


PHP's object-oriented programming paradigm offers advantages to project management and organizations
PHP’s object-oriented programming paradigm provides advantages for project management and organization
With the rapid development of the Internet, websites and applications of all sizes have sprung up. come out. In order to meet the growing needs and improve development efficiency and maintainability, the use of object-oriented programming (OOP) has become the mainstream of modern software development. In dynamic scripting languages like PHP, OOP brings many advantages to project management and organization. This article will introduce some of them and give corresponding code examples.
- Code Reuse and Modularization
Object-oriented programming organizes code by using the concepts of classes and objects. A class is an abstract data type that encapsulates properties and methods. Objects are instances of classes, and multiple objects can be created through classes. This approach makes code reusable and easy to maintain.
The following is a simple example that presents a class named Person and the process of creating an object of that class and accessing its properties and methods.
class Person { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function getName() { return $this->name; } public function getAge() { return $this->age; } } $person = new Person("John", 30); echo $person->getName(); // 输出 "John" echo $person->getAge(); // 输出 30
In this example, the Person class encapsulates a person's name (name) and age (age), and provides methods to obtain the name and age. By creating an object of Person class, we can easily access and modify these properties without having to write the same code repeatedly.
- Encapsulation and information hiding
Another important concept in object-oriented programming is encapsulation and information hiding. By encapsulating data and methods in classes, we can control access to these data and methods and provide a public interface for other objects to use. In this way, we can hide implementation details and expose only necessary interfaces, thus improving security and reducing unnecessary dependencies.
The following is a simple example showing the application of encapsulation and information hiding in PHP.
class BankAccount { private $balance; public function deposit($amount) { $this->balance += $amount; } public function withdraw($amount) { if ($amount > $this->balance) { throw new Exception("Insufficient balance"); } $this->balance -= $amount; } public function getBalance() { return $this->balance; } } $account = new BankAccount(); $account->deposit(100); $account->withdraw(50); echo $account->getBalance(); // 输出 50
In this example, the BankAccount class represents a bank account, encapsulating the private property balance and the public methods deposit, withdraw and getBalance. Through encapsulation, we can ensure that the balance can only be modified through the deposit and withdraw methods, thus ensuring the security of the account.
- Inheritance and Polymorphism
Inheritance and polymorphism are two important concepts in object-oriented programming. Inheritance allows us to create a new class and inherit properties and methods from an existing class. Doing so reduces the workload of rewriting code and makes it easy to add or modify functionality.
Polymorphism means that in the inheritance relationship, the subclass can have its own implementation, and the method of the parent class can receive the subclass object as a parameter and call the relevant method correctly. This flexibility improves code scalability and maintainability.
The following is a simple example showing the application of inheritance and polymorphism in PHP.
class Animal { public function makeSound() { echo "Animal makes sound"; } } class Dog extends Animal { public function makeSound() { echo "Dog barks"; } } class Cat extends Animal { public function makeSound() { echo "Cat meows"; } } $animal = new Animal(); $dog = new Dog(); $cat = new Cat(); $animal->makeSound(); // 输出 "Animal makes sound" $dog->makeSound(); // 输出 "Dog barks" $cat->makeSound(); // 输出 "Cat meows"
In this example, the Animal class is a base class, and the Dog and Cat classes inherit from Animal. Each class overrides the makeSound method to provide its own implementation. When the makeSound method is called, the corresponding subclass method will be called according to the type of the object, realizing polymorphism.
Summary:
Through the above examples, we can clearly see that PHP’s object-oriented programming paradigm provides many advantages for project management and organization. Code reuse and modularization enable developers to write code more efficiently; encapsulation and information hiding improve security and maintainability; while inheritance and polymorphism increase code scalability and flexibility. Therefore, when developing large-scale projects, we should make full use of PHP's object-oriented programming features to improve development efficiency and code quality.
The above is the detailed content of PHP's object-oriented programming paradigm offers advantages to project management and organizations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


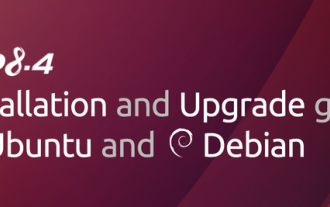
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
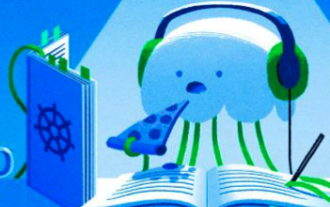
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
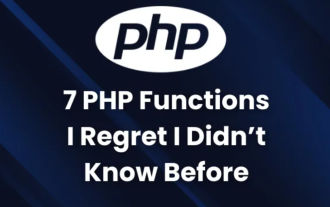
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
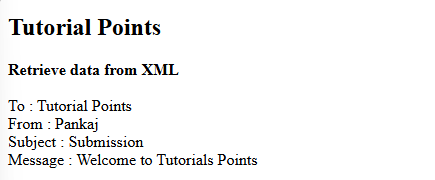
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
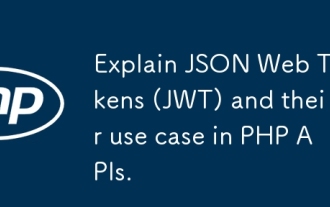
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
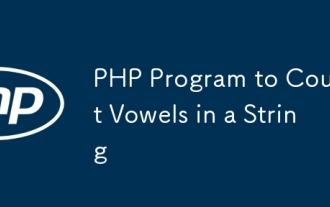
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
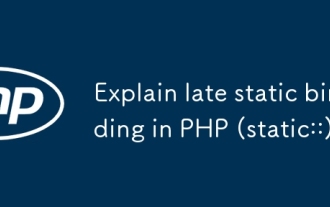
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
