


Best practices for implementing HTML/XML parsing and processing in PHP
Best Practices for Implementing HTML/XML Parsing and Processing in PHP
Overview:
In web development, it is often necessary to process and parse HTML or XML document. As a popular server-side scripting language, PHP provides a wealth of tools and function libraries that can easily implement HTML/XML parsing and processing. This article will introduce the best practices for HTML/XML parsing and processing in PHP and provide some code examples.
1. Use built-in functions for HTML parsing
PHP provides multiple built-in functions for HTML parsing, the most commonly used of which are:
- file_get_contents: used for reading HTML file content.
- strip_tags: used to remove HTML tags.
- htmlspecialchars: used to convert special characters into HTML entities.
Code example 1: Use file_get_contents to read HTML file content
$html = file_get_contents('example.html'); echo $html;
Code example 2: Use strip_tags to remove HTML tags
$html = '<h1 id="Hello-World">Hello, World!</h1><p>This is an example.</p>'; $plainText = strip_tags($html); echo $plainText;
Code example 3: Use htmlspecialchars to convert Special characters
$text = 'This is some <b>bold</b> text.'; $encodedText = htmlspecialchars($text); echo $encodedText;
2. Use extension libraries for advanced HTML/XML parsing
In addition to built-in functions, PHP also provides multiple extension libraries for advanced HTML/XML parsing and processing. The most commonly used ones are:
- DOMDocument: used to create, modify and query HTML/XML documents.
- SimpleXML: Used to parse and process simple XML documents.
Code example 4: Use DOMDocument to query HTML elements
$html = '<h1 id="Hello-World">Hello, World!</h1><p>This is an example.</p>'; $dom = new DOMDocument; $dom->loadHTML($html); $element = $dom->getElementsByTagName('h1')->item(0); echo $element->nodeValue;
Code example 5: Use SimpleXML to parse XML documents
$xml = <<<XML <root> <name>John Doe</name> <age>30</age> </root> XML; $simplexml = simplexml_load_string($xml); $name = $simplexml->name; $age = $simplexml->age; echo $name, ' is ', $age, ' years old.';
3. Processing special features in HTML/XML Situation
In actual HTML/XML parsing processing, some special situations may be encountered, requiring additional processing and conversion.
- Processing namespaces
If you want to process an XML document containing a namespace, you need to use the corresponding function or method to process the namespace.
Code example 6: Processing namespace
$xml = <<<XML <root xmlns:ns="http://example.com"> <ns:name>John Doe</ns:name> <ns:age>30</ns:age> </root> XML; $simplexml = simplexml_load_string($xml); $simplexml->registerXPathNamespace('ns', 'http://example.com'); $names = $simplexml->xpath('//ns:name'); foreach ($names as $name) { echo $name; }
- Processing attributes
If you want to process the attributes of HTML/XML tags, you need to use the corresponding methods to obtain and modify them Attributes.
Code example 7: Processing HTML tag attributes
$html = '<a href="http://example.com">Link</a>'; $dom = new DOMDocument; $dom->loadHTML($html); $element = $dom->getElementsByTagName('a')->item(0); $href = $element->getAttribute('href'); echo $href;
Conclusion:
Through PHP's built-in functions and extension libraries, we can easily implement HTML/XML parsing and processing. In actual applications, appropriate methods and functions are selected for processing according to specific needs and scenarios. By mastering the best practices for HTML/XML parsing and processing, you can improve development efficiency and achieve more flexible and reliable web applications.
The above is the detailed content of Best practices for implementing HTML/XML parsing and processing in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
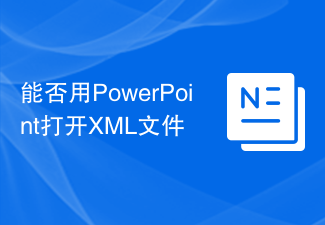
Can XML files be opened with PPT? XML, Extensible Markup Language (Extensible Markup Language), is a universal markup language that is widely used in data exchange and data storage. Compared with HTML, XML is more flexible and can define its own tags and data structures, making the storage and exchange of data more convenient and unified. PPT, or PowerPoint, is a software developed by Microsoft for creating presentations. It provides a comprehensive way of
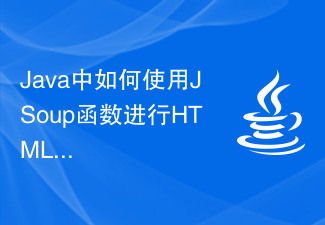
HTML is the basic representation of web pages. If you want to obtain and manipulate the content of an HTML document in Java, you need to use an open source parsing tool, such as the JSoup function. JSoup is a Java library for processing HTML documents. It provides a very simple way to extract specific data and elements from HTML documents. This article will introduce the use of JSoup in Java. Importing JSoup First, you need to import the JSoup library in your Java project. You can use Mave
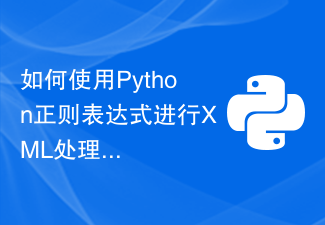
In daily data processing scenarios, data processing in different formats requires different parsing methods. For data in XML format, we can use regular expressions in Python for parsing. This article will introduce the basic ideas and methods of using Python regular expressions for XML processing. Introduction to XML Basics XML (Extensible Markup Language) is a markup language used to describe data. It provides a structured method to represent data. An important feature of XML
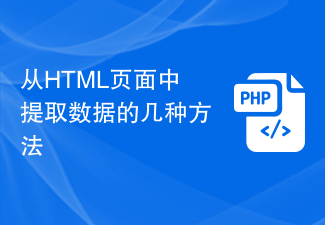
HTML page is the most common type of Internet page. It is written in the form of markup language and includes many tags and elements. In many cases, we need to extract data from HTML pages so that the pages can be correctly analyzed, managed, and processed. This article will introduce some methods to extract data from HTML pages to help readers complete this task easily. 1. Use regular expressions. Regular expressions are a commonly used tool in text processing and one of the most basic methods for extracting data from HTML pages.
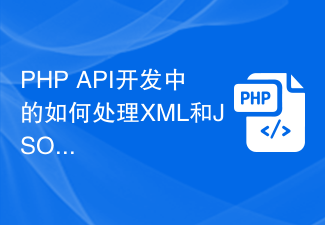
In modern software development, many applications need to interact through APIs (Application Programming Interfaces), allowing data sharing and communication between different applications. In PHP development, APIs are a common technology that allow PHP developers to integrate with other systems and work with different data formats. In this article, we will explore how to handle XML and JSON format data in PHPAPI development. XML format data processing XML (Extensible Markup Language) is a commonly used data format used in various
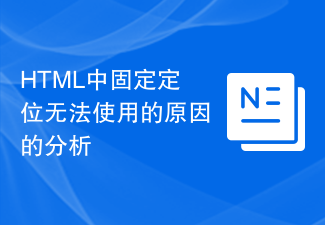
HTML is a markup language used to build web pages. It provides rich tags and attributes to achieve various web page layout effects. Among them, fixed positioning is a commonly used layout method, which allows elements to be displayed at a fixed position relative to the browser window or parent element, and is not affected by scrolling. However, not all HTML elements support fixed positioning. This article will analyze the reasons why fixed positioning is not supported in HTML and provide specific code examples. First, we need to understand the syntax of fixed positioning. In HTML, use CSS styles
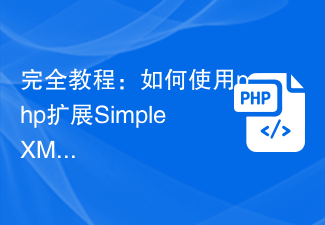
Complete Tutorial: How to Use PHP Extension SimpleXML to Process XML Data Introduction: In web development, processing XML data is a common task. PHP provides many built-in XML processing tools, the most commonly used of which is the SimpleXML extension. SimpleXML provides a simple and intuitive way to parse and manipulate XML data. This tutorial will introduce how to use the SimpleXML extension to process XML data, including parsing XML, accessing and modifying nodes, and converting XML

In-depth understanding of XML processing techniques in Java development In modern software development, XML (Extensible Markup Language) has become a very common data exchange and configuration file format. As a widely used programming language, Java provides rich APIs and tools to process XML files. In this article, we will delve into XML processing techniques in Java development to help developers better understand and apply XML. 1. Basic concepts of XML XML is a markup language used to describe data. It uses markup
