C program to find length of linked list
Linked lists use dynamic memory allocation, i.e. they grow and shrink accordingly. They are defined as collections of nodes. Here, a node has two parts, data and links. The representation of data, links and linked lists is as follows -
Types of linked lists
There are four types of linked lists, as follows: -
- Singly linked list/Singly linked list
- Double/double linked list
- Cycling singly linked list
- Cycling double linked list
We use the recursive method to find the length of the linked list The logic is -
int length(node *temp){ if(temp==NULL) return l; else{ l=l+1; length(temp->next); } }
Program
The following is a C program to find the length of a linked list-
Live demonstration
#include#include typedef struct linklist{ int data; struct linklist *next; }node; int l=0; int main(){ node *head=NULL,*temp,*temp1; int len,choice,count=0,key; do{ temp=(node *)malloc(sizeof(node)); if(temp!=NULL){ printf(" enter the elements in a list : "); scanf("%d",&temp->data); temp->next=NULL; if(head==NULL){ head=temp; }else{ temp1=head; while(temp1->next!=NULL){ temp1=temp1->next; } temp1->next=temp; } }else{ printf("
Memory is full"); } printf("
press 1 to enter data into list: "); scanf("%d",&choice); }while(choice==1); len=length(head); printf("The list has %d no of nodes",l); return 0; } //recursive function to find length int length(node *temp){ if(temp==NULL) return l; else{ l=l+1; length(temp->next); } }
Output
When When executing the above program, the following results are produced -
Run 1: enter the elements in a list: 3 press 1 to enter data into list: 1 enter the elements in a list: 56 press 1 to enter data into list: 1 enter the elements in a list: 56 press 1 to enter data into list: 0 The list has 3 no of nodes Run 2: enter the elements in a list: 12 press 1 to enter data into list: 1 enter the elements in a list: 45 press 1 to enter data into list: 0 The list has 2 no of nodes
The above is the detailed content of C program to find length of linked list. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
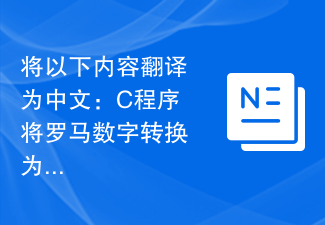
Given below is a C language algorithm to convert Roman numerals to decimal numbers: Algorithm Step 1 - Start Step 2 - Read Roman numerals at runtime Step 3 - Length: = strlen(roman) Step 4 - For i=0 to Length-1 Step 4.1-switch(roman[i]) Step 4.1.1-case'm': &nbs
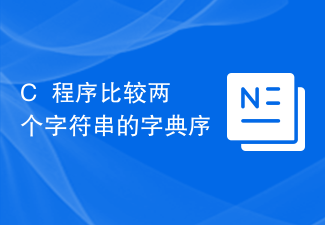
Lexicographic string comparison means that strings are compared in dictionary order. For example, if there are two strings 'apple' and 'appeal', the first string will come last because the first three characters of 'app' are the same. Then for the first string the character is 'l' and in the second string the fourth character is 'e'. Since 'e' is shorter than 'l', it will come first if we sort lexicographically. Strings are compared lexicographically before being arranged. In this article, we will see different techniques for lexicographically comparing two strings using C++. Using the compare() function in C++ strings The C++string object has a compare()
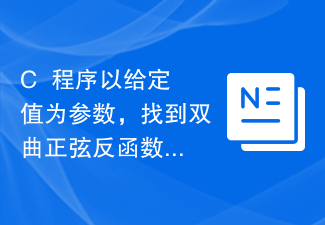
Hyperbolic functions are defined using hyperbolas instead of circles and are equivalent to ordinary trigonometric functions. It returns the ratio parameter in the hyperbolic sine function from the supplied angle in radians. But do the opposite, or in other words. If we want to calculate an angle from a hyperbolic sine, we need an inverse hyperbolic trigonometric operation like the hyperbolic inverse sine operation. This course will demonstrate how to use the hyperbolic inverse sine (asinh) function in C++ to calculate angles using the hyperbolic sine value in radians. The hyperbolic arcsine operation follows the following formula -$$\mathrm{sinh^{-1}x\:=\:In(x\:+\:\sqrt{x^2\:+\:1})}, Where\:In\:is\:natural logarithm\:(log_e\:k)
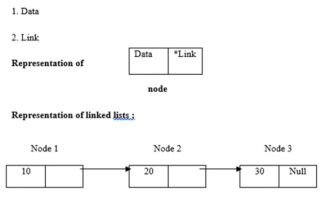
Linked lists use dynamic memory allocation, i.e. they grow and shrink accordingly. They are defined as collections of nodes. Here, a node has two parts, data and links. The representation of data, links and linked lists is as follows - Types of linked lists There are four types of linked lists, as follows: - Single linked list/Singly linked list Double/Doubly linked list Circular single linked list Circular double linked list We use the recursive method to find the length of the linked list The logic is -intlength(node *temp){ if(temp==NULL) returnl; else{&n
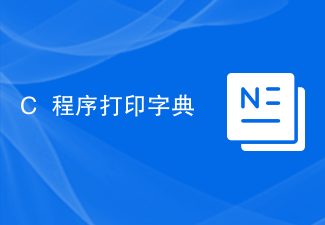
A map is a special type of container in C++ in which each element is a pair of two values, namely a key value and a map value. The key value is used to index each item, and the mapped value is the value associated with the key. Regardless of whether the mapped value is unique, the key is always unique. To print map elements in C++ we have to use iterator. An element in a set of items is indicated by an iterator object. Iterators are primarily used with arrays and other types of containers (such as vectors), and they have a specific set of operations that can be used to identify specific elements within a specific range. Iterators can be incremented or decremented to reference different elements present in a range or container. The iterator points to the memory location of a specific element in the range. Printing a map in C++ using iterators First, let's look at how to define
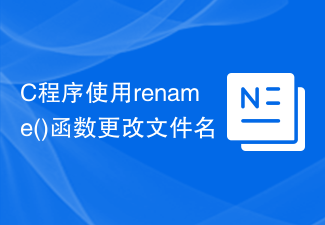
The rename function changes a file or directory from its old name to its new name. This operation is similar to the move operation. So we can also use this rename function to move files. This function exists in the stdio.h library header file. The syntax of the rename function is as follows: intrename(constchar*oldname,constchar*newname); The function of the rename() function accepts two parameters. One is oldname and the other is newname. Both parameters are pointers to constant characters that define the old and new names of the file. Returns zero if the file was renamed successfully; otherwise, returns a nonzero integer. During a rename operation
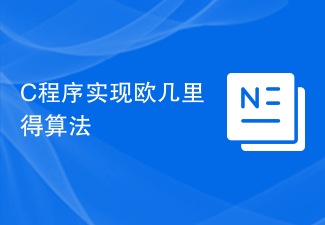
The problem implements Euclidean's algorithm to find the greatest common divisor (GCD) and least common multiple (LCM) of two integers and outputs the results with a given integer. Solution The solution to implement Euclidean algorithm to find the greatest common divisor (GCD) and least common multiple (LCM) of two integers is as follows - the logic of finding GCD and LCM is as follows - if (firstno*secondno!=0){ gcd= gcd_rec(firstno,secondno); printf("TheGCDof%dand%dis%d",
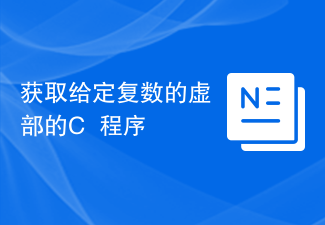
Modern science relies heavily on the concept of plural numbers, which was first established in the early 17th century by Girolamo Cardano, who introduced it in the 16th century. The formula for complex numbers is a+ib, where a holds the html code and b is a real number. A complex number is said to have two parts: the real part <a> and the imaginary part (<ib>). The value of i or iota is √-1. The plural class in C++ is a class used to represent complex numbers. The complex class in C++ can represent and control several complex number operations. Let's take a look at how to represent and control the display of plural numbers. imag() member function As mentioned above, complex numbers are composed of real part and imaginary part. To display the real part we use real()
