Written in C++, find the number of subarrays whose sum is less than K
In this post, we will find the number of subarrays with sum less than K using C. In this problem, we have an array arr[] and an integer K. Now we need to find the subarrays whose sum is less than K. Below is the example −
Input : arr[] = {1, 11, 2, 3, 15} K = 10 Output : 4 {1}, {2}, {3} and {2, 3}
Methods to find the solution
Now we will use two different methods to solve the given problem-
Brute force cracking
In this method we will iterate through all sub-arrays and calculate their sum and if the sum is less than k then compare with k to increase our answer.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int arr[] = {1, 11, 2, 3, 15}; // given array int k = 10; // given k int size = sizeof(arr) / sizeof(int); // size of our array. int ans = 0; // counter variable. for(int i = 0; i < size; i++){ // outer loop. int sum = 0; for(int j = i; j < size; j++){ // inner loop. sum = sum + arr[j]; if(sum < k) // comparing with k. ans++; // incrementing our ans if sum is less than k. } } cout << ans << "\n"; return 0; }
Output
4
However, this method is not very good because its time complexity is very highO(N*N), where n is the size of the array.
We'll look for alternative solutions using the sliding window approach (which will help us reduce the time complexity of the program).
Efficient method
Different from brute force attack
Efficient method
strong>, we will not traverse all sub-arrays. Instead, only when the sum of the subarrays exceeds k , we iterate and move the left boundary to the right boundary and repeat until the entire array is traversed.
Example
#include <bits/stdc++.h> using namespace std; int main(){ int arr[] = {1, 11, 2, 3, 15}; // given array int k = 10; // given k int size = sizeof(arr) / sizeof(int); // size of our array. int ans = 0; // counter variable. int start = 0; // left border. int end = 0; // right border. int sum = 0; while(end < size && start < size){ // till the whole array is traversed. while(sum >= k && start < end){ sum = sum - arr[start]; start++; } if(end >= start) ans = ans + end - start; sum += arr[end]; end++; } cout << ans << "\n"; return 0; }
Output
4
We use Sliding Window Technique to make our program faster or better when dealing with larger constraints Efficient.
Explanation of the above code
In this approach we usually iterate until our sum is less than k and increment our answer based on it, this is the key change in the code that happens when the sum When it is greater than or equal to k. In this case, we start incrementing our left bound until it is less than or equal to k or the sum is greater than or equal to k. As our processing proceeds further, it iterates through other possible subarrays that may be formed. The sum of these new subarrays less than k is added to our answer, so our answer is incremented.
Compared with the previous brute force solution, this method is very efficient because its time complexity is O(N), where N is the number of our array size.
Conclusion
In this article, we used sliding window technique to solve the problem of finding the number of subarrays whose sum is less than k. We also learned the C program for this problem and our complete approach to solving it (both trivial and efficient). We can write the same program in other languages like C, Java, Python and others. Hope you find this article helpful.
The above is the detailed content of Written in C++, find the number of subarrays whose sum is less than K. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
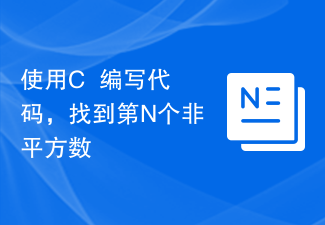
We all know numbers that are not the square of any number, such as 2, 3, 5, 7, 8, etc. There are N non-square numbers, and it is impossible to know every number. So, in this article, we will explain everything about squareless or non-square numbers and ways to find the Nth non-square number in C++. Nth non-square number If a number is the square of an integer, then the number is called a perfect square. Some examples of perfect square numbers are -1issquareof14issquareof29issquareof316issquareof425issquareof5 If a number is not the square of any integer, then the number is called non-square. For example, the first 15 non-square numbers are -2,3,5,6,
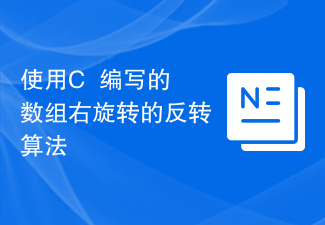
In this article, we will learn about the reversal algorithm to rotate a given array to the right by k elements, for example −Input:arr[]={4,6,2,6,43,7,3,7},k= 4Output:{43,7,3,7,4,6,2,6}Explanation:Rotatingeachelementofarrayby4-elementtotherightgives{43,7,3,7,4,6,2,6}.Input:arr[]={8 ,5,8,2,1,4,9,3},k=3Output:{4,9,3,8,5,8,2,1} Find the solution
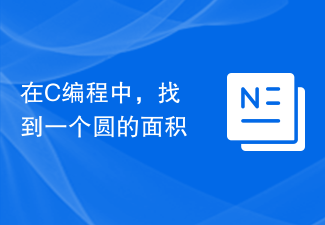
A circle is a closed figure. All points on a circle are equidistant from a point inside the circle. The center point is called the center of the circle. The distance from a point to the center of a circle is called the radius. Area is a quantitative representation of the span of dimensions of a closed figure. The area of a circle is the area enclosed within the dimensions of the circle. The formula to calculate the area of a circle, Area=π*r*r To calculate the area, we give the radius of the circle as input, we will use the formula to calculate the area, algorithm STEP1: Takeradiusasinputfromtheuserusingstdinput.STEP2: Calculatetheareaofcircleusing, area=(
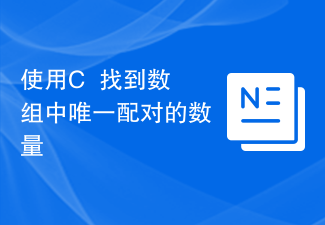
We need proper knowledge to create several unique pairs in array syntax of C++. While finding the number of unique pairs, we count all the unique pairs in the given array i.e. all possible pairs can be formed where each pair should be unique. For example -Input:array[]={5,5,9}Output:4Explanation:Thenumberoffalluniquepairsare(5,5),(5,9),(9,5)and(9,9).Input:array[]= {5,4,3,2,2}Output:16 Ways to Find Solution There are two ways to solve this problem, they are −
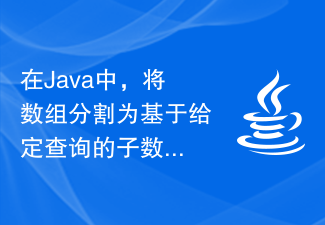
We have two arrays of integers, one with the calculated elements and the other with the split points required to split the array to generate subsets, we have to calculate the sum of each subset in each split and return the maximum subset Let's go through the example Understanding: - input −intarr[]=intarr[]={9,4,5,6,7}intsplitPoints[]={0,2,3,1}; output−the maximum subarray sum after each split [ 22,13,9,9] Explanation − Here we decompose the array according to its split points and get the maximum subset after each split and after the first split → {9} and {4,5,6,7 }>>The maximum sum of subarrays is -22 after the second split→{9},{4
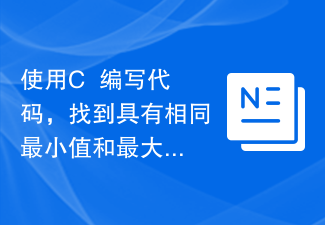
In this article, we will use C++ to solve the problem of finding the number of subarrays whose maximum and minimum values are the same. The following is an example of the problem −Input:array={2,3,6,6,2,4,4,4}Output:12Explanation:{2},{3},{6},{6},{2 },{4},{4},{4},{6,6},{4,4},{4,4}and{4,4,4}arethesubarrayswhichcanbeformedwithmaximumandminimumelementsame.Input:array={3,3, 1,5,
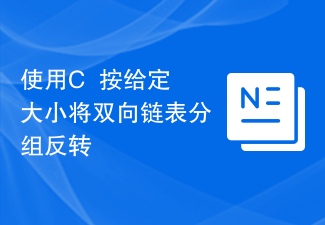
In this problem, we are given a pointer to the head of the linked list and an integer k. In a group of size k, we need to reverse the linked list. For example -Input:1<->2<->3<->4<->5(doublylinkedlist),k=3Output:3<->2<->1<->5<->4 looking for solutions Method In this problem, we will formulate a recursive algorithm to solve this problem. In this method we will use recursion and solve the problem using recursion. Example#include<iostream&
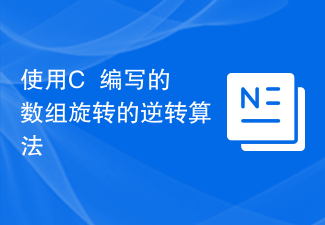
In the given problem, we have an array and we need to rotate the array by d elements using inversion algorithm like −Input:arr[]=[1,2,3,4,5,6,7], d=2Output:arr[]=[3,4,5,6,7,1,2]Explanation:Asyoucanseewehavetorotatethisarraybyd=2butourmaintaskistoachievethisbyusingareversaltechnique. We performed some inversion technique calculations on the rotation of the array and concluded: First, we reverse
