JavaScript program to check if matrix is upper triangular
An upper triangular matrix is a square matrix with the same number of rows and columns and all elements below the main diagonal from the first cell (located in the upper left corner) to The last cell (in the upper left corner, lower right corner) is zero. The upper triangle means that the elements present in the lower triangle will be zero. We will implement a proper code and the time and space complexities will be explained and discussed.
Example
Input1: mat = [ [ 1, 2, 3, 4], [ 0, 5, 6, 7], [ 0, 0, 8, 9], [ 0, 0, 0, 1] ] Output1: Yes,
Explanation: We can see that the main diagonal contains elements 1, 5, 8 and 1, and all cells below the main diagonal have values of zero.
Input2: mat = [ [ 1, 2, 3, 4], [ 0, 5, 6, 7], [ 0, 0, 8, 9], [ 0, 1, 0, 1] ] Output1: No
Explanation: We can see that the main diagonal contains elements 1, 5, 8 and 1, and all cells below the main diagonal have non-zero values because the second column of the last row contains non-zero value value.
method
We have seen the example above, now let’s look at the steps to implement the code:
First, we will create a function in which we pass the given matrix. We will traverse only the lower part of the main diagonal of the matrix, i.e. each cell (i,j) where j is less than i. If we find any cell with a non-zero value, we will return false, otherwise we will eventually return true.
Example
// function to traverse over the matrix function check(mat){ // getting total number of rows of matrix var rows = mat.length // traversing over the section present above the main diagonal for(var i = 0; i < rows; i++){ for(var j = 0; j < i; j++){ if(mat[i][j] != 0){ return false; } } } return true; } // defining the matrix var mat = [ [ 1, 2, 3, 4], [ 0, 5, 6, 7], [ 0, 0, 8, 9], [ 0, 0, 0, 1] ] // given matrix console.log("The given matrix is: "); console.log(mat) if(check(mat)){ console.log("The given matrix is an upper triangular matrix"); } else{ console.log("The given matrix is not an upper triangular matrix"); } // updating matrix mat = [ [ 1, 2, 3, 4], [ 0, 5, 6, 7], [ 0, 0, 8, 9], [ 0, 1, 0, 1] ] // given matrix console.log("The given matrix is: "); console.log(mat) if(check(mat)){ console.log("The given matrix is an upper triangular matrix"); } else{ console.log("The given matrix is not an upper triangular matrix"); }
Output
The given matrix is: [ [ 1, 2, 3, 4 ], [ 0, 5, 6, 7 ], [ 0, 0, 8, 9 ], [ 0, 0, 0, 1 ] ] The given matrix is an upper triangular matrix The given matrix is: [ [ 1, 2, 3, 4 ], [ 0, 5, 6, 7 ], [ 0, 0, 8, 9 ], [ 0, 1, 0, 1 ] ] The given matrix is not an upper triangular matrix
Time and space complexity
The time complexity of the above code is O(N*N), where N is the number of rows of the given matrix. This is because we only iterate through the matrix once.
The space complexity of the above code is O(1) because we are not using any extra space.
in conclusion
In this tutorial, we implemented a JavaScript program to check whether a given matrix is an upper triangular matrix. The upper triangle means that the elements present in the lower triangle will be zero. We iterate over the cells in the matrix where the number of columns is less than the number of rows, with time complexity O(N*N) and space complexity O(1).
The above is the detailed content of JavaScript program to check if matrix is upper triangular. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


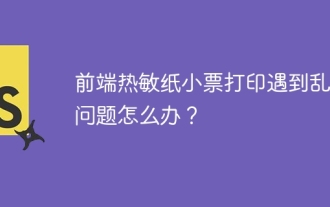
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
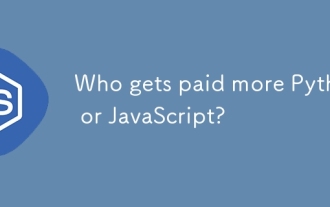
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
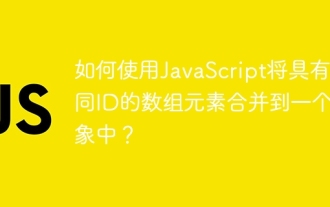
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
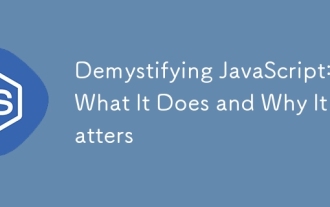
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
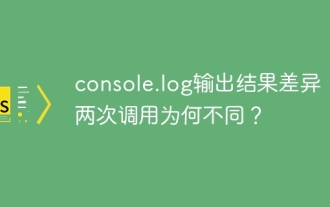
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
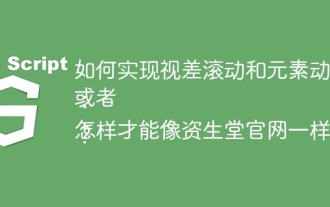
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
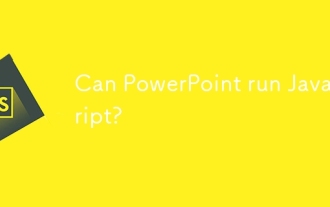
JavaScript can be run in PowerPoint, and can be implemented by calling external JavaScript files or embedding HTML files through VBA. 1. To use VBA to call JavaScript files, you need to enable macros and have VBA programming knowledge. 2. Embed HTML files containing JavaScript, which are simple and easy to use but are subject to security restrictions. Advantages include extended functions and flexibility, while disadvantages involve security, compatibility and complexity. In practice, attention should be paid to security, compatibility, performance and user experience.
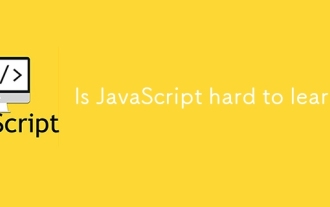
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
