Written in C++, find a pair of numbers in a matrix with a given sum
In this article, we will discuss the program to find pairs with a given sum in a given matrix. For example -
Input : matrix[n][m] = { { 4, 6, 4, 65 }, { 56, 1, 12, 32 }, { 4, 5, 6, 44 }, { 13, 9, 11, 25 } }, SUM = 20 Output : Pair exists. Explanation : Sum = 20 is equal to the sum of numbers 9 and 11 which exists in the matrix. Input : matrix[n][m] = { { 5, 7, 3, 45 }, { 63, 5, 3, 7 }, { 11, 6, 9, 5 }, { 8, 6, 14, 15 } }, SUM = 13 Output : Pair does not exist. Explanation : No pair exists in the matrix whose sum is equal to 7.
Methods to find the solution
Now we will explain two different methods to find the solution to the above problem.
Brute force method
Consider each pair in the given matrix, check if the sum of the pair is equal to the given SUM, if so, print "Pair isn't"; otherwise, Prints "Pairing does not exist". Applying this method is very simple, but it increases the time complexity to O((N*M)2).
Efficient method
This program can store all matrix elements by using hash, then traverse the matrix and check whether the differences of [SUM & (index element)] are equal. If so, print "Exist" and exit the program. If it is NO, "does not exist" after traversing print.
Example
#include <bits/stdc++.h> using namespace std; #define n 4 #define m 4 int main() { int matrix[n][m] = { { 5,7, 3,45 }, { 63, 5, 3, 7 }, { 11, 6, 9, 5 }, { 8, 6, 14, 15 } }; int sum = 7; unordered_set<int> hash; for (int i = 0; i < n; i++) { for (int j = 0; j < m; j++) { if (hash.find(sum - matrix[i][j]) != hash.end()) { cout << "Pair exists." << endl; return 0; } else { hash.insert(matrix[i][j]); } } } cout << "Pair does not exist." << endl; return 0; }
Output
Pair does not exist.
The above code description
- Declares a two-dimensional array and stores elements in it.
- Traverse the array to find if (sum - Matrix[i][j]) != hash.end().
- If the condition is met, print "Pair contains" and return from the main function.
- Otherwise, continue traversing the array and finally print "Pair does notify.".
Conclusion
In this article, we discussed finding pairs or 2D arrays with a given sum in a matrix; we discussed both brute force and efficient ways to solve this problem . We discussed a C program to solve this problem. However, we can write this program in any other language like C, Java, Python, etc. We hope this article was helpful to you.
The above is the detailed content of Written in C++, find a pair of numbers in a matrix with a given sum. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


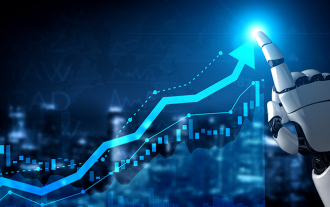
In the first article of this series, we discussed the connections and differences between artificial intelligence, machine learning, deep learning, data science, and more. We also made some hard choices about the programming languages, tools, and more that the entire series would use. Finally, we also introduced a little bit of matrix knowledge. In this article, we will discuss in depth the matrix, the core of artificial intelligence. But before that, let’s first understand the history of artificial intelligence. Why do we need to understand the history of artificial intelligence? There have been many AI booms in history, but in many cases the huge expectations for AI's potential failed to materialize. Understanding the history of artificial intelligence can help us see whether this wave of artificial intelligence will create miracles or is just another bubble about to burst. us
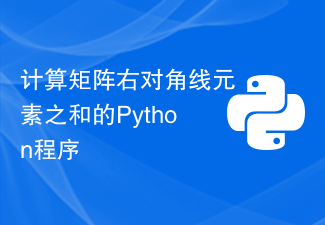
A popular general-purpose programming language is Python. It is used in a variety of industries, including desktop applications, web development, and machine learning. Fortunately, Python has a simple and easy-to-understand syntax that is suitable for beginners. In this article, we will use Python to calculate the sum of the right diagonal of a matrix. What is a matrix? In mathematics, we use a rectangular array or matrix to describe a mathematical object or its properties. It is a rectangular array or table containing numbers, symbols, or expressions arranged in rows and columns. . For example -234512367574 Therefore, this is a matrix with 3 rows and 4 columns, expressed as a 3*4 matrix. Now, there are two diagonals in the matrix, the primary diagonal and the secondary diagonal
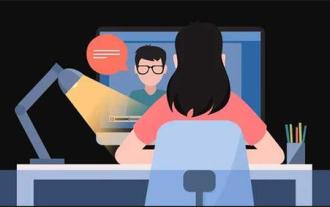
Which one is better, Celeron g4900 or i36100? When it comes to the two processors Celeron G4900 and I36100, there is no doubt that the performance of I36100 is superior. Celeron processors are generally considered low-end processors and are primarily used in budget laptops. The I3 processor is mainly used for high-end processors, and its performance is very good. Whether you are playing games or watching videos, you will not experience any lagging when using the I3 processor. Therefore, if possible, try to buy Intel I-series processors, especially for desktop computers, so that you can enjoy the fun of the online world. How is the performance of the Celeron G4900T? From a performance perspective, the Pentium G4900T performs well in terms of frequency. Compared with the previous version, the CPU performance is
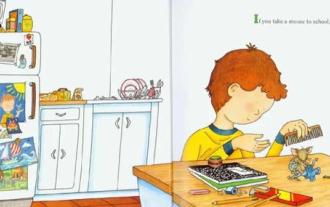
Which ASUS motherboard should be paired with the R55600? The ASUS ROGStrixB550-FGaming motherboard is an excellent choice. It is perfectly compatible with Ryzen55600X processor and provides excellent performance and features. This motherboard has a reliable power supply system, can support overclocking, and provides a wealth of expansion slots and ports to meet daily use and gaming needs. ROGStrixB550-FGaming is also equipped with high-quality audio solutions, fast network connections and reliable heat dissipation design to ensure that the system remains efficient and stable. In addition, this motherboard adopts a gorgeous ROG style and is equipped with gorgeous RGB lighting effects, adding visual enjoyment to your computer. All in all, ASUS ROGStri
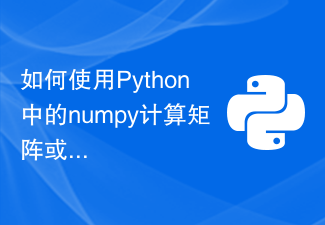
In this article, we will learn how to calculate the determinant of a matrix using the numpy library in Python. The determinant of a matrix is a scalar value that can represent the matrix in compact form. It is a useful quantity in linear algebra and has numerous applications in various fields including physics, engineering, and computer science. In this article, we will first discuss the definition and properties of determinants. We will then learn how to use numpy to calculate the determinant of a matrix and see how it is used in practice through some examples. Thedeterminantofamatrixisascalarvaluethatcanbeusedtodescribethepropertie
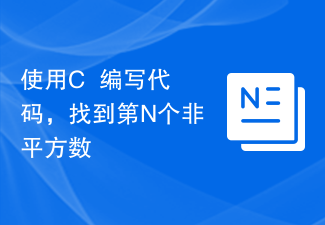
We all know numbers that are not the square of any number, such as 2, 3, 5, 7, 8, etc. There are N non-square numbers, and it is impossible to know every number. So, in this article, we will explain everything about squareless or non-square numbers and ways to find the Nth non-square number in C++. Nth non-square number If a number is the square of an integer, then the number is called a perfect square. Some examples of perfect square numbers are -1issquareof14issquareof29issquareof316issquareof425issquareof5 If a number is not the square of any integer, then the number is called non-square. For example, the first 15 non-square numbers are -2,3,5,6,
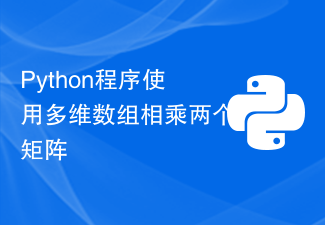
A matrix is a set of numbers arranged in rows and columns. A matrix with m rows and n columns is called an mXn matrix, and m and n are called its dimensions. A matrix is a two-dimensional array created in Python using lists or NumPy arrays. In general, matrix multiplication can be done by multiplying the rows of the first matrix by the columns of the second matrix. Here, the number of columns of the first matrix should be equal to the number of rows of the second matrix. Input and output scenario Suppose we have two matrices A and B. The dimensions of these two matrices are 2X3 and 3X2 respectively. The resulting matrix after multiplication will have 2 rows and 1 column. [b1,b2][a1,a2,a3]*[b3,b4]=[a1*b1+a2*b2+a3*a3][a4,a5,a6][b5,b6][a4*b2+a
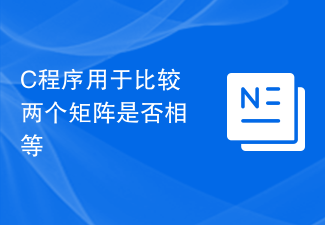
The user must enter the order of the two matrices as well as the elements of both matrices. Then, compare the two matrices. Two matrices are equal if both matrix elements and sizes are equal. If the matrices are equal in size but not equal in elements, then the matrices are shown to be comparable but not equal. If the sizes and elements do not match, the display matrices cannot be compared. The following program is a C program, used to compare whether two matrices are equal-#include<stdio.h>#include<conio.h>main(){ intA[10][10],B[10][10]; in
