


Study the underlying development principles of PHP: Detailed explanation of file processing and IO operation optimization techniques
Study on the underlying development principles of PHP: Detailed explanation of file processing and IO operation optimization techniques
Introduction:
PHP is a widely used server-side scripting language. The underlying development principles are critical to optimizing performance and improving user experience. This article will deeply study the file processing and IO operation optimization techniques in the underlying development principles of PHP, and explain it in detail with code examples.
1. File processing optimization skills
In PHP development, it is often necessary to read, write, modify and delete files. In order to improve the efficiency of file processing, we can adopt the following optimization techniques:
- Use multiple processes or multi-threads to process file IO operations
Single-threaded IO operations will cause performance bottlenecks. By using multiple processes Or multi-threading can realize parallel processing of multiple IO operations. The following is a sample code that uses multiple processes to handle file IO operations:
<?php $files = ['file1.txt', 'file2.txt', 'file3.txt']; $result = []; for ($i = 0; $i < count($files); $i++) { $pid = pcntl_fork(); // 创建子进程 if ($pid == -1) { die('Fork failed'); } elseif ($pid == 0) { // 子进程处理文件IO操作 $file = $files[$i]; // ... exit(); // 子进程退出 } else { // 父进程记录子进程ID $result[$i] = $pid; } } // 等待子进程退出 foreach ($result as $pid) { pcntl_waitpid($pid, $status); } ?>
- Reduce the number of file reads and writes as much as possible
Reducing the number of file reads and writes can reduce the overhead of IO operations. When reading a file, we can use the memory functions (file_get_contents, file_put_contents) provided by PHP to read or write the file at once. The following is a sample code that uses file_get_contents and file_put_contents to read and write files:
<?php // 一次性读取文件 $data = file_get_contents('file.txt'); // 一次性写入文件 file_put_contents('file.txt', $data); ?>
- Use efficient file opening methods
When opening a file, you can use flags related to file operations bit to specify the opening method. For example, use the "r" flag to open a file for reading only, and use the "w" flag to open a file for writing only. Reasonable selection of file opening methods can reduce the overhead of IO operations. The following is a sample code that uses flag bits to open a file:
<?php // 以只读方式打开文件 $handle = fopen('file.txt', 'r'); // ... fclose($handle); ?>
2. IO operation optimization skills
In addition to file processing, network IO operations are also an important part of PHP underlying development. In order to improve the performance of network IO operations, we can use the following optimization techniques:
- Use non-blocking IO operations
By default, PHP's network IO operations are blocking, that is, every IO The operation must wait for the result to be returned before continuing with the next instruction. In order to improve the concurrency and throughput of IO operations, non-blocking IO operations can be used. The following is a sample code using non-blocking IO operations:
<?php // 创建非阻塞socket $socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP); socket_set_nonblock($socket); // 连接服务器 socket_connect($socket, '127.0.0.1', 8080); // 等待连接完成 $read = [$socket]; $write = [$socket]; $except = []; socket_select($read, $write, $except, 5); // 发送数据 $buffer = 'Hello, World! '; socket_write($socket, $buffer, strlen($buffer)); // 接收数据 $result = ''; socket_recv($socket, $result, 1024, 0); // 关闭连接 socket_close($socket); ?>
- Set the IO operation timeout reasonably
Due to the uncertainty of the network environment, IO operations sometimes fail due to network abnormalities or The server is unresponsive and causes blocking. In order to avoid long-term blocking, you can set the timeout for IO operations. The following is a sample code that uses socket_set_option to set the IO operation timeout:
<?php // 创建socket $socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP); // 设置连接超时时间为2秒 socket_set_option($socket, SOL_SOCKET, SO_RCVTIMEO, ['sec'=>2, 'usec'=>0]); // 连接服务器 socket_connect($socket, '127.0.0.1', 8080); // 发送数据 $buffer = 'Hello, World! '; socket_write($socket, $buffer, strlen($buffer)); // 关闭连接 socket_close($socket); ?>
Conclusion:
This article mainly studies the file processing and IO operation optimization techniques in the underlying development principles of PHP, combined with the code Examples are explained in detail. In terms of file processing, it is recommended to use multiple processes or multi-threads to handle file IO operations, reduce the number of file reads and writes, and use appropriate file opening methods. In terms of IO operations, it is recommended to use non-blocking IO operations and set the IO operation timeout reasonably. These optimization techniques can significantly improve the performance and user experience of PHP, and are very helpful for underlying optimization in actual development.
The above is the detailed content of Study the underlying development principles of PHP: Detailed explanation of file processing and IO operation optimization techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


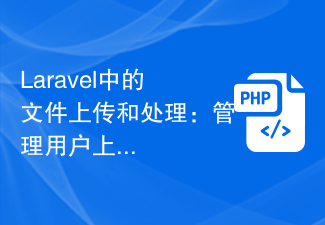
File Uploading and Processing in Laravel: Managing User Uploaded Files Introduction: File uploading is a very common functional requirement in modern web applications. In the Laravel framework, file uploading and processing becomes very simple and efficient. This article will introduce how to manage user-uploaded files in Laravel, including verification, storage, processing, and display of file uploads. 1. File upload File upload refers to uploading files from the client to the server. In Laravel, file uploads are very easy to handle. first,
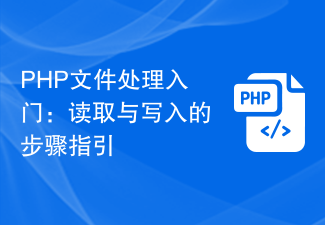
Getting started with PHP file processing: Step-by-step guide for reading and writing In web development, file processing is a common task, whether it is reading files uploaded by users or writing the results to files for subsequent use. Understand how to use PHP Document processing is very important. This article will provide a simple guide to introduce the basic steps of reading and writing files in PHP, and attach code examples for reference. File reading in PHP, you can use the fopen() function to open a file and return a file resource (file
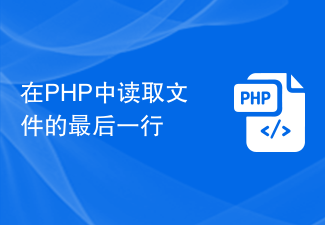
To read the last line of a file from PHP, the code is as follows -$line='';$f=fopen('data.txt','r');$cursor=-1;fseek($f,$cursor, SEEK_END);$char=fgetc($f);//Trimtrailingnewlinecharactersinthefilewhile($char===""||$char==="\r"){&
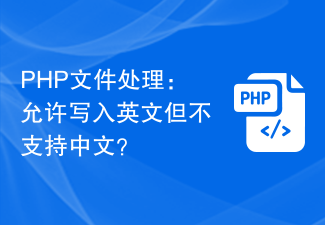
Title: PHP file processing: English writing is allowed but Chinese characters are not supported. When using PHP for file processing, sometimes we need to restrict the content in the file to only allow writing in English and not support Chinese characters. This requirement may be to maintain file encoding consistency, or to avoid garbled characters caused by Chinese characters. This article will introduce how to use PHP for file writing operations, ensure that only English content is allowed to be written, and provide specific code examples. First of all, we need to be clear that PHP itself does not actively limit
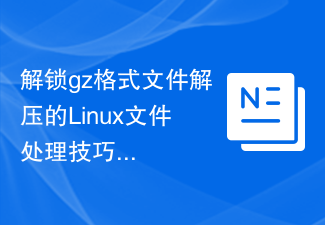
Linux file processing skills: Master the trick of decompressing gz format files. In Linux systems, you often encounter files compressed using gz (Gzip) format. This file format is very common in network transmission and file storage. If we want to process these .gz format files, we need to learn how to decompress them. This article will introduce several methods of decompressing .gz files and provide specific code examples to help readers master this technique. Method 1: Use the gzip command to decompress in Linux systems, the most common
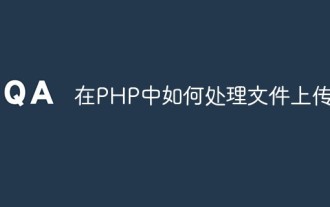
With the continuous development of Internet technology, the file upload function has become an essential part of many websites. In the PHP language, we can handle file uploads through some class libraries and functions. This article will focus on the file upload processing method in PHP. 1. Form settings In the HTML form, we need to set the enctype attribute to "multipart/form-data" to support file upload. The code is as follows: <formaction="upload.
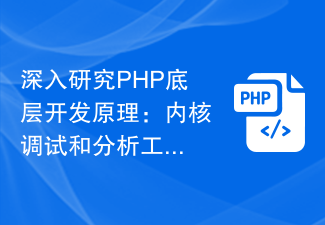
In-depth study of PHP's underlying development principles: Overview of kernel debugging and analysis tools As a programming language widely used in Web development, PHP's underlying development principles have always attracted the attention of developers. Understanding the underlying development principles of PHP is very important for improving code performance, troubleshooting problems, and expanding development. In this article, we will delve into the underlying development principles of PHP and introduce some practical kernel debugging and analysis tools to help readers better understand and apply PHP's underlying development. 1. PHP kernel debugging tool GDB
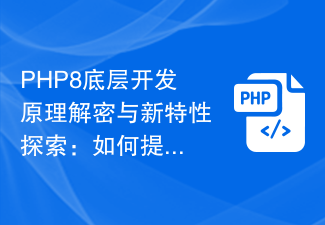
Decryption of the underlying development principles of PHP8 and exploration of new features: how to improve code quality. With the rapid development of Internet technology, PHP, as a very popular back-end development language, is widely used around the world. As the latest version of the PHP language, PHP8 brings many exciting new features and improved underlying development principles. These anticipated updates provide developers with more choices and opportunities to optimize code quality. This article will decrypt the underlying development principles of PHP8 and explore its new features to help developers improve their code
