How to verify CVV number using regular expression?
The three or four-digit number is called the Card Verification Value (CVV) and can be found on the back of most credit and debit cards and on the front of American Express cards . It is also known as CVV2 and CSC (Card Security Code).
The CVV code is a security mechanism that ensures the purchaser has a valid card. It was developed to help prevent unauthorized transactions. This information is often required when shopping online over the phone or when you don't have a card on hand.
method
The following method is used to verify the CVV number using regular expressions -
For 3-digit CVV code
For 4-digit CVV code
Method 1: For 3-digit CVV code
Most credit and debit cards have a security feature specifically printed on the back of them. This specific feature is a three-digit number named by a CVV or Card Verification Value Code, which comes in handy when shopping online or over the phone to verify the authenticity of a card without having the actual card in hand. As long as the given input conforms to the confirmed CVV code configuration format, it can be identified through regular expressions.
A string called a regular expression specifies a search pattern. In the case of CVV numbers, you can use regular expressions to verify that the input has three digits.
grammar
The syntax for validating three-digit CVV codes using regular expressions is as follows -
^\d {3}$
Where d represents any numeric character, 3 represents the exact number of times the previous character (digit) should appear, and $ represents the end of the string.
A string consisting of exactly three digits (in the format of a three-digit CVV code) matches this regular expression pattern. It can be used to check the accuracy of user input and ensure that CVV codes are entered in the correct format.
algorithm
Algorithm using regular expression to verify 3-digit CVV code−
Step 1 - Create a regular expression pattern that matches a 3-digit number. The correct pattern is "d3", where "d" stands for any number and "3" means there must be exactly three numbers.
Step 2 - Design a method to check the CVV code. After receiving a string as input, the function should return a boolean indicating whether the string matches the CVV pattern.
Step 3 - Use Python’s re module within the method to compile the regular expression pattern. An example is "cvv_pattern = re.compile(r'd3')".
Step 4 - Check whether the input string matches the CVV pattern using the match() method of the compiled pattern. As an illustration, use "match = cvv_pattern.match(input_str)".
Step 5 - Return True if the match is successful (i.e. the input string matches the CVV pattern). If not, returns False.
Example 1
Describes how to use regular expressions in C to automatically verify 3-digit CVV codes.
In this example, the sample CVV code is initially defined as a string variable. Then, using the syntax [0-9]3, we build a regular expression pattern that matches any three consecutive numbers.
Use the std::regex_match() function to compare regular expression patterns with CVV codes. If the CVV code matches the pattern, print "Valid CVV Code" on the screen, otherwise display "Invalid CVV Code".
#include <iostream> #include <regex> #include <string> int main() { std::string cvv = "123"; // Example CVV code std::regex cvv_regex("[0-9]{3}"); if (std::regex_match(cvv, cvv_regex)) { std::cout << "Valid CVV code\n"; } else { std::cout << "Invalid CVV code\n"; } return 0; }
Output
Valid CVV code
Method 2: For 4-digit CVV codes
In the world of credit and debit cards, the 4-digit CVV code is a variation of the Card Verification Value (CVV). While most card users only use the three-digit CVV code, American Express uses a four-digit code. By applying regular expressions, individuals can ensure that any correctly entered 4-digit CVV number is effectively verified.
grammar
Regular expression syntax that can be used to verify 4-digit CVV codes -
^\d {4}$
This regular expression is broken down as follows -
^ - string starts with
\d - any number (0-9)
{4} - Exactly four times
$ - end of string
This regular expression only recognizes strings with a total of four digits. If the user-supplied CVV code is not exactly four digits and contains any non-numeric characters, the CVV code will be output as invalid because the regular expression will not match.
algorithm
Step-by-step algorithm for validating 4-digit CVV codes using regular expressions -
Step 1 - Create a regular expression pattern that matches four digits. The formula for this pattern is d4, which corresponds to any run of four numbers.
Step 2 - Get the user’s CVV code.
Step 3 - To see if the CVV code matches the pattern, use a regular expression pattern. For example, you can use the re.match() function to compare CVV code with patterns in Python.
Step 4 - If the CVV code matches the pattern, then the CVV code is a legal 4-digit CVV code. You can trade now.
Step 5 - If your CVV code does not resemble the pattern, it is not a valid 4-digit CVV code. Error messages can be printed to the user and valid CVV codes entered can be printed on the screen.
示例 2
以下是一个使用正则表达式验证四位数字CVV码的C++示例,无需用户输入:
在这个例子中,我们使用std::regex类构造了一个匹配4位数字的正则表达式模式。cvv_regex变量保存了这个模式。
std regex_match 函数用于查找 cvv 字符串是否与 cvv_regex 模式匹配。 CVV码关注字符串是否符合模式;否则,确认无效。
#include <iostream> #include <regex> int main() { std::string cvv = "124"; // The CVV code to validate // Regular Expression to match 4-digit numbers std::regex cvv_regex("\b\d{4}\b"); if (std::regex_match(cvv, cvv_regex)) { std::cout << "Valid CVV code." << std::endl; } else { std::cout << "Invalid CVV code." << std::endl; } return 0; }
输出
Invalid CVV code.
结论
使用正则表达式验证CVV号码可以帮助确保输入的格式正确,并满足有效CVV的标准。CVV模式通常由正则表达式"bd3,4b"表示,该表达式匹配一个由三或四个数字组成的字符串,字符串前后有单词边界。开发人员可以通过将CVV输入与该正则表达式进行匹配来增强信用卡和借记卡交易的安全性。
The above is the detailed content of How to verify CVV number using regular expression?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
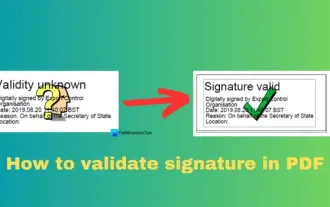
We usually receive PDF files from the government or other agencies, some with digital signatures. After verifying the signature, we see the SignatureValid message and a green check mark. If the signature is not verified, the validity is unknown. Verifying signatures is important, let’s see how to do it in PDF. How to Verify Signatures in PDF Verifying signatures in PDF format makes it more trustworthy and the document more likely to be accepted. You can verify signatures in PDF documents in the following ways. Open the PDF in Adobe Reader Right-click the signature and select Show Signature Properties Click the Show Signer Certificate button Add the signature to the Trusted Certificates list from the Trust tab Click Verify Signature to complete the verification Let
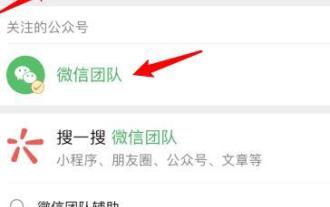
1. After opening WeChat, click the search icon, enter WeChat team, and click the service below to enter. 2. After entering, click the self-service tool option in the lower left corner. 3. After clicking, in the options above, click the option of unblocking/appealing for auxiliary verification.
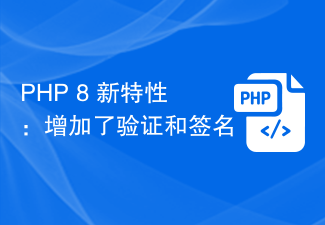
PHP8 is the latest version of PHP, bringing more convenience and functionality to programmers. This version has a special focus on security and performance, and one of the noteworthy new features is the addition of verification and signing capabilities. In this article, we'll take a closer look at these new features and their uses. Verification and signing are very important security concepts in computer science. They are often used to ensure that the data transmitted is complete and authentic. Verification and signatures become even more important when dealing with online transactions and sensitive information because if someone is able to tamper with the data, it could potentially
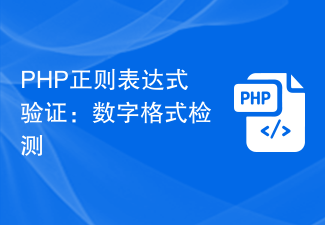
PHP regular expression verification: Number format detection When writing PHP programs, it is often necessary to verify the data entered by the user. One of the common verifications is to check whether the data conforms to the specified number format. In PHP, you can use regular expressions to achieve this kind of validation. This article will introduce how to use PHP regular expressions to verify number formats and provide specific code examples. First, let’s look at common number format validation requirements: Integers: only contain numbers 0-9, can start with a plus or minus sign, and do not contain decimal points. floating point
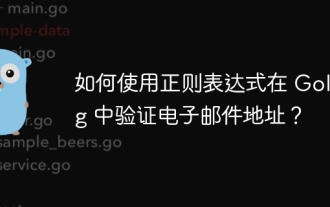
To validate email addresses in Golang using regular expressions, follow these steps: Use regexp.MustCompile to create a regular expression pattern that matches valid email address formats. Use the MatchString function to check whether a string matches a pattern. This pattern covers most valid email address formats, including: Local usernames can contain letters, numbers, and special characters: !.#$%&'*+/=?^_{|}~-`Domain names must contain at least One letter, followed by letters, numbers, or hyphens. The top-level domain (TLD) cannot be longer than 63 characters.
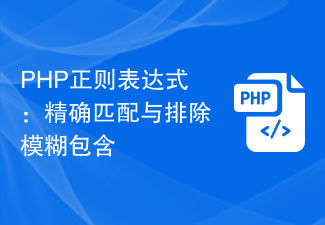
PHP Regular Expressions: Exact Matching and Exclusion Fuzzy inclusion regular expressions are a powerful text matching tool that can help programmers perform efficient search, replacement and filtering when processing text. In PHP, regular expressions are also widely used in string processing and data matching. This article will focus on how to perform exact matching and exclude fuzzy inclusion operations in PHP, and will illustrate it with specific code examples. Exact match Exact match means matching only strings that meet the exact condition, not any variations or extra words.
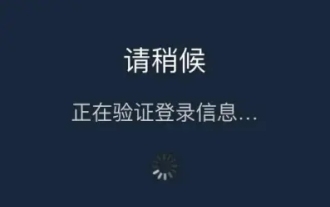
Steam is a platform used by game enthusiasts. You can buy and purchase many games here. However, recently many users have been stuck in the mobile token verification interface when logging into Steam and cannot log in successfully. Faced with this Most users don't know how to solve this situation. It doesn't matter. Today's software tutorial is here to answer the questions for users. Friends in need can check out the operation methods. Steam mobile token error? Solution 1: For software problems, first find the steam software settings on the mobile phone, request assistance page, and confirm that the network using the device is running normally, click OK again, click Send SMS, you can receive the verification code on the mobile phone page, and you are done. Verify, resolve when processing a request
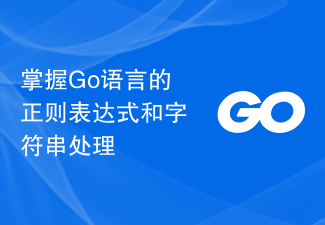
As a modern programming language, Go language provides powerful regular expressions and string processing functions, allowing developers to process string data more efficiently. It is very important for developers to master regular expressions and string processing in Go language. This article will introduce in detail the basic concepts and usage of regular expressions in Go language, and how to use Go language to process strings. 1. Regular expressions Regular expressions are a tool used to describe string patterns. They can easily implement operations such as string matching, search, and replacement.
