


Utilize the underlying development principles of PHP8 to analyze new features: build excellent web applications
Use the underlying development principles of PHP8 to analyze new features: build outstanding web applications
With the release of PHP8, we have ushered in many powerful and exciting New features. These new features not only greatly improve the performance and efficiency of PHP, but also provide developers with more creativity and flexibility. In this article, we’ll take a deep dive into the underlying development principles of PHP8 and demonstrate these features by building a stunning web application.
1. JIT compiler
PHP8 introduces the JIT (Just In Time) compiler, which is an important new feature. The JIT compiler can compile PHP code directly into native machine code instead of executing it through an interpreter. This compilation method greatly improves the execution speed of PHP and significantly improves performance.
Let’s take a look at an example:
<?php $input = 100; $sum = 0; for ($i = 1; $i <= $input; $i++) { $sum += $i; } echo "Sum of numbers from 1 to $input is: $sum";
In PHP7 and previous versions, the execution time of the above code may be relatively long. However, in PHP8, due to the introduction of the JIT compiler, the execution time of the code is greatly shortened and the overall performance is improved.
2. New type system
PHP8 introduces a new type system, including class attribute type declaration, parameter type declaration and return type declaration. This makes the code clearer and more readable, and allows for type checking at compile time, reducing errors and debugging time.
Let us see an example:
<?php class User { public int $id; public string $name; } function getUserById(int $id): ?User { // 根据ID从数据库中查询用户数据 // ... if ($userExists) { $user = new User(); $user->id = $id; $user->name = $name; return $user; } return null; } $userId = 123; $user = getUserById($userId); if ($user) { echo "User found: {$user->name}"; } else { echo "User not found."; }
In the above code, we define a User class and use the type declaration and return type declaration in the getUserById function. The advantage of this is that we can find type errors earlier in the development process, improving code quality and efficiency.
3. New error handling mechanism
PHP8 has improved the error handling mechanism and introduced new Throwable interface, Error class and Exception class. With these improvements, we can better handle errors in our code, provide more detailed exception information, and better track exception handling.
The following is an example:
<?php try { // 尝试执行一个可能会出错的操作 // ... } catch (Throwable $e) { // 处理异常 echo "Exception: " . $e->getMessage(); }
In the above code, we use the try-catch statement to capture exceptions that may occur, and define the type of exception through the Throwable interface. In this way, we can perform different handling operations for different types of exceptions, making the code more robust and reliable.
4. Better performance and memory management
PHP8 further improves the execution efficiency of the code by improving the underlying memory management and garbage collection mechanism. The garbage collection mechanism in the new version can more accurately identify unused memory blocks and recycle them in a timely manner, effectively reducing memory usage and waste.
Summary:
The underlying development principles of PHP8 bring more creativity and flexibility to developers. With a JIT compiler, a new type system, error handling mechanisms, and performance optimizations, we can build great web applications. Let us fully explore the potential of PHP8 and provide users with a better user experience.
(over)
The above is the detailed content of Utilize the underlying development principles of PHP8 to analyze new features: build excellent web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


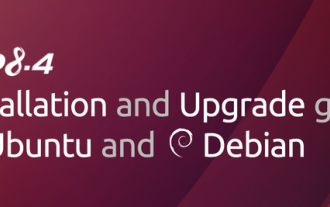
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
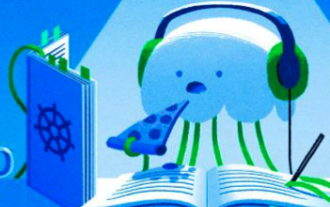
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
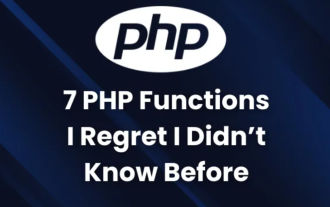
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
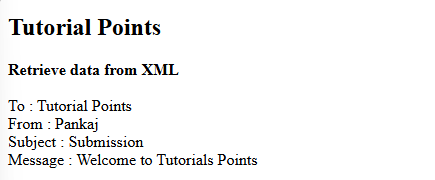
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
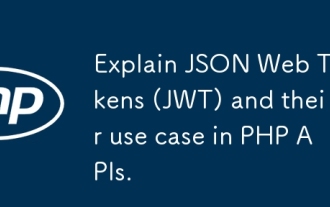
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
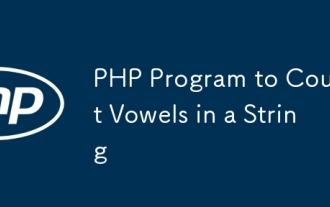
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
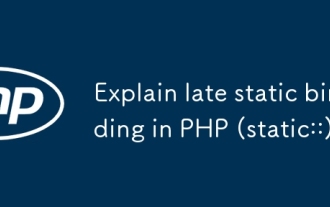
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
