequency array.
Step 8− Set all elements zero.
Step 9 - Check if the frequency of string[j]-'a' is zero.
Step 10− If it is zero, increase it by 1.
Step 11− Otherwise, break it into an inner loop.
Step 12 - If the count is greater than zero, return the count.
Step 13 - Otherwise, return -1.
Step 14 - Termination.
Syntax for creating all different substrings
string.substring(start, end)
In this syntax, we can see how to make minimal changes to a string such that all substrings are different.
parameter
Start - A starting position needs to be declared. The index of the first character here is 0.
End − It is an optional process located at the end (including but not limited to).
method
Method 1−Find the minimum number of changes that make all substrings of the string different.
Find the minimum number of changes such that all substrings of the string become different
In this method, we will learn how to make all substrings different. Here, every character has to be different. We just need to find the number of characters. If the length of the string is more than 26, then we just need to convert it to a string. Here we will write the same logic in different locales.
Example 1: Using C
#include <bits/stdc++.h> using namespace std; const int MAX_CHAR = 26; int minChanges(string &str) { int n = str.length(); if (n > MAX_CHAR) return -1; int dist_count = 0; int count[MAX_CHAR] = {0}; for (int i = 0; i < n; i++) { if (count[str[i] - 'a'] == 0) dist_count++; count[(str[i] - 'a')]++; } return (n - dist_count); } int main() { string str = "aebaecedabbeedee"; cout << minChanges(str); return 0; }
Output
11
Example 2: By using Java
import java.lang.*; import java.util.*; public class tutorialspoint { static final int MAX_CHAR = 26; public static int minChanges(String str) { int n = str.length(); if (n > MAX_CHAR) return -1; int dist_count = 0; int count[] = new int[MAX_CHAR]; for(int i = 0; i < MAX_CHAR; i++) count[i] = 0; for (int i = 0; i < n; i++) { if(count[str.charAt(i)-'a'] == 0) dist_count++; count[str.charAt(i)-'a']++; } return (n-dist_count); } public static void main (String[] args) { String str = "aebaecedabbeedee"; System.out.println(minChanges(str)); } }
Output
11
Example 1: Using Python
MAX_CHAR = [26] def minChanges(str): n = len(str ) if (n > MAX_CHAR[0]): return -1 dist_count = 0 count = [0] * MAX_CHAR[0] for i in range(n): if (count[ord(str[i]) - ord('a')] == 0) : dist_count += 1 count[(ord(str[i]) - ord('a'))] += 1 return (n - dist_count) if __name__ == '__main__': str = "aebaecedabbeedee" print(minChanges(str))
Output
11
in conclusion
Today, in this article, we learned how to make all substrings different with minimal changes. Here we have created some possible codes by following the described algorithm in C, Java and Python. Hopefully this helps you gain a more comprehensive understanding of the subject.
The above is the detailed content of Minimally modify a string so that all substrings are different. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


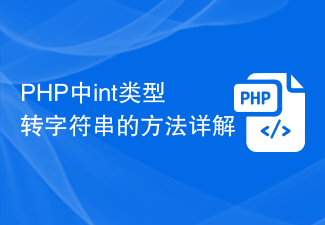
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
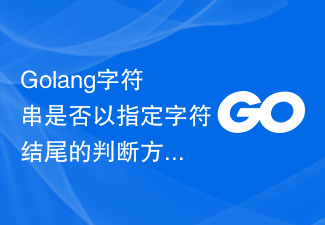
Title: How to determine whether a string ends with a specific character in Golang. In the Go language, sometimes we need to determine whether a string ends with a specific character. This is very common when processing strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference. First, let's take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be
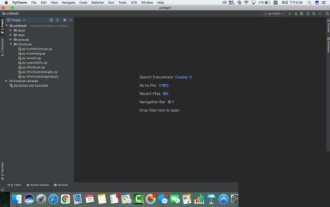
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
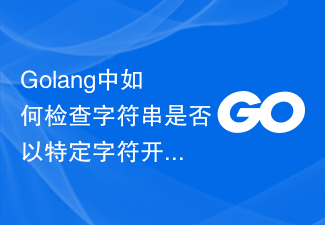
How to check if a string starts with a specific character in Golang? When programming in Golang, you often encounter situations where you need to check whether a string begins with a specific character. To meet this requirement, we can use the functions provided by the strings package in Golang to achieve this. Next, we will introduce in detail how to use Golang to check whether a string starts with a specific character, with specific code examples. In Golang, we can use HasPrefix from the strings package
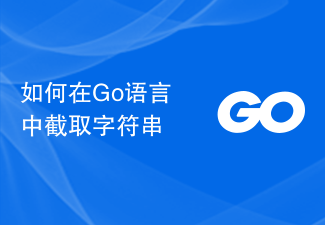
Go language is a powerful and flexible programming language that provides rich string processing functions, including string interception. In the Go language, we can use slices to intercept strings. Next, we will introduce in detail how to intercept strings in Go language, with specific code examples. 1. Use slicing to intercept a string. In the Go language, you can use slicing expressions to intercept a part of a string. The syntax of slice expression is as follows: slice:=str[start:end]where, s
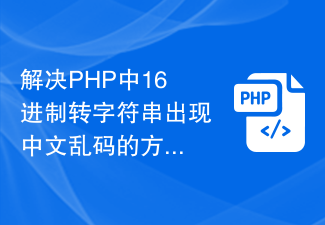
Methods to solve Chinese garbled characters when converting hexadecimal strings in PHP. In PHP programming, sometimes we encounter situations where we need to convert strings represented by hexadecimal into normal Chinese characters. However, in the process of this conversion, sometimes you will encounter the problem of Chinese garbled characters. This article will provide you with a method to solve the problem of Chinese garbled characters when converting hexadecimal to string in PHP, and give specific code examples. Use the hex2bin() function for hexadecimal conversion. PHP’s built-in hex2bin() function can convert 1
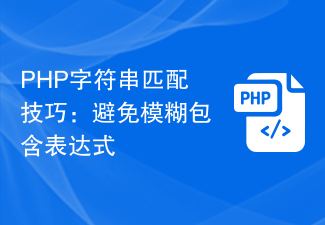
PHP String Matching Tips: Avoid Ambiguous Included Expressions In PHP development, string matching is a common task, usually used to find specific text content or to verify the format of input. However, sometimes we need to avoid using ambiguous inclusion expressions to ensure match accuracy. This article will introduce some techniques to avoid ambiguous inclusion expressions when doing string matching in PHP, and provide specific code examples. Use preg_match() function for exact matching In PHP, you can use preg_mat
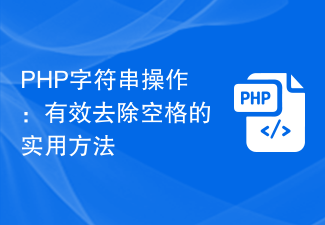
PHP String Operation: A Practical Method to Effectively Remove Spaces In PHP development, you often encounter situations where you need to remove spaces from a string. Removing spaces can make the string cleaner and facilitate subsequent data processing and display. This article will introduce several effective and practical methods for removing spaces, and attach specific code examples. Method 1: Use the PHP built-in function trim(). The PHP built-in function trim() can remove spaces at both ends of the string (including spaces, tabs, newlines, etc.). It is very convenient and easy to use.
