


How to implement object encapsulation and hiding through PHP object-oriented simple factory pattern
How to realize object encapsulation and hiding through PHP object-oriented simple factory pattern
Introduction:
In PHP object-oriented programming, encapsulation is a kind of implementation data Hidden important concepts. Encapsulation can encapsulate data and related operations in a class, and operate the data through a public interface exposed to the outside world. The simple factory pattern is a commonly used design pattern for creating objects. It separates the creation and use of objects, making the system more flexible and easy to expand. This article will combine sample code to introduce how to implement object encapsulation and hiding through the PHP object-oriented simple factory pattern.
Implementation steps:
- Create an abstract class or interface to define the public interface of the object.
- Create concrete classes, implement abstract classes or interfaces, and encapsulate and hide data.
- Create a simple factory class in which instances of specific classes are created based on conditions.
Code Example:
- Create an abstract class or interface.
<?php abstract class Shape { protected $color; abstract public function draw(); } ?>
- Create concrete classes and encapsulate and hide data.
<?php class Circle extends Shape { private $radius; public function __construct($radius, $color) { $this->radius = $radius; $this->color = $color; } public function draw() { echo "Drawing a ".$this->color." circle with radius ".$this->radius.".<br>"; } } class Square extends Shape { private $length; public function __construct($length, $color) { $this->length = $length; $this->color = $color; } public function draw() { echo "Drawing a ".$this->color." square with length ".$this->length.".<br>"; } } ?>
- Create a simple factory class.
<?php class ShapeFactory { public static function create($type, $params) { switch ($type) { case 'circle': return new Circle($params['radius'], $params['color']); case 'square': return new Square($params['length'], $params['color']); default: throw new Exception('Invalid shape type.'); } } } ?>
Usage example:
<?php $params = [ 'radius' => 5, 'color' => 'red' ]; $circle = ShapeFactory::create('circle', $params); $circle->draw(); $params = [ 'length' => 10, 'color' => 'blue' ]; $square = ShapeFactory::create('square', $params); $square->draw(); ?>
Through the above code examples, we can see that the encapsulation and hiding of objects can be achieved through the PHP object-oriented simple factory pattern. Properties in a concrete class are encapsulated as private properties, which can only be specified through the constructor method and accessed through the public methods of the abstract class or interface. The simple factory class is responsible for creating instances of specific classes based on conditions, separating the creation and use of objects, and realizing the hiding of objects.
Conclusion:
Through the PHP object-oriented simple factory pattern, we can easily realize the encapsulation and hiding of objects. This approach can improve the maintainability and scalability of the code and make the system more flexible. In actual development, appropriate design patterns can be selected according to specific needs to improve code quality and efficiency.
The above is the detailed content of How to implement object encapsulation and hiding through PHP object-oriented simple factory pattern. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


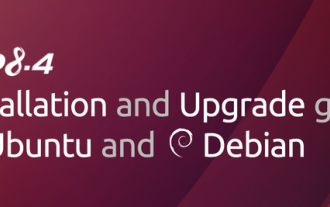
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
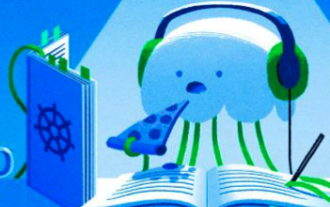
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
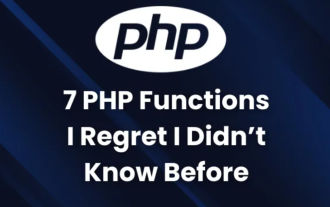
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
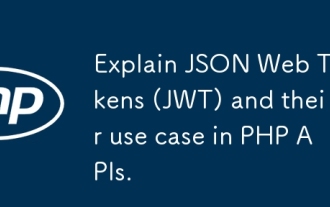
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
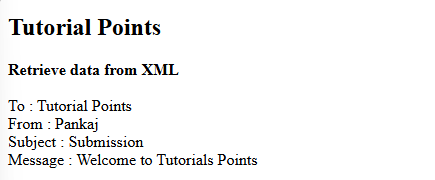
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
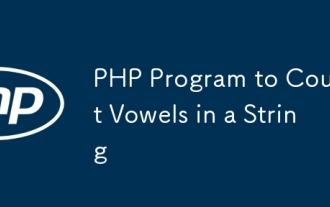
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
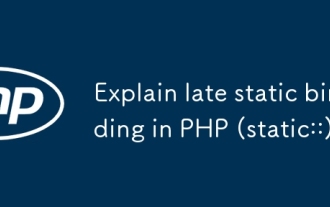
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
