


Getting Started with PHP File Processing: Detailed Basic Steps for Reading and Writing
Introduction to PHP file processing: Detailed basic steps for reading and writing
Overview:
In web development, processing files is a very common task Task. As a powerful server-side scripting language, PHP provides a wealth of file processing functions and methods, which can easily read and write file contents. This article will introduce the basic steps of reading and writing files using PHP and provide corresponding code examples.
- Read file content:
Reading file content is one of the common file processing tasks. PHP provides multiple functions and methods to achieve this goal. The following are the general steps for reading the contents of a file:
(1) Open the file:
To open a file in PHP, you need to use the fopen() function. This function requires two parameters, the first parameter is the file path, and the second parameter is the file opening mode. Common file opening modes are:
- "r": read-only, the file pointer points to the beginning of the file.
- "w": Write only, clear the file content, and create a new file if the file does not exist.
- "a": Write only, append content to the end of the file, create a new file if the file does not exist.
- "x": Write only, create a new file, and fail to open if the file already exists.
- "b": Binary mode, used to process binary files.
Code example 1: Read file content
$filename = "test.txt"; $file = fopen($filename, "r"); if ($file) { while (($line = fgets($file)) !== false) { echo $line; } fclose($file); } else { echo "文件打开失败!"; }
(2) Read file content:
You can use the fgets() function to read file content. This function reads the contents of the file one line at a time and moves the file pointer to the next line. If the end of the file is read, false will be returned.
(3) Close the file:
After reading the file content, you should use the fclose() function to close the file and release system resources.
- Write file content:
Writing file content is also one of the frequently used functions. PHP also provides multiple functions and methods to achieve this goal. The following are the general steps for writing file content:
(1) Open the file:
Writing file content also requires the use of the fopen() function. The common file opening mode used is "w" and "a".
Code example 2: Write file content
$filename = "test.txt"; $file = fopen($filename, "w"); if ($file) { $content = "Hello, World!"; fwrite($file, $content); fclose($file); echo "文件写入成功!"; } else { echo "文件打开失败!"; }
(2) Write file content:
You can use the fwrite() function to write file content. This function requires two parameters, the first parameter is the open file pointer, and the second parameter is the content to be written.
- Error handling:
During the file processing process, various error conditions may occur, such as failure to open the file, failure to read, failure to write, etc. To handle these errors, conditional statements and exception handling mechanisms can be used. For example, when opening a file, you can use conditional statements to determine whether the file is successfully opened; when reading or writing a file, you can use conditional statements to determine whether the operation is successful; if an error occurs, you can handle it accordingly through the exception handling mechanism.
Code Example 3: File Processing Error Handling
$filename = "test.txt"; try { $file = fopen($filename, "r"); if (!$file) { throw new Exception("文件打开失败!"); } while (($line = fgets($file)) !== false) { echo $line; } fclose($file); } catch (Exception $e) { echo $e->getMessage(); }
Conclusion:
Through the introduction of this article, we have learned the basic steps of using PHP to read and write files. For the file processing needs in developing web applications, mastering these basic knowledge will greatly improve development efficiency and code quality. In actual development, you also need to pay attention to error handling and security considerations to ensure the correctness and reliability of file operations.
The above is the detailed content of Getting Started with PHP File Processing: Detailed Basic Steps for Reading and Writing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

How to use pandas to read txt files correctly requires specific code examples. Pandas is a widely used Python data analysis library. It can be used to process a variety of data types, including CSV files, Excel files, SQL databases, etc. At the same time, it can also be used to read text files, such as txt files. However, when reading txt files, we sometimes encounter some problems, such as encoding problems, delimiter problems, etc. This article will introduce how to read txt correctly using pandas
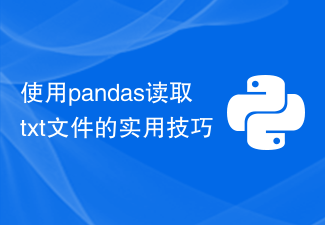
Practical tips for reading txt files using pandas, specific code examples are required. In data analysis and data processing, txt files are a common data format. Using pandas to read txt files allows for fast and convenient data processing. This article will introduce several practical techniques to help you better use pandas to read txt files, along with specific code examples. Reading txt files with delimiters When using pandas to read txt files with delimiters, you can use read_c
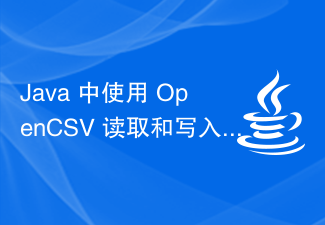
Example of using OpenCSV to read and write CSV files in Java. CSV (Comma-SeparatedValues) refers to comma-separated values and is a common data storage format. In Java, OpenCSV is a commonly used tool library for reading and writing CSV files. This article will introduce how to use OpenCSV to implement examples of reading and writing CSV files. Introducing the OpenCSV library First, you need to introduce the OpenCSV library to

Tips for solving Chinese garbled characters written by PHP into txt files. With the rapid development of the Internet, PHP, as a widely used programming language, is used by more and more developers. In PHP development, it is often necessary to read and write text files, including txt files that write Chinese content. However, due to encoding format problems, sometimes the written Chinese will appear garbled. This article will introduce some techniques to solve the problem of Chinese garbled characters written into txt files by PHP, and provide specific code examples. Problem analysis in PHP, text
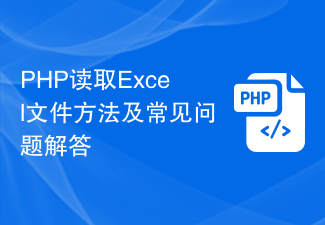
How to read Excel files with PHP and FAQs Excel is a very common spreadsheet file format, and many businesses and data are stored in Excel files. During the development process, if you need to import the data in the Excel file into the system, you need to use PHP to read the Excel file. This article will introduce how to read Excel files with PHP and answer common questions. 1. How to read Excel files with PHP 1. Use the PHPExcel class library PHPExcel is a P
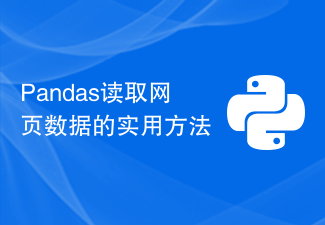
The practical method of reading web page data in Pandas requires specific code examples. During data analysis and processing, we often need to obtain data from web pages. As a powerful data processing tool, Pandas provides convenient methods to read and process web page data. This article will introduce several commonly used practical methods for reading web page data in Pandas, and attach specific code examples. Method 1: Use the read_html() function. Pandas’ read_html() function can read directly from the web page.
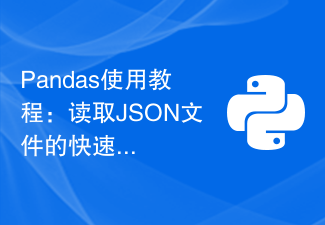
Quick Start: Pandas method of reading JSON files, specific code examples are required Introduction: In the field of data analysis and data science, Pandas is one of the important Python libraries. It provides rich functions and flexible data structures, and can easily process and analyze various data. In practical applications, we often encounter situations where we need to read JSON files. This article will introduce how to use Pandas to read JSON files, and attach specific code examples. 1. Installation of Pandas
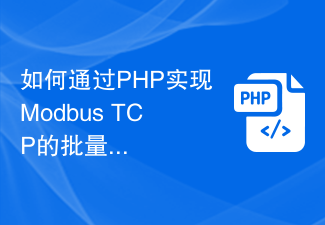
How to realize batch reading and writing of ModbusTCP through PHP 1. Introduction to ModbusTCP ModbusTCP is an industrial communication protocol based on TCP/IP protocol, which is commonly used for equipment communication in the field of industrial control. By using the ModbusTCP protocol, data reading and writing operations between devices can be achieved. This article will introduce the use of PHP language to implement batch reading and writing of ModbusTCP, and attach corresponding code examples. 2. Environment preparation at the beginning of compilation
