


Detailed analysis of the underlying development principles of PHP7: the process from syntax analysis to semantic analysis
Detailed analysis of the underlying development principles of PHP7: the process from syntax analysis to semantic analysis
The underlying development principles of programming languages are basic knowledge that programmers must master. In the underlying development of PHP7, syntax parsing and semantic analysis are two very important processes. This article will analyze these two processes in detail and attach corresponding code examples.
1. Grammar parsing
Grammar parsing is the process of converting PHP code into a syntax tree. Its main task is to convert codes in the form of strings into structured data that can be understood by computers. In PHP7, syntax parsing is implemented using generators and parsers.
- Generator
A generator is a function that can use the yield statement to return a value and retain the current state of the function. Generators are a flexible and efficient implementation that save memory and CPU usage. The following is a simple generator example:
function myGenerator() { yield 'Hello'; yield 'World'; } $gen = myGenerator(); foreach ($gen as $value) { echo $value . ' '; } // 输出结果:Hello World
In the above code, the myGenerator() function is a generator function that uses the yield statement to return two values respectively. In a foreach loop, the generator function is executed and a value is returned each time through the loop.
- Parser
The parser is the key component that converts PHP code into a syntax tree. In PHP7, the parser is implemented using lexical analysis and syntax analysis.
- Lexical analysis: The lexical analyzer parses the source code into individual tags, such as variable names, keywords, operators, etc. The following is a simple lexical analysis example:
$code = '<?php $a = 1 + 2; ?>'; $tokens = token_get_all($code); foreach($tokens as $token) { if (is_array($token)) { echo "Line {$token[2]}: {$token[1]}".PHP_EOL; } } // 输出结果: // Line 1: <?php // Line 1: $ // ...
In the above code, the token_get_all() function parses the source code into tokens and stores them in the tokens array. By looping through the tokens array, we can output the content and line number of each token.
- Grammar analysis: The grammar analyzer converts the tokens obtained by lexical analysis into a syntax tree. The following is a simple syntax analysis example:
$code = '<?php $a = 1 + 2; ?>'; $tokens = token_get_all($code); $parser = new Parser(); $ast = $parser->parse($tokens); print_r($ast); // 输出结果: // Array // ( // [0] => Array // ( // [name] => AST_ASSIGN // [left] => Array // ( // [name] => AST_VAR // [value] => $a // ...
In the above code, the token_get_all() function parses the source code into tokens and stores them in the tokens array. We then use the parse() method of the Parser class to convert the token into a syntax tree, which will be returned as an array.
2. Semantic analysis
Semantic analysis is the process of analyzing and processing syntax trees. It is mainly used to check code consistency and logical errors, and perform type inference. In PHP7, semantic analysis is implemented using symbol tables and type systems.
- Symbol table
The symbol table is a data structure used to store information about variables and functions in the code. In semantic analysis, the symbol table plays an important role and can check problems such as variable redefinition and variable type errors. The following is a simple symbol table example:
$symbol_table = array(); function addVariable($name, $type) { global $symbol_table; if (isset($symbol_table[$name])) { throw new Exception("Variable {$name} is already defined"); } $symbol_table[$name] = $type; } function getVariableType($name) { global $symbol_table; if (!isset($symbol_table[$name])) { throw new Exception("Variable {$name} is not defined"); } return $symbol_table[$name]; } addVariable('a', 'int'); addVariable('b', 'string'); echo getVariableType('a'); // 输出结果:int echo getVariableType('b'); // 输出结果:string
In the above code, we use a global variable $symbol_table to store variable information. The addVariable() function is used to add variables to the symbol table, and the getVariableType() function is used to obtain the type of the variable. If the variable is already defined, an exception is thrown.
- Type system
The type system is a mechanism for type checking variables and expressions. In semantic analysis, the type system can check for problems such as type mismatches and type conversion errors. The following is a simple type system example:
function add($a, $b) { return $a + $b; } echo add(1, 2); // 输出结果:3 echo add("Hello", "World"); // 输出结果:HelloWorld echo add(1, "World"); // 抛出异常:Invalid operand types
In the above code, the add() function is used to add two operands. When the operand type is an integer, the add() function performs an addition operation; when the operand type is a string, the add() function performs a string concatenation operation. If the operand types do not match, an exception is thrown.
Summary:
The underlying development principles of PHP7 involve two core processes: syntax parsing and semantic analysis. Syntax parsing is the process of converting PHP code into a syntax tree, which is implemented using generators and parsers. Semantic analysis is the process of analyzing and processing syntax trees, which is implemented using symbol tables and type systems. A deep understanding of these two processes is very important for underlying development and code optimization.
Through the detailed analysis and code examples of this article, I believe that readers will have a deeper understanding of the underlying development principles of PHP7 and be able to apply them to actual development. I hope this article can help you, and I wish you go further and further on the road of underlying development of PHP7!
The above is the detailed content of Detailed analysis of the underlying development principles of PHP7: the process from syntax analysis to semantic analysis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


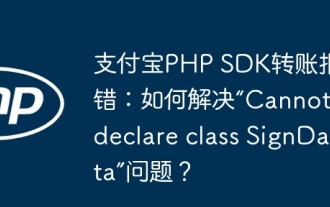
Alipay PHP...
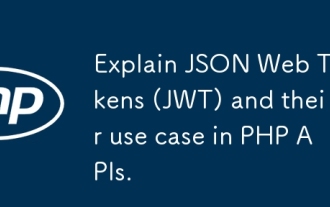
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
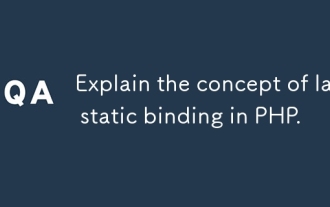
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
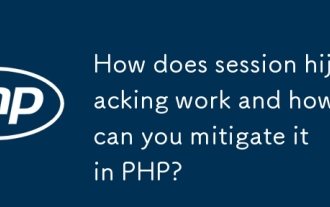
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
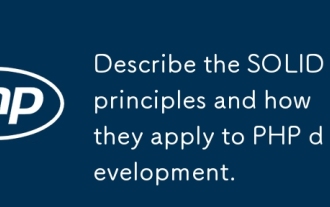
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
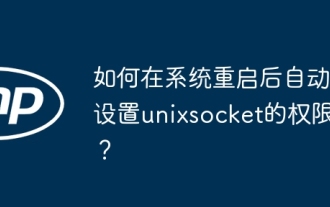
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
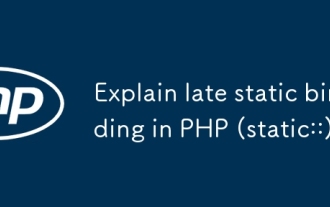
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
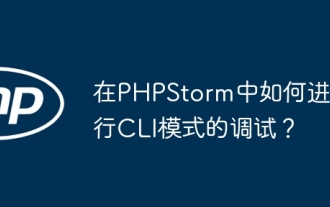
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
