PHP实现的Mysql读写分离
主要特性:
简单的读写分离
一个主数据库,可以添加更多的只读数据库
读写分离但不用担心某些特性不支持
缺点:同时连接两个数据库
英文比较烂,也写几个字吧
php code for mysql read/write split feature: simply rw split one master,can add more slaves support all mysql feature link to the master and slave at the same time
mysql_rw_php.class.php
<?php /**************************************** *** mysql-rw-php version 0.1 @ 2009-4-16 *** code by hqlulu#gmail.com *** http://www.aslibra.com *** http://code.google.com/p/mysql-rw-php/ *** code modify from class_mysql.php (uchome) ****************************************/ class mysql_rw_php { //查询个数 var $querynum = 0; //当前操作的数据库连接 var $link = null; //字符集 var $charset; //当前数据库 var $cur_db = ''; //是否存在有效的只读数据库连接 var $ro_exist = false; //只读数据库连接 var $link_ro = null; //读写数据库连接 var $link_rw = null; function mysql_rw_php(){ } function connect($dbhost, $dbuser, $dbpw, $dbname = '', $pconnect = 0, $halt = TRUE) { if($pconnect) { if(!$this->link = @mysql_pconnect($dbhost, $dbuser, $dbpw)) { $halt && $this->halt('Can not connect to MySQL server'); } } else { if(!$this->link = @mysql_connect($dbhost, $dbuser, $dbpw)) { $halt && $this->halt('Can not connect to MySQL server'); } } //只读连接失败 if(!$this->link && !$halt) return false; //未初始化rw时,第一个连接作为rw if($this->link_rw == null) $this->link_rw = $this->link; if($this->version() > '4.1') { if($this->charset) { @mysql_query("SET character_set_connection=$this->charset, character_set_results=$this->charset, character_set_client=binary", $this->link); } if($this->version() > '5.0.1') { @mysql_query("SET sql_mode=''", $this->link); } } if($dbname) { $this->select_db($dbname); } } //连接一个只读的mysql数据库 function connect_ro($dbhost, $dbuser, $dbpw, $dbname = '', $pconnect = 0){ if($this->link_rw == null) $this->link_rw = $this->link; $this->link = null; //不产生halt错误 $this->connect($dbhost, $dbuser, $dbpw, $dbname, $pconnect, false); if($this->link){ //连接成功 //echo "link ro sussess!<br>"; $this->ro_exist = true; $this->link_ro = $this->link; if($this->cur_db){ //如果已经选择过数据库则需要操作一次 @mysql_select_db($this->cur_db, $this->link_ro); } }else{ //连接失败 //echo "link ro failed!<br>"; $this->link = &$this->link_rw; } } //设置一系列只读数据库并且连接其中一个 function set_ro_list($ro_list){ if(is_array($ro_list)){ //随机选择其中一个 $link_ro = $ro_list[array_rand($ro_list)]; $this->connect_ro($link_ro['dbhost'], $link_ro['dbuser'], $link_ro['dbpw']); } } function select_db($dbname) { //同时操作两个数据库连接 $this->cur_db = $dbname; if($this->ro_exist){ @mysql_select_db($dbname, $this->link_ro); } return @mysql_select_db($dbname, $this->link_rw); } function fetch_array($query, $result_type = MYSQL_ASSOC) { return mysql_fetch_array($query, $result_type); } function fetch_one_array($sql, $type = '') { $qr = $this->query($sql, $type); return $this->fetch_array($qr); } function query($sql, $type = '') { $this->link = &$this->link_rw; //判断是否select语句 if($this->ro_exist && preg_match ("/^(\s*)select/i", $sql)){ $this->link = &$this->link_ro; } $func = $type == 'UNBUFFERED' && @function_exists('mysql_unbuffered_query') ? 'mysql_unbuffered_query' : 'mysql_query'; if(!($query = $func($sql, $this->link)) && $type != 'SILENT') { $this->halt('MySQL Query Error', $sql); } $this->querynum++; return $query; } function affected_rows() { return mysql_affected_rows($this->link); } function error() { return (($this->link) ? mysql_error($this->link) : mysql_error()); } function errno() { return intval(($this->link) ? mysql_errno($this->link) : mysql_errno()); } function result($query, $row) { $query = @mysql_result($query, $row); return $query; } function num_rows($query) { $query = mysql_num_rows($query); return $query; } function num_fields($query) { return mysql_num_fields($query); } function free_result($query) { return mysql_free_result($query); } function insert_id() { return ($id = mysql_insert_id($this->link)) >= 0 ? $id : $this->result($this->query("SELECT last_insert_id()"), 0); } function fetch_row($query) { $query = mysql_fetch_row($query); return $query; } function fetch_fields($query) { return mysql_fetch_field($query); } function version() { return mysql_get_server_info($this->link); } function close() { return mysql_close($this->link); } function halt($message = '', $sql = '') { $dberror = $this->error(); $dberrno = $this->errno(); echo "<div style=\"position:absolute;font-size:11px;font-family:verdana,arial;background:#EBEBEB; padding:0.5em;\"> <b>MySQL Error</b><br> <b>Message</b>: $message<br> <b>SQL</b>: $sql<br> <b>Error</b>: $dberror<br> <b>Errno.</b>: $dberrno<br> </div>"; exit(); } } ?>
example.php
<?php /**************************************** *** mysql-rw-php version 0.1 @ 2009-4-16 *** code by hqlulu#gmail.com *** http://www.aslibra.com *** http://code.google.com/p/mysql-rw-php/ *** code modify from class_mysql.php (uchome) ****************************************/ require_once('mysql_rw_php.class.php'); //rw info $db_rw = array( 'dbhost'=>'www.aslibra.com', 'dbuser'=>'aslibra', 'dbpw'=>'www.aslibra.com', 'dbname'=>'test' ); $db_ro = array( array( 'dbhost'=>'www.aslibra.com:4306', 'dbuser'=>'aslibra', 'dbpw'=>'www.aslibra.com' ) ); $DB = new mysql_rw_php; //connect Master $DB->connect($db_rw[dbhost], $db_rw[dbuser], $db_rw[dbpw], $db_rw[dbname]); //Method 1: connect one server $DB->connect_ro($db_ro[0][dbhost], $db_ro[0][dbuser], $db_ro[0][dbpw]); //Method 2: connect one server from a list by rand $DB->set_ro_list($db_ro); //send to rw $sql = "insert into a set a='test'"; $DB->query($sql); //send to ro $sql = "select * from a"; $qr = $DB->query($sql); while($row = $DB->fetch_array($qr)){ echo $row[a]; } ?>
以上就是PHP实现的Mysql读写分离的内容,更多相关内容请关注PHP中文网(www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
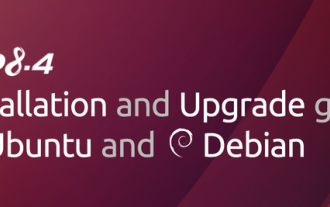
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati

One of the major changes introduced in MySQL 8.4 (the latest LTS release as of 2024) is that the "MySQL Native Password" plugin is no longer enabled by default. Further, MySQL 9.0 removes this plugin completely. This change affects PHP and other app
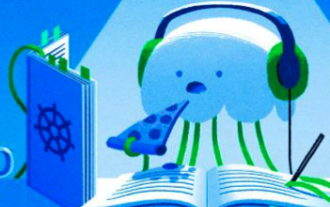
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
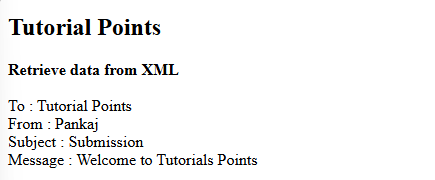
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
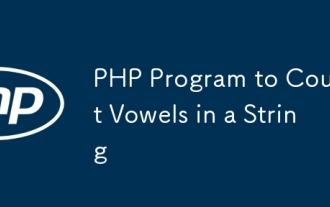
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
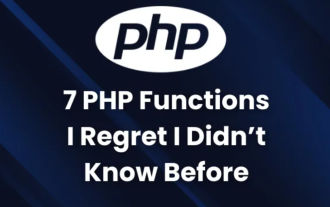
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
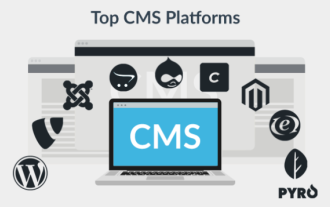
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
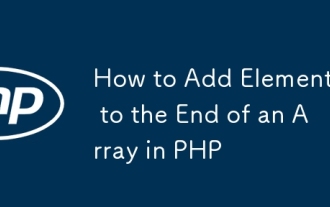
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
