C# program to check if HashTable collection is empty
The Hashtable collection in C# is a collection of key-value pairs organized according to the hash code of the key. The hash code is calculated using the hash code function.
Each element in a hash table is a key-value pair with a unique key. The key must also be non-null. Values can be empty and repeated.
In this article, we will discuss how to check if a hash table collection is empty.
How to check if the hash table collection is empty?
The class that implements hash table collection in C# is the Hashtable class. We can check if the hash table collection is empty by counting the number of elements present in the hash table.
To do this, we can use the "Count" property of the Hashtable class, which returns the number of elements in the hash table.
Therefore, if the Count property returns 0, it means that the hash table is empty, and if it returns a value greater than 0, it means that the hash table has elements.
Let’s first take a look at the prototype of the Count property of the Hashtable class.
grammar
public virtual int Count { get; }
Return value - Attribute value of type Int32
Description - Gets the number of key-value pairs contained in the hash table.
Namespaces
System.Collections
As can be seen from the above description of the Count attribute, we can use this attribute to obtain the number of key-value pairs in the hash table collection.
Now let's look at some programming examples that will help us understand this Count property.
Example
Let's see how the first program checks whether the hash table is empty. The procedure is as follows.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable myTable = new Hashtable(); //get the count of items in hashtable int mySize = myTable.Count; if(mySize == 0) Console.WriteLine("Hashtable is empty"); else Console.WriteLine("The hashtable is not empty. It has {0} item(s)", mySize); } }
In this program, we create a Hashtable object but do not add any elements to it. We then use the Count property to retrieve the count of elements present in the hash table. Finally, the value returned by the Count property is calculated and a message is displayed accordingly indicating whether the hash table is empty.
Output
The program generates the following output.
Hashtable is empty
Since there are no elements in the hash table, the message: Hash table is empty is displayed.
Now let us add some elements to the hash table in the above program. Now we add two elements to the hash table using the "Add()" method.
Example
The program is as follows.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable myTable = new Hashtable(); myTable.Add("1", "One"); myTable.Add("2", "Two"); //get the count of items in hashtable int mySize = myTable.Count; if(mySize == 0) Console.WriteLine("Hashtable is empty"); else Console.WriteLine("The hashtable is not empty. It has {0} item(s).", mySize); } }
Output
Here we add two elements to the hash table. The output now changes to look like below.
The hashtable is not empty. It has 2 item(s)
As we can see, the Count property returns the number of elements in the hash table.
Now let us see another example for better understanding.
Example
The procedure is as follows.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable langCode = new Hashtable(); langCode.Add("Perl", ""); //get the count of items in hashtable int hashtabSize = langCode.Count; if(hashtabSize == 0) Console.WriteLine("Hashtable is empty"); else Console.WriteLine("Hashtable is not empty. It has {0} item(s)", hashtabSize); } }
Output
Here we have a langCode hash table with one element in it. We again use the Count property to return the number of elements in the hash table. The output of this program is shown below.
Hashtable is not empty. It has 1 item(s)
Since there is an element in the hash table, the message is given appropriately. Now let us delete the elements present in the hash table. To do this, we simply comment out the line that adds elements to the hash table.
Example
The procedure is as follows.
using System; using System.Collections; class Program { public static void Main() { // Create a Hashtable Hashtable langCode = new Hashtable(); //langCode.Add("Perl", ""); //get the count of items in hashtable int hashtabSize = langCode.Count; if(hashtabSize == 0) Console.WriteLine("Hashtable is empty"); else Console.WriteLine("Hashtable is not empty. It has {0} item(s)", hashtabSize); } }
Output
There are no elements in the hash table now. So when we use the Count property on this hash table, it returns zero. So the output shows that the hash table is empty.
Hashtable is empty
So, since there is no direct method in the Hashtable class to check if the hash table is empty, we use the Count property of the Hashtable class to get the number of elements in the hash table. If Count returns 0, we conclude that the hash table is empty. If it returns a non-zero value, it means there are elements in the hash table.
The above is the detailed content of C# program to check if HashTable collection is empty. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


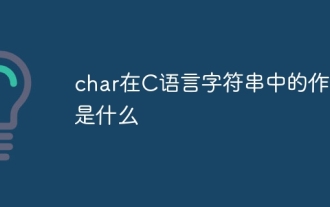
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
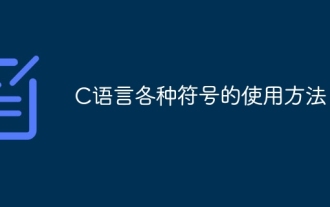
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
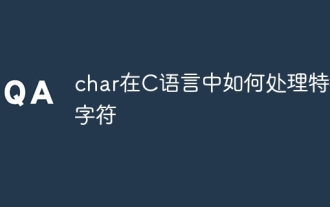
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
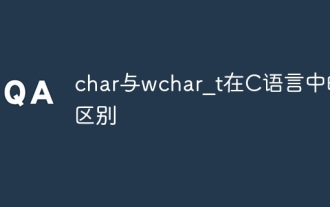
In C language, the main difference between char and wchar_t is character encoding: char uses ASCII or extends ASCII, wchar_t uses Unicode; char takes up 1-2 bytes, wchar_t takes up 2-4 bytes; char is suitable for English text, wchar_t is suitable for multilingual text; char is widely supported, wchar_t depends on whether the compiler and operating system support Unicode; char is limited in character range, wchar_t has a larger character range, and special functions are used for arithmetic operations.
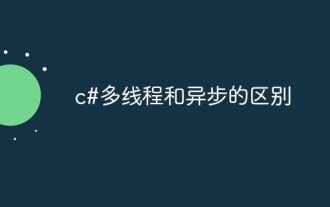
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
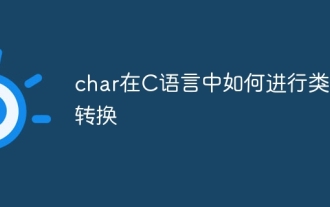
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.
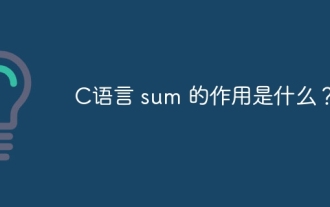
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
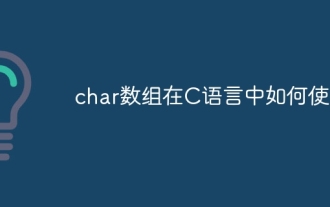
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
