


How to use API interfaces for data interaction and communication in PHP projects?
How to use API interfaces for data interaction and communication in PHP projects?
Overview:
In modern network application development, using API interfaces for data interaction and communication has become a common practice. Whether you are building a web application or a mobile application, exchanging data with the server through API interfaces can achieve front-end and back-end decoupling, improving development efficiency and flexibility. This article will introduce how to use API interfaces for data interaction and communication in PHP projects, and provide some practical code examples.
- Understand the concepts and principles of API interfaces
API (Application Programming Interface) refers to a set of methods and protocols provided by an application to allow other applications to access and use its functions and data . The API interface can provide different operations, such as obtaining data, storing data, updating data, etc. Common API interface formats include RESTful API, SOAP API, etc. - Use cURL library for API requests
cURL is a powerful open source library through which HTTP requests and data transmission can be conveniently made in PHP. Using the cURL library in a PHP project, you can easily connect to the API interface and send and receive data.
The following is a simple code example that shows how to send an HTTP GET request using the cURL library:
<?php // 初始化cURL $curl = curl_init(); // 设置请求的URL地址 curl_setopt($curl, CURLOPT_URL, 'http://example.com/api/data'); // 设置请求方式为GET curl_setopt($curl, CURLOPT_HTTPGET, true); // 执行HTTP请求并获取响应 $response = curl_exec($curl); // 关闭cURL资源和释放内存 curl_close($curl); // 处理响应数据 if ($response !== false) { // 处理API返回的数据 $data = json_decode($response, true); // 输出数据 print_r($data); } else { // 处理请求失败的情况 echo 'API请求失败'; } ?>
In the above example, we use curl_init()
The function initializes a cURL object and uses the curl_setopt()
function to set the requested URL address and request method to GET. Then use the curl_exec()
function to execute the HTTP request and save the response in the variable $response
. Finally, we use the json_decode()
function to decode the response data into a PHP array, process and output it.
- Use the API interface to perform data addition, deletion, modification and query operations
Through the API interface, we can implement data addition, deletion, modification and query operations. The specific operation methods and parameters vary according to the design of the API interface, and the corresponding settings need to be made according to the documentation and requirements of the API interface.
The following is a sample code that shows how to use the API interface to add, delete, modify and query data:
<?php // 数据查询 function get_data($id) { $url = 'http://example.com/api/data/' . $id; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HTTPGET, true); $response = curl_exec($curl); curl_close($curl); if ($response !== false) { $data = json_decode($response, true); return $data; } else { return false; } } // 数据添加 function add_data($data) { $url = 'http://example.com/api/data'; $post_data = json_encode($data); $headers = [ 'Content-Type: application/json', ]; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_POST, true); curl_setopt($curl, CURLOPT_POSTFIELDS, $post_data); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); $response = curl_exec($curl); curl_close($curl); if ($response !== false) { $result = json_decode($response, true); return $result; } else { return false; } } // 数据更新 function update_data($id, $data) { $url = 'http://example.com/api/data/' . $id; $put_data = json_encode($data); $headers = [ 'Content-Type: application/json', ]; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'PUT'); curl_setopt($curl, CURLOPT_POSTFIELDS, $put_data); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); $response = curl_exec($curl); curl_close($curl); if ($response !== false) { $result = json_decode($response, true); return $result; } else { return false; } } // 数据删除 function delete_data($id) { $url = 'http://example.com/api/data/' . $id; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_CUSTOMREQUEST, 'DELETE'); $response = curl_exec($curl); curl_close($curl); if ($response !== false) { $result = json_decode($response, true); return $result; } else { return false; } } // 使用示例 $data = get_data(1); print_r($data); $new_data = ['name' => 'John', 'age' => 30]; $result = add_data($new_data); print_r($result); $update_data = ['name' => 'John Doe', 'age' => 35]; $result = update_data(1, $update_data); print_r($result); $result = delete_data(1); print_r($result); ?>
In the above sample code, we use four functions:get_data()
is used to query data, add_data()
is used to add data, update_data()
is used to update data, delete_data()
Used to delete data. By setting different request methods and parameters, different operations can be achieved.
When using these functions, it is very important to ensure the correctness and security of the data. According to actual needs and API interface requirements, some identity verification, parameter verification and data processing operations can be performed to ensure data integrity and reliability.
Summary:
Data interaction and communication through API interfaces are common practices in modern web development. By using the cURL library to send HTTP requests, we can easily connect and interact with the server's API interface. At the same time, we can also perform data addition, deletion, modification and query operations through the API interface to achieve data management and processing. In actual applications, the request method and parameters need to be flexibly set according to specific needs and API interface requirements to meet the needs of the project.
(Note: The above example code is for reference only. In actual operation, the corresponding settings and adjustments need to be made according to the project needs and API interface requirements.)
The above is the detailed content of How to use API interfaces for data interaction and communication in PHP projects?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
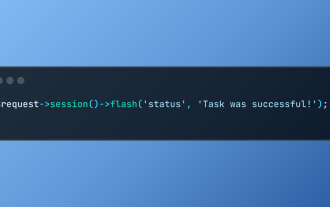
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
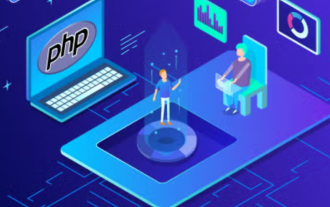
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
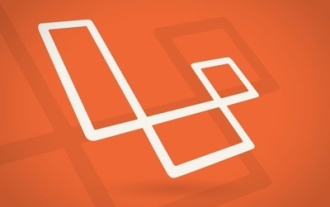
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
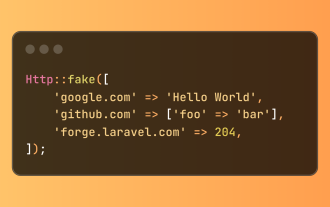
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
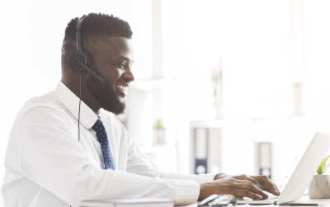
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
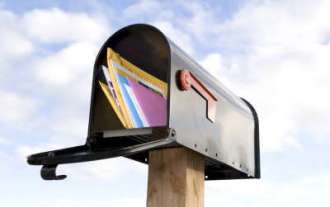
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
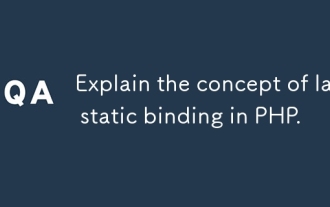
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
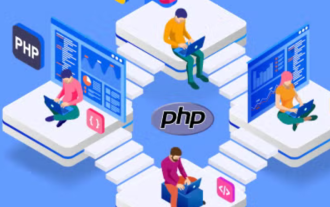
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
