


Detailed explanation of the underlying development principles of PHP: object-oriented programming and class implementation
Detailed explanation of the underlying development principles of PHP: object-oriented programming and class implementation
With the development of Web development, PHP, as a commonly used server-side scripting language, has gradually It has attracted the attention and use of a large number of developers. The underlying development principles of PHP are part of what PHP developers must master, especially object-oriented programming and class implementation. This article will introduce in detail the implementation principles of object-oriented programming and classes in the underlying development of PHP, and give relevant code examples.
1. Object-oriented programming (OOP)
Object-oriented programming is a programming paradigm that encapsulates data and operations on data to form objects. In PHP, we can define a class through the class keyword. A class is an abstract data type used to describe the structure and behavior of an object.
- Class definition
The following is a simple class definition example:
class Car { public $brand; public $color; public function drive() { echo "I am driving a " . $this->color . " " . $this->brand . "."; } }
In the above example, we define a class named Car class, which has two properties (brand and color) and one method (drive). Properties are characteristics of a class, while methods are behavior of a class.
- Creation and use of objects
Creating an object requires using the new keyword to instantiate a class. The following is an example of using the Car class to create an object and call methods:
$myCar = new Car(); $myCar->brand = "Honda"; $myCar->color = "red"; $myCar->drive(); // 输出:I am driving a red Honda.
The above code first uses the new keyword to create a Car object named $myCar, and then assigns values to the object's attributes brand and color respectively. , and finally call the object's method drive().
2. Implementation principles of classes
- Member access control of classes
In PHP, we can use public, protected and private Access modifiers control access to members of a class.
- public: Public members can be accessed from anywhere.
- protected: Protected members can only be accessed within the class and subclasses.
- private: Private members can only be accessed within the class.
- Constructor and destructor methods
Constructor and destructor are special methods in a class.
- The construction method is defined using __construct(), which is used to initialize the object when it is created.
- The destructor method is defined using __destruct(), which is used to clean up the object when it is destroyed.
The following is an example with a constructor and destructor:
class Person { private $name; public function __construct($name) { $this->name = $name; echo "Hello, I am " . $this->name . "."; } public function __destruct() { echo "Goodbye, " . $this->name . "."; } } $person = new Person("John"); // 输出:Hello, I am John.
In the above example, we created a class named Person, and the constructor receives a parameter $name and assign it to the private property name of the class. When a Person object is created, the constructor method is automatically called and the corresponding prompt is output.
3. Summary
Object-oriented programming and class implementation are a very important part of the underlying development of PHP. Through the introduction of this article, we have learned about the basic concepts of object-oriented programming and the definition of classes, as well as the use of class member access control, construction methods, and destructor methods.
In actual development, reasonable use of object-oriented programming and class implementation can improve the readability and maintainability of the code. At the same time, understanding the underlying development principles of PHP is also very helpful for us to deeply understand the operating mechanism of PHP and optimize the code.
I hope this article can be helpful to the learning and practice of PHP's underlying development principles.
The above is the detailed content of Detailed explanation of the underlying development principles of PHP: object-oriented programming and class implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
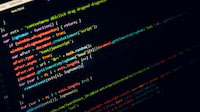
Introduction In today's rapidly evolving digital world, it is crucial to build robust, flexible and maintainable WEB applications. The PHPmvc architecture provides an ideal solution to achieve this goal. MVC (Model-View-Controller) is a widely used design pattern that separates various aspects of an application into independent components. The foundation of MVC architecture The core principle of MVC architecture is separation of concerns: Model: encapsulates the data and business logic of the application. View: Responsible for presenting data and handling user interaction. Controller: Coordinates the interaction between models and views, manages user requests and business logic. PHPMVC Architecture The phpMVC architecture follows the traditional MVC pattern, but also introduces language-specific features. The following is PHPMVC
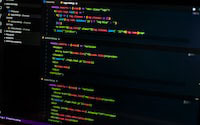
SOLID principles are a set of guiding principles in object-oriented programming design patterns that aim to improve the quality and maintainability of software design. Proposed by Robert C. Martin, SOLID principles include: Single Responsibility Principle (SRP): A class should be responsible for only one task, and this task should be encapsulated in the class. This can improve the maintainability and reusability of the class. classUser{private$id;private$name;private$email;publicfunction__construct($id,$nam
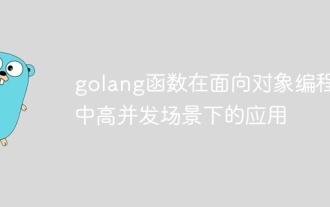
In high-concurrency scenarios of object-oriented programming, functions are widely used in the Go language: Functions as methods: Functions can be attached to structures to implement object-oriented programming, conveniently operating structure data and providing specific functions. Functions as concurrent execution bodies: Functions can be used as goroutine execution bodies to implement concurrent task execution and improve program efficiency. Function as callback: Functions can be passed as parameters to other functions and be called when specific events or operations occur, providing a flexible callback mechanism.
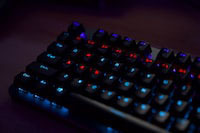
1. Introduction to Python Python is a general-purpose programming language that is easy to learn and powerful. It was created by Guido van Rossum in 1991. Python's design philosophy emphasizes code readability and provides developers with rich libraries and tools to help them build various applications quickly and efficiently. 2. Python basic syntax The basic syntax of Python is similar to other programming languages, including variables, data types, operators, control flow statements, etc. Variables are used to store data. Data types define the data types that variables can store. Operators are used to perform various operations on data. Control flow statements are used to control the execution flow of the program. 3.Python data types in Python
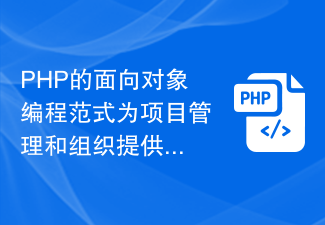
PHP's object-oriented programming paradigm provides advantages for project management and organization With the rapid development of the Internet, websites and applications of all sizes have sprung up. In order to meet the growing needs and improve development efficiency and maintainability, the use of object-oriented programming (Object-Oriented Programming, OOP for short) has become the mainstream of modern software development. In dynamic scripting languages like PHP, OOP brings many advantages to project management and organization. This article will introduce
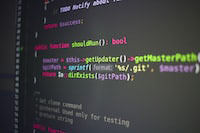
What is object-oriented programming? Object-oriented programming (OOP) is a programming paradigm that abstracts real-world entities into classes and uses objects to represent these entities. Classes define the properties and behavior of objects, and objects instantiate classes. The main advantage of OOP is that it makes code easier to understand, maintain and reuse. Basic Concepts of OOP The main concepts of OOP include classes, objects, properties and methods. A class is the blueprint of an object, which defines its properties and behavior. An object is an instance of a class and has all the properties and behaviors of the class. Properties are characteristics of an object that can store data. Methods are functions of an object that can operate on the object's data. Advantages of OOP The main advantages of OOP include: Reusability: OOP can make the code more
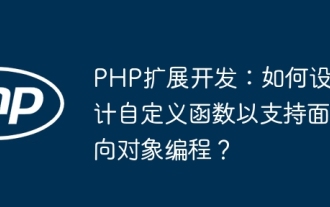
PHP extensions can support object-oriented programming by designing custom functions to create objects, access properties, and call methods. First create a custom function to instantiate the object, and then define functions that get properties and call methods. In actual combat, we can customize the function to create a MyClass object, obtain its my_property attribute, and call its my_method method.
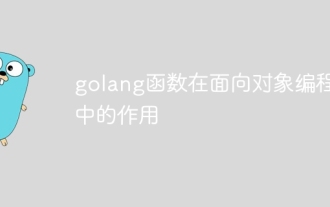
In the Go language, functions play a key role in object-oriented programming: Encapsulation: encapsulating behavior and operating objects. Operations: Perform operations on objects, such as modifying field values or performing tasks.
