


PHP from beginner to advanced -- learn basic syntax and concepts
PHP entry to advanced - learn basic syntax and concepts
Introduction:
PHP (Hypertext Preprocessor) is a popular server-side scripting language that is widely used Applied to web development. In this article, we will start from the entry level, gradually learn the basic syntax and concepts of PHP in depth, and provide code examples for your reference.
1. PHP installation and configuration
Before you start learning PHP, you first need to install PHP on your machine. You can download the latest version of PHP from the official website (https://www.php.net/downloads.php) and configure it according to the corresponding installation guide. After successfully completing the installation and configuration, you can verify that the installation was successful by entering the following command in the terminal: php -v.
2. The first PHP program
Let us start with a simple "Hello, World!" program:
<?php echo "Hello, World!"; ?>
In the above example, tag is interpreted as PHP code. Echo is used to output text to the browser. The effect is to display the text "Hello, World!" on the web page.
3. Variables and data types
In PHP, you do not need to explicitly declare the type of a variable. The following are some commonly used data types and their examples:
<?php $string = "Hello, World!"; // 字符串类型 $number = 1234; // 整数类型 $float = 3.14; // 浮点数类型 $boolean = true; // 布尔类型 $array = array("apple", "banana"); // 数组类型
You can use the var_dump() function to view the type and value of the variable:
<?php var_dump($string);
4. Operators
PHP provides A series of operators used to implement common arithmetic, logical and comparison operations. The following are some commonly used operator examples:
<?php $a = 10; $b = 5; $sum = $a + $b; // 加法运算 $diff = $a - $b; // 减法运算 $product = $a * $b; // 乘法运算 $quotient = $a / $b; // 除法运算 $modulus = $a % $b; // 取模运算 $is_equal = ($a == $b); // 等于运算 $is_not_equal = ($a != $b); // 不等于运算 $is_greater = ($a > $b); // 大于运算 $is_less = ($a < $b); // 小于运算
5. Conditional statements and loops
In PHP, conditional statements and loop structures are similar to other programming languages. Here are some examples:
<?php if ($a > $b) { echo "a is greater than b"; } elseif ($a < $b) { echo "a is less than b"; } else { echo "a is equal to b"; } for ($i = 1; $i <= 10; $i++) { echo $i; } while ($a < 10) { echo $a; $a++; }
6. Function
A function is a reusable code block that can help us organize the code and improve the readability and maintainability of the code. The following is an example:
<?php function add($num1, $num2) { return $num1 + $num2; } $result = add(5, 3); echo $result;
7. File operation
In PHP, you can read and write files using the file operation function. The following are some examples of commonly used file operation functions:
<?php $file = fopen("test.txt", "w"); fwrite($file, "Hello, World!"); fclose($file); $file = fopen("test.txt", "r"); echo fgets($file); fclose($file);
8. Classes and Objects
PHP is an object-oriented programming (Object-Oriented Programming, abbreviated as OOP) language that supports the concepts of classes and objects . The following is an example of classes and objects:
<?php class Animal { public $name; public function greet() { echo "Hello, my name is " . $this->name; } } $cat = new Animal(); $cat->name = "Tom"; $cat->greet();
Conclusion:
In this article, we started from the installation and configuration of PHP, gradually learned the basic syntax and concepts of PHP in depth, and provided a large number of Code examples are provided for your reference. I hope that by studying this article, you can have a deeper understanding of PHP and be able to use it flexibly in actual development. I wish you success in your studies and develop high-quality PHP applications!
The above is the detailed content of PHP from beginner to advanced -- learn basic syntax and concepts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
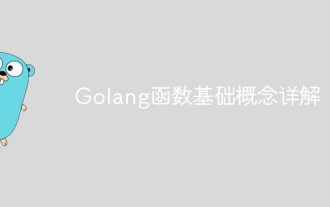
With the rapid development of modern programming languages, Golang has become the language of choice for more and more people. As one of the basic concepts in the Golang language, functions provide powerful tool support for programmers. This article will explain in detail the definition, parameters, return values, scope and other basic concepts of Golang functions, as well as some advanced application scenarios and techniques, to help readers better understand and use Golang functions. 1. Definition of function A function is a basic concept in Golang. It is a paragraph that can be repeated
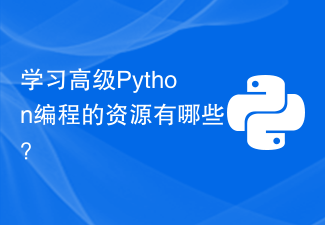
The demand for Python as a programming language has driven its rich resources for learning its different aspects. While beginners have a variety of tutorials and guides to help them get started, advanced learners often struggle to find resources that meet their specific needs. In this article, we'll explore a series of resources designed to improve your Python skills, covering topics such as advanced language features, design patterns, performance optimization, and more. Advanced Python language featuresTogetthemostoutofPython,it’simportanttomasteritsadvancedlanguagefeatures.Thesefeaturesenableefficient,rea
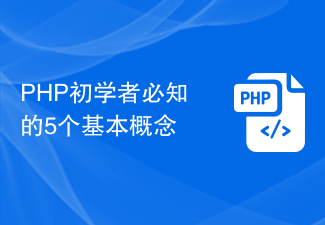
With the continuous development of Internet technology, PHP, as a Web programming language, is becoming more and more popular. PHP is widely used and can be developed using PHP from simple static websites to large e-commerce websites. Whether you are a novice who is just starting to learn PHP or a developer who already has some experience, it is very necessary to master some basic concepts. In this article, I will introduce 5 basic concepts that PHP beginners must know. Variables in PHP are containers used to store data. In PHP,
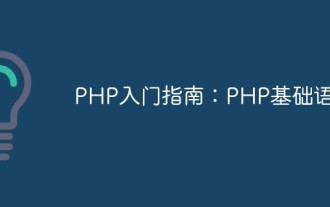
PHP is a server-side scripting language that is used to develop dynamic websites, web applications, and web programs. PHP has a wide range of applications, and both beginners and experienced developers can benefit from it. This article will provide you with an introductory guide to the basic syntax of PHP. If you want to learn PHP programming and build a solid foundation from scratch, you've come to the right place. The basic structure of PHP. A PHP program contains the following three parts: <?php//PHP code?>& on both sides of the code
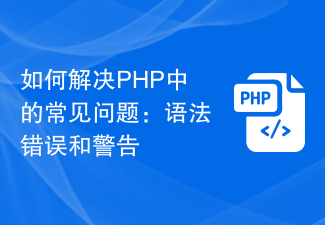
PHP is a widely used server-side programming language often used to build dynamic web pages. However, when writing PHP scripts, you often encounter various syntax errors and warnings. These errors and warnings can cause your code to not run properly or cause problems, so it's important to resolve them. This article will introduce common problems in PHP: syntax errors and warnings, and provide effective methods on how to solve these problems. Syntax Errors Syntax errors are a very common problem when writing scripts in PHP. Syntax errors may be due to the following reasons
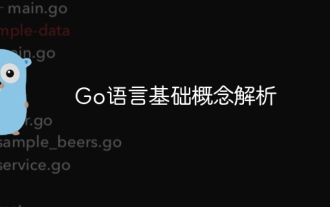
Go language is a high-performance, concurrent programming language. Its basic concepts include: basic types (integers, floating point numbers, Boolean values, strings, characters); declaring variables through the var keyword and supporting type inference; using the const keyword Declare constants; provide standard control flow statements; declare functions through the func keyword; introduce packages through the import statement. Practical cases demonstrate the generation of Fibonacci sequences and deepen the understanding of these concepts.
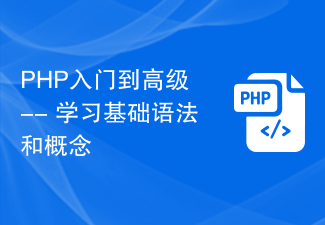
PHP entry to advanced - learn basic syntax and concepts Introduction: PHP (Hypertext Preprocessor) is a popular server-side scripting language widely used in Web development. In this article, we will start from the entry level, gradually learn the basic syntax and concepts of PHP in depth, and provide code examples for your reference. 1. PHP installation and configuration Before starting to learn PHP, you first need to install PHP on your machine. You can download it from the official website (https://w
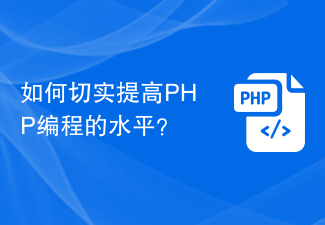
PHP is a language widely used in web development, and its popularity and usage rate are quite high. Many beginners will encounter some difficulties when learning PHP programming, such as not knowing how to improve their programming level. Below we will introduce some methods to make it easier for you to improve your PHP programming level. Learn the latest technology Internet technology updates very quickly, and PHP is no exception. If you want to become an excellent PHP programmer, you must first learn the latest PHP technology and master the latest Web development technology, such as M
