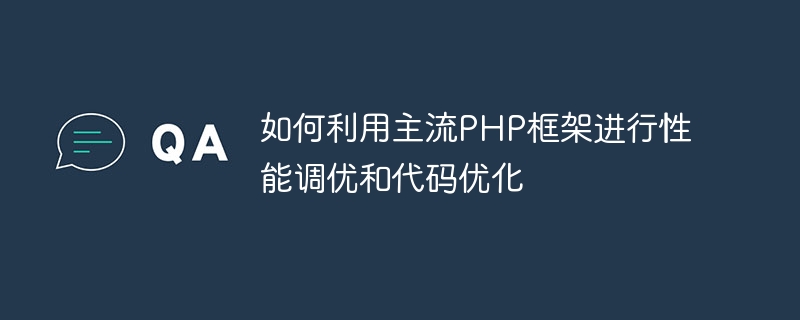
How to use mainstream PHP frameworks for performance tuning and code optimization
Abstract: PHP is a widely used programming language, and mainstream PHP frameworks can help We develop applications more efficiently. However, while writing code, we should also pay attention to performance tuning and code optimization to improve application performance and user experience. This article will focus on how to use mainstream PHP frameworks for performance tuning and code optimization, and provide code examples.
Introduction:
In the development process of network applications, performance tuning and code optimization are crucial links. By optimizing our code, we can improve our application's performance, loading speed, and user experience. The mainstream PHP frameworks, such as Laravel, Symfony and Yii, etc., provide various functions and tools to help us perform performance tuning and code optimization. Next, we will detail some common techniques and methods of these frameworks.
1. Performance tuning and code optimization in the Laravel framework:
- Use caching: Laravel provides a complete caching system that can cache frequently accessed data in memory , thereby reducing the number of database queries. For example, you can use Laravel's cache facade to cache query results or views to improve application response speed.
// 缓存查询结果
$users = Cache::remember('users', $minutes, function () {
return DB::table('users')->get();
});
// 缓存视图
if (Cache::has('view.homepage')) {
return Cache::get('view.homepage');
} else {
$content = view('homepage')->render();
Cache::put('view.homepage', $content, $minutes);
return $content;
}
Copy after login
- Use queues to process tasks: For some time-consuming tasks, you can use Laravel's queue system to process them asynchronously, thereby improving the response speed of the application. For example, you can use Laravel's task scheduler to put tasks into a queue and utilize queue listeners to handle tasks.
// 创建任务
class ProcessPodcast implements ShouldQueue
{
public function handle()
{
// 处理任务
}
}
// 将任务放入队列
ProcessPodcast::dispatch();
Copy after login
- Use lazy loading: Laravel supports lazy loading, which means loading related models or associated data when needed. This can reduce unnecessary database queries and improve performance. For example, you can use Laravel's
with
method to lazily load associated data.
$posts = AppPost::with('comments')->get();
Copy after login
2. Performance tuning and code optimization in the Symfony framework:
- Use Doctrine query cache: The Symfony framework integrates Doctrine ORM by default, and you can enable query cache by To reduce the number of database queries. You can use the cache function with a simple configuration.
- Use HTTP caching: The Symfony framework has built-in support for HTTP caching, which can cache pages on the client or server side, thereby reducing unnecessary page requests. HTTP caching can be implemented by configuring related HTTP header information such as
Cache-Control
and Expires
.
- Use event listeners: The Symfony framework provides an event system that can handle some time-consuming operations by defining event listeners. For example, before adding order information to the database, you can use event listeners to handle the rest of the logic in a database transaction.
3. Performance tuning and code optimization in the Yii framework:
- Use data caching: The Yii framework has a built-in complete data caching system that supports a variety of Cache drivers, such as file cache, memory cache and Redis cache, etc. You can use Yii's cache component to cache data and speed up database access.
- Use relational data preloading: The Yii framework provides a mechanism for relational data preloading, which can load data from multiple related models in one query, thereby reducing the number of database queries. Preload related associated model data by using Yii's
with
method.
- Use Gii to generate efficient code: Gii is the code generator that comes with the Yii framework. It can generate basic CURD operation code based on the data table and automatically optimize the generated code. You can use Gii to generate efficient code and reduce the workload of manually writing repetitive CURD code.
Conclusion:
Performance tuning and code optimization are important aspects of developing high-performance applications. Mainstream PHP frameworks, such as Laravel, Symfony and Yii, provide a wealth of functions and tools to help us perform performance tuning and code optimization. By using technologies such as caching, queuing, and lazy loading, application performance and user experience can be improved. I hope that some common tips and methods provided in this article can help you make better use of mainstream PHP frameworks for performance tuning and code optimization.
References:
- Laravel Documentation: https://laravel.com/docs
- Symfony Documentation: https://symfony.com/doc
- Yii Documentation: https://www.yiiframework.com/doc
The above is the detailed content of How to use mainstream PHP frameworks for performance tuning and code optimization. For more information, please follow other related articles on the PHP Chinese website!