C++ program access private members of class
Private members of a class can only be accessed by members of the class. This is done to maintain object-oriented encapsulation principles, ensuring that data and its associated functions are kept in a single unit and can only be accessed from members of the class. C has three different access controls to specify the visibility of members of a class. These three access control characters are −
Public − If the members of a class have public visibility, then these members can be accessed from any other class.
Private − Class members with private visibility can only be accessed from within the class.
Protected − protected class members can be accessed from with9in the class or from its subclasses only.
For this article, we will only focus on accessing private members of a class.
Use getter and setter methods to access data members
The Getter and setter functions are used to access and modify private members of the class. As the name suggests, the getter function returns the data member, while the setter function is used to "set" or modify the data member. We use two examples to further understand this concept, but before that, the basic syntax is given below.
grammar
Getter/ Accessor functions −
private: <datatype> value; public: <datatype> getterFunction() { return <datatype> this->value; }
Setter/Mutator function−
private: <datatype> value; public: void setterFunction(<datatype> _value) { this->value = _value; }
Example
is:Example
#include <iostream> using namespace std; class Test{ private: int value; public: //the getter function int getValue() { return this->value; } //the setter function void setValue(int _value) { this->value = _value; } }; int main(){ Test test; test.setValue(15); cout << "The value we set is: " << test.getValue() << endl; return 0; }
Output
The value we set is: 15
Accessing member functions from within another function
The situation is the same when we access a private member function. We have to access it from inside the class member method in the same way as we access the data members. We can use the "this" pointer to avoid name conflicts.
grammar
private: <returntype> function_name(params) {}; public: <returntype> another_function(params) { <datatype> var = this->function_name(arguments); }
Functions that call private member functions should be declared public. The public function will only be executed when called from an object of this class.
The Chinese translation ofExample
is:Example
#include <iostream> using namespace std; class Test{ private: int value; //multiplies the member value by 10 void multiplyValue() { this->value = this->value * 10; } public: //the getvalue function calls the multiply value function int multiplyAndGetValue() { this->multiplyValue(); return this->value; } //the setter function void setValue(int _value) { this->value = _value; } }; int main(){ Test test; test.setValue(15); cout << "The value after setting and multiplying is: " << test.multiplyAndGetValue() << endl; return 0; }
Output
The value after setting and multiplying is: 150
Use friend classes
In C, a friend class is a class that can access private and protected members of other classes that are not visible to other classes. To declare a class as a friend of another class, use the keyword ‘friend’. Let's see how it works.
grammar
class A{ private: ..... friend class B; }; class B{ //class body };
Example
is:Example
#include <iostream> using namespace std; class Test1{ private: int value; public: Test1(int _value) { this->value = _value; } //we declare another class as a friend friend class Test2; }; class Test2{ public: //displays the value of the other class object void display(Test1 &t) { cout << "The value of Test1 object is: " << t.value; } }; int main(){ //creating two class objects of the two classes Test1 test1(15); Test2 test2; //calling the friend class function test2.display(test1); return 0; }
Output
The value of Test1 object is: 15
Use friend function
In C, friend functions are similar to friend classes. Here, we can declare a specific function that is not a member of the class as a "friend" and it will get access to the private members of the class. Let's look at the syntax of how to define a function as "Friend".
grammar
class A{ private: ..... friend <return_type> function_name(params); }; <return_type> function_name(params) { //function body }
Example
is:Example
#include <iostream> using namespace std; class Test1{ private: int value; public: Test1(int _value) { this->value = _value; } //we declare a friend function friend void display(Test1); }; void display(Test1 t) { cout << "The value of Test1 object is: " << t.value; } int main(){ //creating two class objects of the two classes Test1 test1(55); //calling the friend class function display(test1); return 0; }
Output
The value of Test1 object is: 55
in conclusion
When we access private data members of a class, it is best to use accessor/getter and modifier/setter functions. This is the safest way to access data members of a class. One thing to always remember is that functions that access private members should be declared public. Friend functions are not available in other object-oriented languages because this does not always preserve the properties of object-oriented encapsulation. The friend relationship is asymmetric. If class A declares class B as a friend, then class B will have access to all members of A, but A will not be able to access all private members of B.
The above is the detailed content of C++ program access private members of class. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


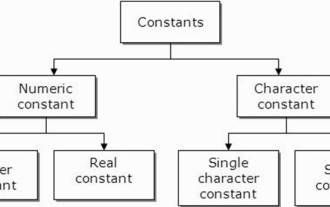
A constant is also called a variable and once defined, its value does not change during the execution of the program. Therefore, we can declare a variable as a constant referencing a fixed value. It is also called text. Constants must be defined using the Const keyword. Syntax The syntax of constants used in C programming language is as follows - consttypeVariableName; (or) consttype*VariableName; Different types of constants The different types of constants used in C programming language are as follows: Integer constants - For example: 1,0,34, 4567 Floating point constants - Example: 0.0, 156.89, 23.456 Octal and Hexadecimal constants - Example: Hex: 0x2a, 0xaa.. Octal
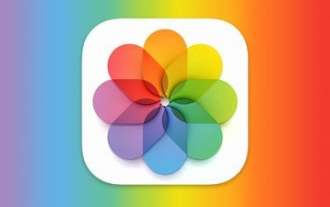
In iOS17, Apple has more control over what apps can see in photos. Read on to learn how to manage app access by app. In iOS, Apple's in-app photo picker lets you share specific photos with the app, while the rest of your photo library remains private. Apps must request access to your entire photo library, and you can choose to grant the following access to apps: Restricted Access – Apps can only see images that you can select, which you can do at any time in the app or by going to Settings > ;Privacy & Security>Photos to view selected images. Full access – App can view photos
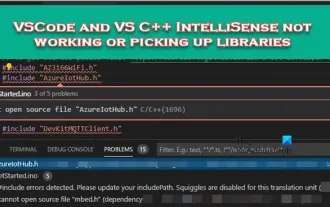
VS Code and Visual Studio C++ IntelliSense may not be able to pick up libraries, especially when working on large projects. When we hover over #Include<wx/wx.h>, we see the error message "CannotOpen source file 'string.h'" (depends on "wx/wx.h") and sometimes, autocomplete Function is unresponsive. In this article we will see what you can do if VSCode and VSC++ IntelliSense are not working or extracting libraries. Why doesn't my Intellisense work in C++? When working with large files, IntelliSense sometimes
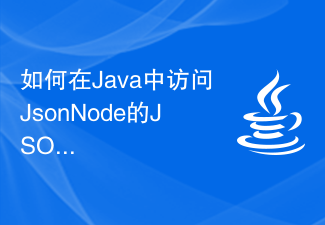
A JsonNode is Jackson's JSON tree model that can read JSON into JsonNode instances and write JsonNode into JSON. We can use Jackson to read JSON into a JsonNode by creating an ObjectMapper instance and calling the readValue() method. We can access fields, arrays or nested objects using the get() method of the JsonNode class. We can use the asText() method to return a valid string representation and convert the node's value to Javaint using the asInt() method of the JsonNode class. In the example below we can access Json
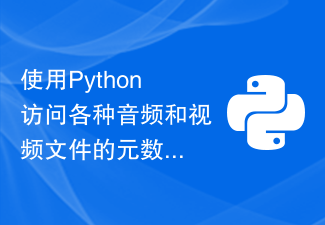
We can access the metadata of audio files using Mutagen and the eyeD3 module in Python. For video metadata we can use movies and the OpenCV library in Python. Metadata is data that provides information about other data, such as audio and video data. Metadata for audio and video files includes file format, file resolution, file size, duration, bitrate, etc. By accessing this metadata, we can manage media more efficiently and analyze the metadata to obtain some useful information. In this article, we will take a look at some of the libraries or modules provided by Python for accessing metadata of audio and video files. Access audio metadata Some libraries for accessing audio file metadata are - using mutagenesis
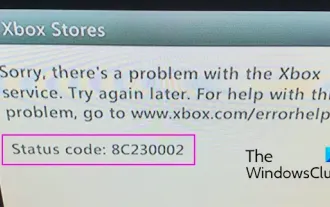
Are you unable to purchase or watch content on your Xbox due to error code 8C230002? Some users keep getting this error when trying to purchase or watch content on their console. Sorry, there's a problem with the Xbox service. Try again later. For help with this issue, visit www.xbox.com/errorhelp. Status Code: 8C230002 This error code is usually caused by temporary server or network problems. However, there may be other reasons, such as your account's privacy settings or parental controls, that may prevent you from purchasing or viewing specific content. Fix Xbox Error Code 8C230002 If you receive error code 8C when trying to watch or purchase content on your Xbox console
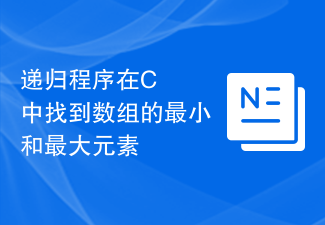
We take the integer array Arr[] as input. The goal is to find the largest and smallest elements in an array using a recursive method. Since we are using recursion, we will iterate through the entire array until we reach length = 1 and then return A[0], which forms the base case. Otherwise, the current element is compared to the current minimum or maximum value and its value is updated recursively for subsequent elements. Let’s look at various input and output scenarios for this −Input −Arr={12,67,99,76,32}; Output −Maximum value in the array: 99 Explanation &mi
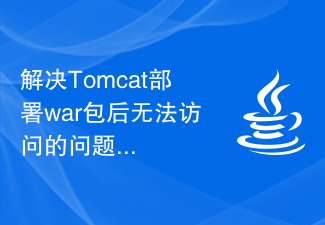
How to solve the problem that Tomcat cannot successfully access the war package after deploying it requires specific code examples. As a widely used Java Web server, Tomcat allows developers to package their own developed Web applications into war files for deployment. However, sometimes we may encounter the problem of being unable to successfully access the war package after deploying it. This may be caused by incorrect configuration or other reasons. In this article, we'll provide some concrete code examples that address this dilemma. 1. Check Tomcat service
