


How to use PHP to implement form validation and submission functions
How to use PHP to implement form validation and submission functions
Overview:
In website development, forms are one of the important ways to interact with users. Forms are often used to collect information from users or receive feedback from users. In order to ensure the legality and integrity of the data submitted in the form, we need to verify the form. This article will introduce how to use PHP to implement form validation and submission functions, and provide relevant code examples.
Step 1: Create HTML form
First, we need to create an HTML form to collect user input data. The sample code is as follows:
<!DOCTYPE html> <html> <head> <title>表单验证和提交示例</title> </head> <body> <h2>用户信息</h2> <form action="submit.php" method="post"> <label for="name">姓名:</label> <input type="text" id="name" name="name" required><br><br> <label for="email">邮箱:</label> <input type="email" id="email" name="email" required><br><br> <label for="phone">电话:</label> <input type="tel" id="phone" name="phone" required><br><br> <input type="submit" value="提交"> </form> </body> </html>
Step 2: Create a PHP script file
Next, we need to create a PHP script file to validate the form data and submit it to the server. The sample code is as follows (the file name is submit.php):
<!DOCTYPE html> <html> <head> <title>表单验证和提交示例</title> </head> <body> <h2>提交结果</h2> <?php // 验证表单数据 $name = $_POST['name']; $email = $_POST['email']; $phone = $_POST['phone']; $errors = []; // 验证姓名 if (empty($name)) { $errors[] = "姓名不能为空"; } // 验证邮箱 if (empty($email)) { $errors[] = "邮箱不能为空"; } else if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $errors[] = "邮箱格式不正确"; } // 验证电话 if (empty($phone)) { $errors[] = "电话不能为空"; } else if (!preg_match('/^d{11}$/', $phone)) { $errors[] = "电话格式不正确"; } // 如果有错误,则显示错误信息 if (!empty($errors)) { foreach ($errors as $error) { echo "<p>{$error}</p>"; } } else { // 执行提交操作 // ... echo "<p>提交成功</p>"; } ?> </body> </html>
Step 3: Form verification
In the above code, we obtain the form submission through PHP's $_POST
super global variable data and verify it. If the data does not meet the requirements, error information is added to the $errors
array. The specific verification rules are as follows:
- The name cannot be empty
- The email address cannot be empty and must be a legal email format
- The phone number cannot be empty and must be It is an 11-digit number
Step 4: Submit operation
If all the data in the form is verified, you can perform the relevant submission operation. Here is only a simple success tip. In actual operation, you may need to store data in the database or send emails.
Summary:
Through the above steps, we can use PHP to implement form verification and submission functions. In actual development, we can conduct more detailed verification of form fields and add more submission operations according to specific needs. This ensures that we receive valid and complete data from our users.
Note: Due to security risks in modern website development, we strongly recommend dual verification on both front-end and back-end to enhance the security of the website.
The above is the detailed content of How to use PHP to implement form validation and submission functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
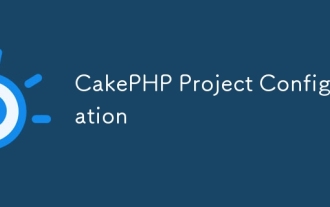
In this chapter, we will understand the Environment Variables, General Configuration, Database Configuration and Email Configuration in CakePHP.
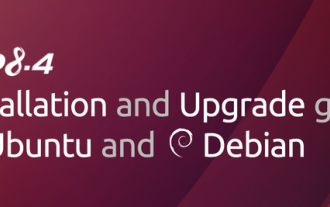
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
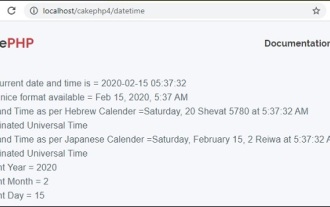
To work with date and time in cakephp4, we are going to make use of the available FrozenTime class.
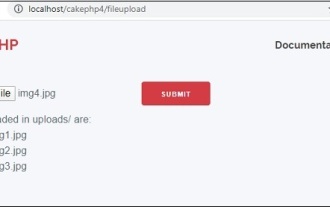
To work on file upload we are going to use the form helper. Here, is an example for file upload.
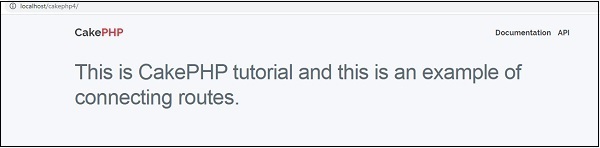
In this chapter, we are going to learn the following topics related to routing ?
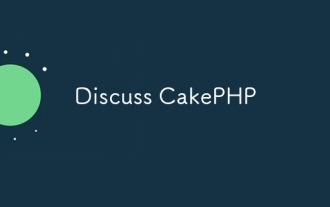
CakePHP is an open-source framework for PHP. It is intended to make developing, deploying and maintaining applications much easier. CakePHP is based on a MVC-like architecture that is both powerful and easy to grasp. Models, Views, and Controllers gu
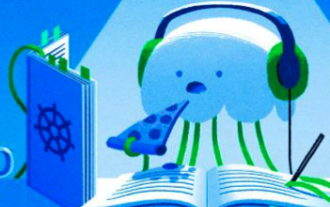
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
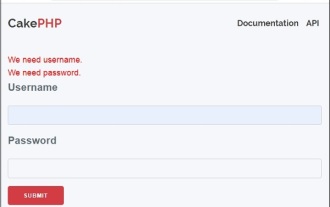
Validator can be created by adding the following two lines in the controller.
