Master the key skills and best practices of the Java technology stack
Key skills and best practices for mastering the Java technology stack
In the field of modern software development, Java has become a widely used programming language. Mastering the key skills and best practices of the Java technology stack is crucial to improving development efficiency and code quality. This article will introduce some key tips and best practices in Java development, with code examples.
1. Reasonable use of object-oriented programming
Java is an object-oriented programming language, and object-oriented programming is one of the core concepts in Java development. Reasonable use of object-oriented programming can improve the maintainability and reusability of code.
When designing a class, you must follow the Single Responsibility Principle (SRP), that is, a class should have only one responsibility. This can maintain the cohesion of the class and facilitate subsequent maintenance and expansion. The following is an example:
public class UserManager { public void addUser(User user) { // 添加用户的逻辑 } public void removeUser(User user) { // 移除用户的逻辑 } }
2. Reasonable use of exception handling mechanism
Exception handling is a very important part of Java programs. Proper handling of exceptions can improve the robustness and readability of the program.
In Java, use try-catch statement blocks to handle exceptions. When catching exceptions, you can choose to catch a specific exception type, or you can choose to catch a more general Exception type. The following is an example:
try { // 可能会导致异常的代码 } catch (FileNotFoundException e) { // 处理FileNotFoundException的逻辑 } catch (IOException e) { // 处理IOException的逻辑 } catch (Exception e) { // 处理其他异常的逻辑 }
3. Use the appropriate collection class
Java provides a variety of collection classes, such as ArrayList, LinkedList, HashMap, etc. Using the appropriate collection class can improve the performance and readability of your program.
ArrayList is suitable for scenarios that require efficient access to data, and LinkedList is suitable for scenarios that require frequent insertion and deletion of elements. HashMap is suitable for scenarios where elements need to be found quickly. The following is an example:
// 使用ArrayList List<String> list = new ArrayList<>(); list.add("item1"); list.add("item2"); list.remove("item1"); // 使用HashMap Map<String, String> map = new HashMap<>(); map.put("key1", "value1"); map.put("key2", "value2"); String value = map.get("key1");
4. Using annotations
Annotations are an important feature in Java, which can be used to add metadata to the code to achieve more advanced functions, such as automated generation. Code, configuration, etc. Reasonable use of annotations can improve the readability and maintainability of code.
When customizing annotations, follow the naming convention and add appropriate elements to the annotations. The following is an example:
@Retention(RetentionPolicy.RUNTIME) @Target(ElementType.TYPE) public @interface MyAnnotation { String value(); String[] tags() default {}; } // 使用自定义注解 @MyAnnotation(value = "MyClass", tags = {"tag1", "tag2"}) public class MyClass { // 类的定义 }
5. Proper handling of resources and memory
Resource and memory management in Java is an important topic. Proper handling of resources and memory can improve the performance and robustness of your program.
When processing files, network connections, and other resources that need to be closed manually, use the try-with-resources statement block to automatically close the resources. Here is an example:
try (InputStream is = new FileInputStream("file.txt"); OutputStream os = new FileOutputStream("output.txt")) { // 处理文件的逻辑 } catch (IOException e) { // 处理IO异常的逻辑 }
In addition to resource management, memory management in Java is also very important. Proper use of memory can improve program performance and stability. The following is an example:
// 设置合适的堆内存大小 java -Xms512m -Xmx1024m MyProgram
Summary
The Java technology stack is a large and complex system, and mastering the key skills and best practices is crucial to improving development efficiency and code quality. This article introduces some key tips and best practices in Java development and provides code examples. I hope this article will be helpful to readers in Java development.
The above is the detailed content of Master the key skills and best practices of the Java technology stack. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


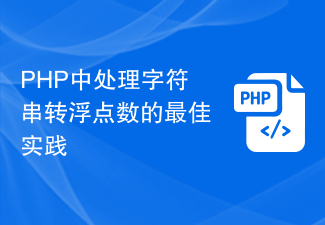
Converting strings to floating point numbers in PHP is a common requirement during the development process. For example, the amount field read from the database is of string type and needs to be converted into floating point numbers for numerical calculations. In this article, we will introduce the best practices for converting strings to floating point numbers in PHP and give specific code examples. First of all, we need to make it clear that there are two main ways to convert strings to floating point numbers in PHP: using (float) type conversion or using (floatval) function. Below we will introduce these two
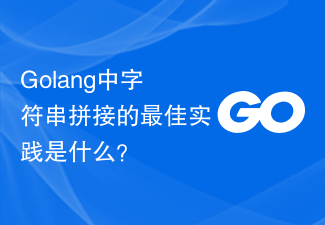
What are the best practices for string concatenation in Golang? In Golang, string concatenation is a common operation, but efficiency and performance must be taken into consideration. When dealing with a large number of string concatenations, choosing the appropriate method can significantly improve the performance of the program. The following will introduce several best practices for string concatenation in Golang, with specific code examples. Using the Join function of the strings package In Golang, using the Join function of the strings package is an efficient string splicing method.
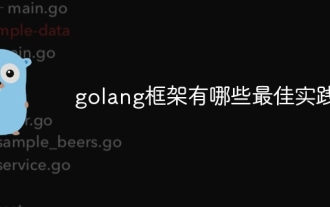
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.

In Go language, good indentation is the key to code readability. When writing code, a unified indentation style can make the code clearer and easier to understand. This article will explore the best practices for indentation in the Go language and provide specific code examples. Use spaces instead of tabs In Go, it is recommended to use spaces instead of tabs for indentation. This can avoid typesetting problems caused by inconsistent tab widths in different editors. The number of spaces for indentation. Go language officially recommends using 4 spaces as the number of spaces for indentation. This allows the code to be
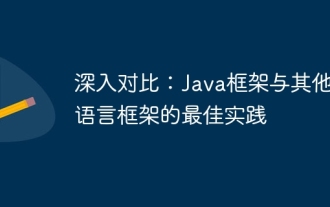
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
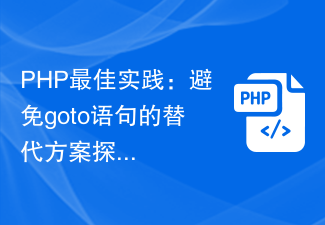
PHP Best Practices: Alternatives to Avoiding Goto Statements Explored In PHP programming, a goto statement is a control structure that allows a direct jump to another location in a program. Although the goto statement can simplify code structure and flow control, its use is widely considered to be a bad practice because it can easily lead to code confusion, reduced readability, and debugging difficulties. In actual development, in order to avoid using goto statements, we need to find alternative methods to achieve the same function. This article will explore some alternatives,
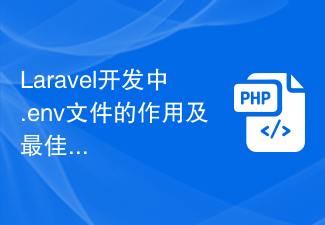
The role and best practices of .env files in Laravel development In Laravel application development, .env files are considered to be one of the most important files. It carries some key configuration information, such as database connection information, application environment, application keys, etc. In this article, we’ll take a deep dive into the role of .env files and best practices, along with concrete code examples. 1. The role of the .env file First, we need to understand the role of the .env file. In a Laravel should
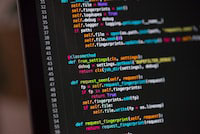
Version Control: Basic version control is a software development practice that allows teams to track changes in the code base. It provides a central repository containing all historical versions of project files. This enables developers to easily rollback bugs, view differences between versions, and coordinate concurrent changes to the code base. Git: Distributed Version Control System Git is a distributed version control system (DVCS), which means that each developer's computer has a complete copy of the entire code base. This eliminates dependence on a central server and increases team flexibility and collaboration. Git allows developers to create and manage branches, track the history of a code base, and share changes with other developers. Git vs Version Control: Key Differences Distributed vs Set
