How to implement social login functionality using PHP
How to use PHP to implement social login function
With the popularity of social networks, more and more websites need to provide third-party social login functions so that users can conveniently Quickly use your existing social account to log in to the website. This article will introduce how to use PHP to implement the social login function and provide relevant code examples.
1. Preparation
Before you start, you need to have an application that can perform social login. For example, if you want to use the Facebook login function, you need to create an application on the Facebook Developer Platform and obtain the corresponding application ID and application key.
2. Configure the environment
First, make sure your PHP environment has the cURL extension installed. cURL is a powerful tool for communicating with servers, and we will use it to interact with social network APIs.
3. Use Facebook login function
Taking Facebook login as an example, the following is a simple PHP code example:
// 引入 Facebook 配置文件和 SDK require_once 'facebook-config.php'; require_once 'facebook-sdk/autoload.php'; // 初始化 Facebook SDK $fb = new FacebookFacebook([ 'app_id' => '{应用 ID}', 'app_secret' => '{应用密钥}', 'default_graph_version' => 'v3.2' ]); // 获取登录 URL $helper = $fb->getRedirectLoginHelper(); $loginUrl = $helper->getLoginUrl('{回调 URL}', ['email']); // 跳转到登录页面 header('Location: ' . $loginUrl); exit;
facebook-config.php in the above code
The file should contain your application ID and application key, and be set to the following form:
<?php return [ 'app_id' => '{应用 ID}', 'app_secret' => '{应用密钥}' ];
The callback URL is the page that the user will be redirected to after successful login, you can define it yourself. At the same time, the ['email']
parameter indicates that we need to obtain the user's email information.
After the user clicks the login link, it will jump to the Facebook login page and display an authorization dialog box, and the user needs to agree to the authorization.
Next, we need to write a callback page to handle the logic after successful login. The following is a simple example:
// 引入 Facebook 配置文件和 SDK require_once 'facebook-config.php'; require_once 'facebook-sdk/autoload.php'; // 初始化 Facebook SDK $fb = new FacebookFacebook([ 'app_id' => '{应用 ID}', 'app_secret' => '{应用密钥}', 'default_graph_version' => 'v3.2' ]); // 获取登录对象 $helper = $fb->getRedirectLoginHelper(); try { // 获取访问令牌 $accessToken = $helper->getAccessToken(); // 使用访问令牌获取用户信息 $response = $fb->get('/me?fields=id,name,email', $accessToken); $user = $response->getGraphUser(); // 输出用户信息 echo '用户 ID:' . $user->getId() . '<br>'; echo '用户名:' . $user->getName() . '<br>'; echo '用户邮箱:' . $user->getEmail() . '<br>'; } catch(FacebookExceptionsFacebookResponseException $e) { echo 'Graph 错误:' . $e->getMessage(); } catch(FacebookExceptionsFacebookSDKException $e) { echo 'SDK 错误:' . $e->getMessage(); }
The ['id', 'name', 'email']
parameters in the above code indicate that we need to obtain the user's ID, name and email information .
4. Use other social login functions
In addition to Facebook login, you can also use similar methods to implement other social login functions, such as using WeChat, Weibo, Google or GitHub to log in. Each social network has its own API, and you need to develop according to the specific API documentation.
Summary
This article introduces how to use PHP to implement the social login function, and gives a code example using Facebook login as an example. By reading this article, you should be able to easily implement social login functionality and implement it into your website or app.
Please note that for security reasons, in actual applications, you need to verify and filter user information to prevent potential security risks. In addition, you also need to handle the logic of the user's first login, such as registering a new account when the user logs in for the first time.
The above is the detailed content of How to implement social login functionality using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
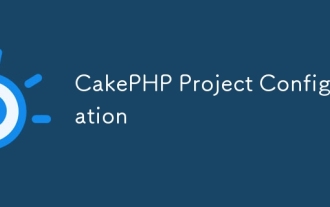
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
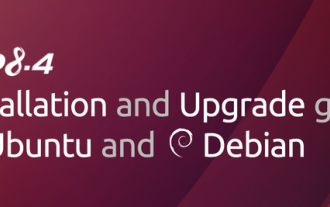
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
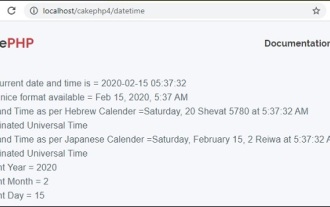
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
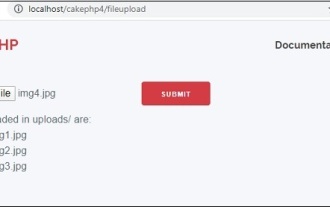
To work on file upload we are going to use the form helper. Here, is an example for file upload.
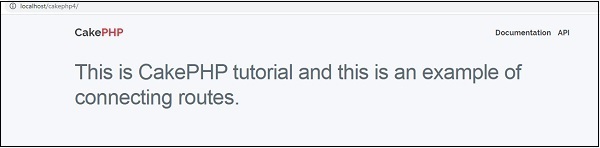
In this chapter, we are going to learn the following topics related to routing ?
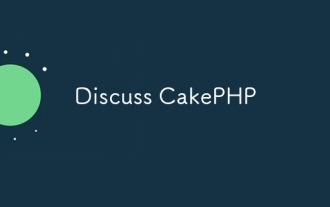
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
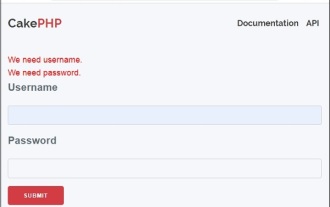
Validator can be created by adding the following two lines in the controller.
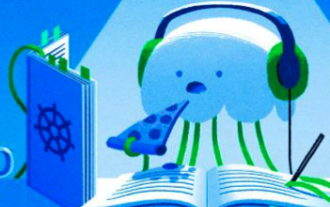
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
