How to build a simple CMS system using PHP
How to use PHP to build a simple CMS system
CMS (Content Management System) refers to the content management system, which can help us easily create, edit and manage Website content. In this article, we will introduce how to use PHP to build a simple CMS system to easily manage the content of the website.
Before we begin, we need to have a basic understanding and mastery of the following concepts:
- PHP: A server-side scripting language used to develop dynamic websites.
- MySQL: A database management system used to store and manage website data.
- HTML, CSS and JavaScript: used to build and beautify website pages.
Next, let’s build our simple CMS system step by step.
Step One: Create Database and Table
First, we need to create a MySQL database and create a table in the database to store the content of the website.
CREATE DATABASE cms; USE cms; CREATE TABLE articles ( id INT AUTO_INCREMENT PRIMARY KEY, title VARCHAR(255) NOT NULL, content TEXT NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
In the above code, we create a database named cms and create a table named articles in it. The table contains four fields: id, title, content and created_at, which are used to store the unique identifier, title, content and creation time of the article respectively.
Step 2: Connect to the database
Next, we need to connect to the database in the PHP file and establish a connection to the database.
$host = 'localhost'; $username = 'your_username'; $password = 'your_password'; $database = 'cms'; $conn = new mysqli($host, $username, $password, $database); if ($conn->connect_error) { die("连接失败:" . $conn->connect_error); } // 设置字符集 $conn->set_charset('utf8');
In the above code, we use the mysqli class to connect to the database. Please replace your_username
and your_password
with your own database username and password.
Step 3: Create a page
Next, we need to create a page for displaying the article list and adding new articles.
<?php // 查询所有文章 $sql = "SELECT * FROM articles"; $result = $conn->query($sql); ?> <!DOCTYPE html> <html> <head> <title>CMS</title> </head> <body> <h1>文章列表</h1> <ul> <?php while ($row = $result->fetch_assoc()): ?> <li> <h2><?php echo $row['title']; ?></h2> <p><?php echo $row['content']; ?></p> <p><?php echo $row['created_at']; ?></p> </li> <?php endwhile; ?> </ul> <h2>添加文章</h2> <form action="add_article.php" method="POST"> <input type="text" name="title" placeholder="标题"> <textarea name="content" placeholder="内容"></textarea> <button type="submit">提交</button> </form> </body> </html>
In the above code, we obtain the list of articles by querying the database, and use a loop to output the title, content and creation time of each article to the page. At the same time, we also created a form where users can enter the title and content of the new article, and the form submission action will be sent to the add_article.php
file.
Step 4: Add Article
Next, we need to create a page add_article.php
that handles adding new articles.
<?php $title = $_POST['title']; $content = $_POST['content']; $sql = "INSERT INTO articles (title, content) VALUES ('$title', '$content')"; if ($conn->query($sql) === TRUE) { echo "文章添加成功"; } else { echo "错误:" . $conn->error; } ?> <a href="index.php">返回</a>
In the above code, we use $_POST
to get the article title and content entered by the user in the form and insert it into the database. If the insertion is successful, we will output "Article added successfully", otherwise we will output an error message. At the same time, we also provide a link that users can click to return to the article list page.
So far, we have completed the construction of a simple CMS system. You can add and view articles through pages, realizing simple content management functions.
Summary:
By using PHP and MySQL, we can easily build a simple CMS system. This system helps us manage the content of our website, including adding, editing and displaying articles. By learning and understanding this simple CMS system, you can further extend and improve it to better suit your actual needs. I hope this article helps you build your own CMS system!
The above is the detailed content of How to build a simple CMS system using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


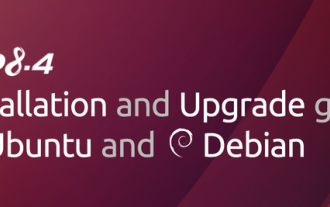
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
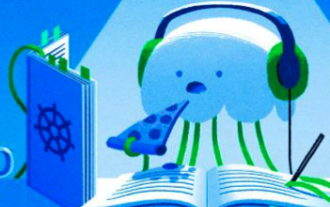
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
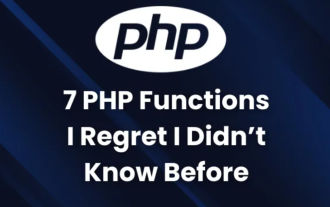
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
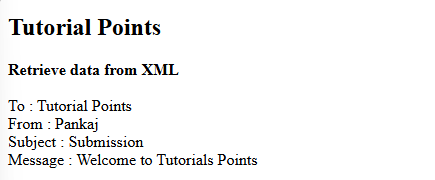
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
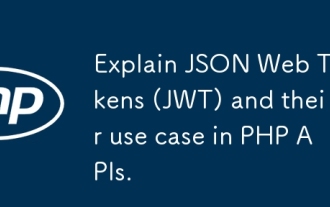
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
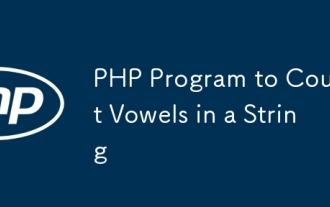
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
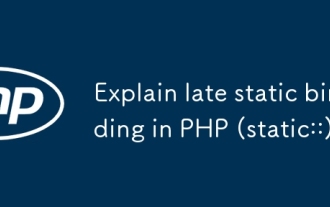
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
