How to implement cache control for RESTful API in PHP
How to implement cache control of RESTful API in PHP
When developing RESTful API, in order to improve performance and reduce server load, caching is a very important consideration . Through appropriate cache control, frequent queries to the database can be reduced, the response speed of the interface can be improved, and network bandwidth and server resources can be saved. This article will introduce how to implement cache control of RESTful API in PHP to provide better performance and stability.
- Using HTTP cache header information
In RESTful API, you can use HTTP header information to control caching. Commonly used header information includes:
- Cache-Control: Instructions to control the cache mechanism, such as
max-age
is used to set the maximum storage time of the cache,no-cache
Used to force resources to be reacquired for each request - Expires: Set the expiration time of the resource. Once it expires, the browser will re-request to update the resource
- Last-Modified: Identifies the last modification time of the resource
- ETag: The entity tag that identifies the resource. Once the resource changes, the ETag also changes
- If-Modified-Since: If the resource is updated after the specified date , then return the updated content
- If-None-Match: If the ETag of the resource is the same as the specified value, then return the 304 Not Modified status code
The following is a sample code to demonstrate How to set HTTP cache header information in PHP:
<?php // 检查是否已经缓存了响应 if(isset($_SERVER['HTTP_IF_MODIFIED_SINCE'])){ // 检查资源是否有更新 $lastModified = filemtime($file); $ifModifiedSince = strtotime($_SERVER['HTTP_IF_MODIFIED_SINCE']); if($lastModified <= $ifModifiedSince){ // 返回304 Not Modified状态码 header('HTTP/1.1 304 Not Modified'); exit; } } // 设置响应的Last-Modified和Cache-Control头信息 header('Last-Modified: '.gmdate('D, d M Y H:i:s', $lastModified).' GMT'); header('Cache-Control: public, max-age=3600'); // 输出响应内容 echo $response; ?>
- Using database cache
In addition to using HTTP header information to control caching, you can also use database cache to improve performance. The response results of the API can be stored in the database, and then the data can be obtained directly from the cache on the next request without the need to perform complex queries and calculations. This approach can significantly reduce database load and query time, and reduce dependence on external resources.
Here is a sample code that demonstrates how to use database caching in PHP:
<?php // 检查是否已经缓存了响应 if($cachedResponse = getCachedResponse($request)){ // 返回缓存的响应结果 echo $cachedResponse; exit; } // 执行复杂的查询和计算 $response = doExpensiveQuery($request); // 存储缓存的响应结果 storeCachedResponse($request, $response); // 输出响应内容 echo $response; ?>
- Using CDN Caching
Another commonly used cache control method is to use CDN (Content Delivery Network). CDN can cache API response results to nodes around the world to provide faster access and better reliability. You can choose to use a cloud service provider, such as AWS CloudFront or Fastly, to customize your caching strategy and manage your CDN.
Using CDN caching requires some configuration work, which usually involves setting cache header information, caching policies, caching rules, etc. For specific configuration steps, please refer to the documentation of the relevant CDN provider.
In summary, caching is crucial to improving the performance and stability of RESTful APIs. By properly setting HTTP header information and using database caching and CDN caching, the server burden can be effectively reduced, the interface response speed can be improved, and a better user experience can be provided. In actual development, choose an appropriate caching strategy according to specific needs to obtain the best performance and effect.
The above is the detailed content of How to implement cache control for RESTful API in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
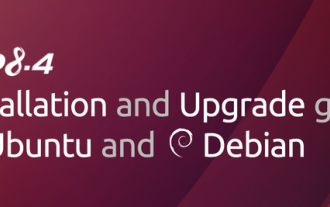
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
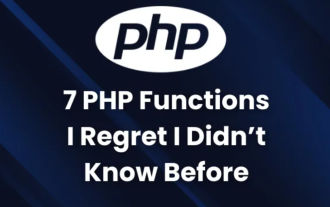
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
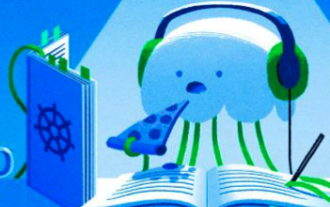
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
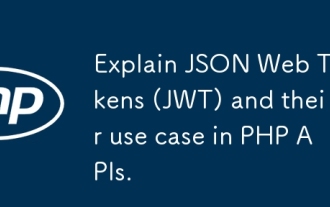
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
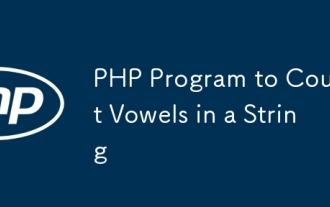
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
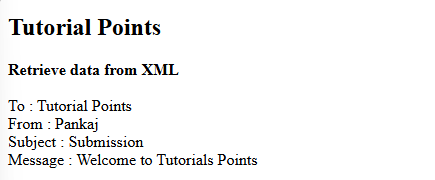
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
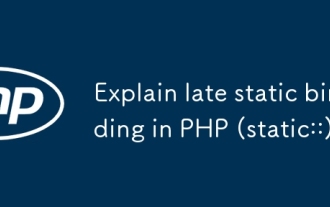
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
