How to use PHP to implement user rights management
How to use PHP to implement user rights management
User rights management is a very important part of website development. It can be used to limit user access rights and protect sensitive data. and function. In PHP, user rights management can be achieved through some simple code.
1. Database design
First, we need to design a database table to store user and permission information. The following is a simple example table structure:
CREATE TABLE `users` ( `id` int(11) NOT NULL AUTO_INCREMENT, `username` varchar(50) NOT NULL, `password` varchar(255) NOT NULL, PRIMARY KEY (`id`) ); CREATE TABLE `permissions` ( `id` int(11) NOT NULL AUTO_INCREMENT, `permission` varchar(50) NOT NULL, PRIMARY KEY (`id`) ); CREATE TABLE `user_permissions` ( `id` int(11) NOT NULL AUTO_INCREMENT, `user_id` int(11) NOT NULL, `permission_id` int(11) NOT NULL, PRIMARY KEY (`id`) );
Stores user information in the users
table, including the user’s unique identifier id
, username
and encrypted password
.
Store permission information in the permissions
table, including the permission's unique identifier id
and the permission name permission
.
Storage the relationship between users and permissions in the user_permissions
table, including association identification id
, user ID user_id
and permission ID permission_id
.
2. User login and permission verification
- User login
User login is the prerequisite for obtaining user permissions. The following is an example login code:
<?php session_start(); // 数据库连接信息 $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "mydb"; $con = mysqli_connect($servername, $username, $password, $dbname); // 获取登录表单提交的用户名和密码 $username = $_POST["username"]; $password = $_POST["password"]; // 验证用户名和密码 $sql = "SELECT * FROM users WHERE username = '$username' AND password = '$password'"; $result = mysqli_query($con, $sql); $row = mysqli_fetch_assoc($result); if ($row) { // 用户登录成功,保存用户信息到 session $_SESSION["user_id"] = $row["id"]; } else { // 用户名或密码错误,返回登录页面或给出错误提示 } ?>
In the above code, first connect to the database through the mysqli_connect
function and obtain the username and password from the login form. Then use an SQL query to verify that the username and password entered by the user match those in the database. If the verification passes, use the $_SESSION
global variable to save the user's id
for subsequent use in permission verification.
- Permission verification
Once the user logs in successfully, we can verify the user's permissions through the following code:
<?php session_start(); // 验证用户是否登录 if (!isset($_SESSION["user_id"])) { // 如果用户未登录,根据需求,可以重定向到登录页面或给出错误提示 } // 获取用户的权限列表 $user_id = $_SESSION["user_id"]; $sql = "SELECT permissions.permission FROM user_permissions INNER JOIN permissions ON user_permissions.permission_id = permissions.id WHERE user_permissions.user_id = $user_id"; $result = mysqli_query($con, $sql); $permissions = array(); while ($row = mysqli_fetch_assoc($result)) { $permissions[] = $row["permission"]; } // 验证用户是否有指定权限 function hasPermission($permission) { global $permissions; return in_array($permission, $permissions); } ?>
In the above code, the user is first verified Are you logged in? If the user is not logged in, you can redirect to the login page or give an error prompt based on actual needs.
Then, query the database through the user's id
, obtain the user's permission list, and save it in an array. Finally, we can define a hasPermission
function that checks whether the user has the specified permissions.
3. Permission Control Example
The following is a simple example that demonstrates how to use user permissions to control website functions:
<?php require_once "auth.php"; // 验证用户是否有编辑文章的权限 if (hasPermission("edit_article")) { // 显示编辑文章的按钮 } ?>
In the above code, we have introduced the authentication code , and use the hasPermission
function to verify whether the user has the edit_article
permission. If the user has this permission, a button to edit the article will appear.
Summary:
Through the above code examples, we can see how to use PHP to implement user rights management. First, we need to design a database table to store user and permission information. Then, through user login and permission verification, we can restrict user access and protect sensitive data and functionality. Finally, the functional display of the website can be controlled according to the user's permissions.
The above is the detailed content of How to use PHP to implement user rights management. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


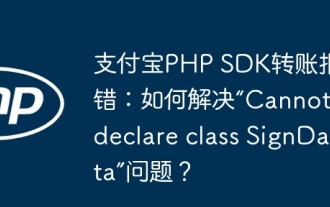
Alipay PHP...
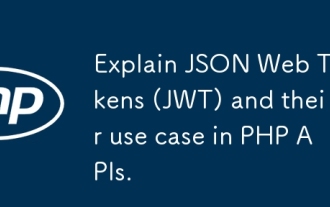
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
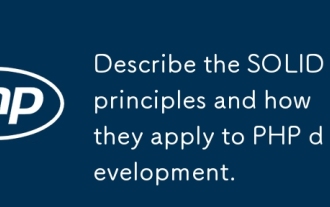
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
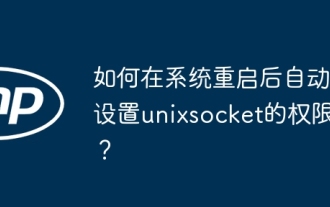
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
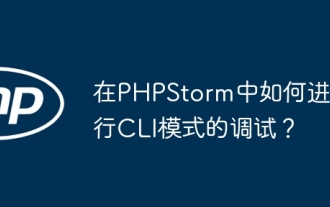
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
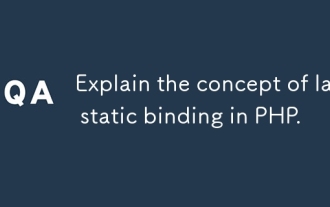
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
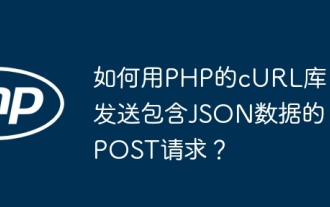
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
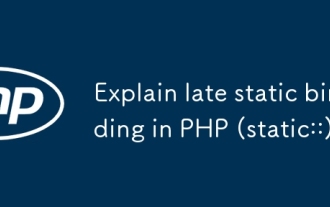
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
