


How to use PHP to write a simple factory pattern to unify the object creation process
How to use PHP to write a simple factory pattern to unify the object creation process
Simple Factory pattern (Simple Factory) is a creational design pattern that can convert instances of objects into The process of object creation is centralized and the object creation process is unified. The simple factory pattern is very useful in actual projects. It can effectively reduce code redundancy and improve the maintainability and scalability of the code. In this article, we will learn how to use PHP to write a simple factory pattern to unify the object creation process. Let’s first understand the basic concepts of the simple factory pattern.
The basic structure of the simple factory pattern is as follows:
- Factory: Factory class, responsible for the unified object creation process. Based on the parameters passed, decide which specific object to create.
- Product: Abstract product class, the base class of all specific products. Defines the methods that need to be implemented for specific products.
- ConcreteProduct: Concrete product class, inherited from abstract product class. Implements the methods defined in the abstract product class.
Now, let’s use PHP to write an example to illustrate how to use the simple factory pattern to unify the object creation process.
First, we create an abstract product class Shape, which defines an abstract method calcArea() for calculating the area of the shape.
abstract class Shape { abstract function calcArea(); }
Then, we create concrete product classes Rectangle and Circle, which respectively inherit from the abstract product class Shape and implement the abstract method calcArea().
class Rectangle extends Shape { private $width; private $height; public function __construct($width, $height) { $this->width = $width; $this->height = $height; } public function calcArea() { return $this->width * $this->height; } } class Circle extends Shape { private $radius; public function __construct($radius) { $this->radius = $radius; } public function calcArea() { return 3.14 * $this->radius * $this->radius; } }
Next, we create a factory class ShapeFactory to dynamically create corresponding specific product objects based on the incoming parameters.
class ShapeFactory { public static function createShape($type, $params) { switch ($type) { case 'Rectangle': return new Rectangle($params['width'], $params['height']); case 'Circle': return new Circle($params['radius']); default: throw new Exception('Invalid shape type'); } } }
Finally, we can use the simple factory pattern to create specific product objects. The following is an example:
$rectangle = ShapeFactory::createShape('Rectangle', ['width' => 2, 'height' => 3]); $circle = ShapeFactory::createShape('Circle', ['radius' => 5]); echo 'Rectangle area: ' . $rectangle->calcArea() . PHP_EOL; echo 'Circle area: ' . $circle->calcArea() . PHP_EOL;
Run the above code, the following results will be output:
Rectangle area: 6 Circle area: 78.5
Through the above example, we can find that using the simple factory pattern can easily unify the object creation process , you only need to decide which specific product object to create based on the parameters passed in in the factory class, without having to repeat the object creation process multiple times in other places in the code.
To summarize, the simple factory pattern is an extremely simple and commonly used design pattern, suitable for scenarios where multiple objects with the same properties and behaviors need to be created. By using the simple factory pattern, we can centralize the instantiation process of objects and improve the maintainability and scalability of the code.
I hope this article will help you understand and apply the simple factory pattern. For more design patterns and PHP programming content, please stay tuned for other articles.
The above is the detailed content of How to use PHP to write a simple factory pattern to unify the object creation process. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


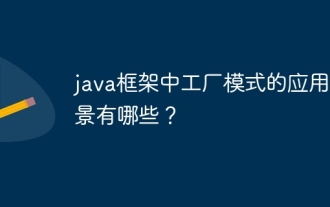
The factory pattern is used to decouple the creation process of objects and encapsulate them in factory classes to decouple them from concrete classes. In the Java framework, the factory pattern is used to: Create complex objects (such as beans in Spring) Provide object isolation, enhance testability and maintainability Support extensions, increase support for new object types by adding new factory classes
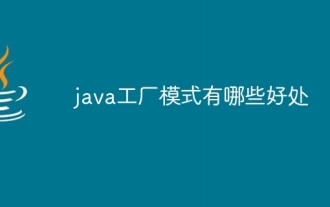
The benefits of the Java factory pattern: 1. Reduce system coupling; 2. Improve code reusability; 3. Hide the object creation process; 4. Simplify the object creation process; 5. Support dependency injection; 6. Provide better performance; 7. Enhance testability; 8. Support internationalization; 9. Promote the open and closed principle; 10. Provide better scalability. Detailed introduction: 1. Reduce the coupling of the system. The factory pattern reduces the coupling of the system by centralizing the object creation process into a factory class; 2. Improve the reusability of code, etc.
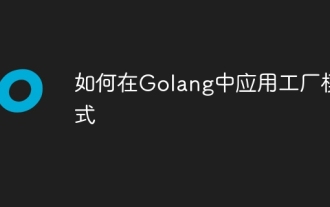
Factory Pattern In Go, the factory pattern allows the creation of objects without specifying a concrete class: define an interface (such as Shape) that represents the object. Create concrete types (such as Circle and Rectangle) that implement this interface. Create a factory class to create objects of a given type (such as ShapeFactory). Use factory classes to create objects in client code. This design pattern increases the flexibility of the code without directly coupling to concrete types.
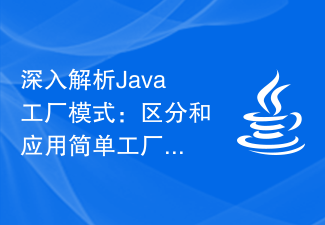
Detailed explanation of Java Factory Pattern: Understand the differences and application scenarios of simple factories, factory methods and abstract factories Introduction In the software development process, when faced with complex object creation and initialization processes, we often need to use the factory pattern to solve this problem. As a commonly used object-oriented programming language, Java provides a variety of factory pattern implementations. This article will introduce in detail the three common implementation methods of the Java factory pattern: simple factory, factory method and abstract factory, and conduct an in-depth analysis of their differences and application scenarios. one,
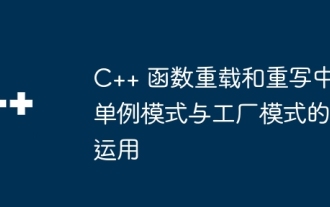
Singleton pattern: Provide singleton instances with different parameters through function overloading. Factory pattern: Create different types of objects through function rewriting to decouple the creation process from specific product classes.
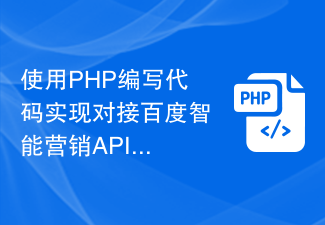
How to use PHP to write code to connect to Baidu Intelligent Marketing API. With the rapid development of the Internet and the further deepening of the advertising and marketing industry, more and more marketers and companies have begun to use various intelligent tools to improve advertising effects and delivery efficiency. . As an important tool, Baidu Intelligent Marketing API can help advertisers and developers realize automated advertising and optimization, thereby improving advertising effectiveness and ROI. This article will introduce how to use PHP to write code to connect to Baidu Intelligent Marketing API, and provide
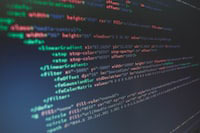
Introduction PHP design patterns are a set of proven solutions to common challenges in software development. By following these patterns, developers can create elegant, robust, and maintainable code. They help developers follow SOLID principles (single responsibility, open-closed, Liskov replacement, interface isolation and dependency inversion), thereby improving code readability, maintainability and scalability. Types of Design Patterns There are many different design patterns, each with its own unique purpose and advantages. Here are some of the most commonly used PHP design patterns: Singleton pattern: Ensures that a class has only one instance and provides a way to access this instance globally. Factory Pattern: Creates an object without specifying its exact class. It allows developers to conditionally
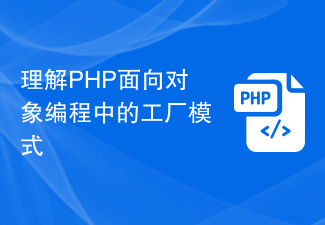
Understanding the Factory Pattern in PHP Object-Oriented Programming The factory pattern is a commonly used design pattern that is used to decouple the creation and use of objects during the process of creating objects. In PHP object-oriented programming, the factory pattern can help us better manage the creation and life cycle of objects. This article will introduce the factory pattern in PHP in detail through code examples. In PHP, we can implement the object creation and initialization process by using the factory pattern instead of directly using the new keyword. The advantage of this is that if changes need to be made in the future
