How to add and remove elements from PHP array
Sep 05, 2023 pm 02:12 PMHow to add and delete elements from PHP arrays
In PHP, arrays are a very common and important data structure. Arrays can hold multiple values and can dynamically add or subtract elements as needed. This article explains how to add and remove array elements in PHP and provides corresponding code examples.
1. Add elements
- Use square brackets [] syntax
The easiest way to add elements is to use square brackets [] syntax. An example is as follows:
1 2 3 |
|
This code will add a new element "grape"
to the original array $arr
. Use print_r()
function to print the array, the output is as follows:
1 2 3 4 5 6 7 |
|
- Use
array_push()
function
PHP also provides The array_push()
function is available, which can add one or more elements to the end of the array at a time. The example is as follows:
1 2 3 |
|
This code will add two new elements "grape"
and "watermelon"# to the original array
$arr ##. Use
print_r() function to print the array, the output is as follows:
1 2 3 4 5 6 7 8 |
|
- Use
- unset()
function
unset() function to delete one or more elements in the array. An example is as follows:
1 2 3 |
|
"banana" with index
1 in the array
$arr. Use the
print_r() function to print the array, the output is as follows:
1 2 3 4 5 |
|
- Use the
- array_splice()
function
The array_splice() function can implement more complex array element deletion operations, and can delete, replace or insert elements in the array. An example is as follows:
1 2 3 |
|
"banana" with index
1 in the array
$arr. Use the
print_r() function to print the array, the output is as follows:
1 2 3 4 5 |
|
- Use
- array_pop()
The function
array_pop() Function deletes the last element in the array. An example is as follows:
1 2 3 |
|
"orange" in the array
$arr. Use the
print_r() function to print the array, the output is as follows:
1 2 3 4 5 |
|
array_push() function,
unset() function,
array_splice() function or
array_pop() function can easily add and delete elements to an array, improving the efficiency and flexibility of PHP development.
The above is the detailed content of How to add and remove elements from PHP array. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
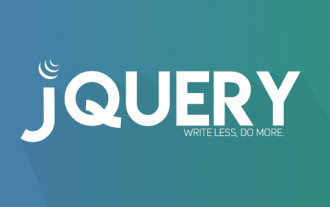
How to delete elements but keep child elements in jquery
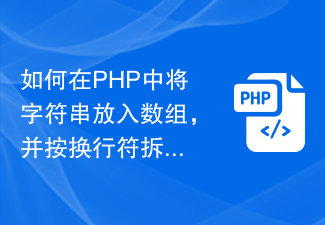
How to put string into array in PHP and split by newline
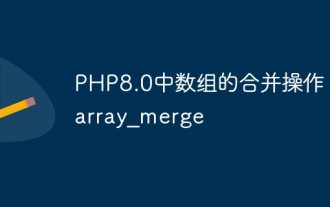
Merge operation of arrays in PHP8.0: array_merge
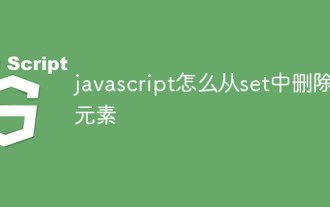
How to delete elements from set in javascript
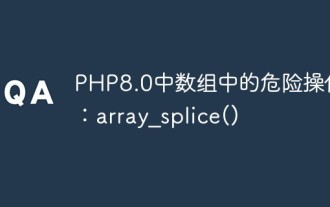
Dangerous operations on arrays in PHP8.0: array_splice()
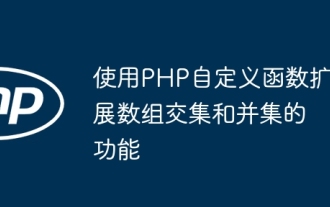
Use PHP custom functions to extend the functionality of array intersection and union

How to delete an element with a specified key in a PHP array

Best Practices for Array Operations with PHP
