


Use Workerman to realize user authentication and authority control of online chat system
Use workerman to implement user authentication and permission control of online chat systems
As a high-performance PHP socket framework, workerman is widely used in the development of real-time communication systems. When developing an online chat system, user authentication and permission control are very important links. This article will introduce how to use Workerman to complete user authentication and permission control, and attach code examples.
- User Authentication
User authentication refers to verifying whether the user's identity is legal. In online chat systems, the Token-based authentication mechanism is usually used. The specific steps are as follows:
Step 1: When the user logs in, the server generates a Token and sends the Token to the client for storage.
Step 2: The client sends the Token to the server in the form of HTTP Header in subsequent requests.
Step 3: When the server receives the request, verify the validity of the Token. If the token is valid, the user is considered logged in and the request can continue to be processed; otherwise, an authentication failure error message is returned.
The following is a sample code that uses workererman to implement user authentication:
require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; use WorkermanConnectionAsyncTcpConnection; use WorkermanProtocolsHttpRequest; use WorkermanProtocolsHttpResponse; $worker = new Worker('http://0.0.0.0:8080'); $users = [ 'user1' => 'password1', 'user2' => 'password2', // ... ]; $worker->onMessage = function ($connection, Request $request) use ($users) { $path = $request->path(); if ($path === '/login') { $username = $request->post('username'); $password = $request->post('password'); if (!isset($users[$username]) || $users[$username] !== $password) { $response = new Response(401, ['Content-Type' => 'application/json'], json_encode(['error' => 'Invalid credentials'])); $connection->send($response); } else { $token = generateToken(); $response = new Response(200, ['Content-Type' => 'application/json'], json_encode(['token' => $token])); $connection->send($response); } } elseif (substr($path, 0, 7) === '/api/v1') { $token = $request->header('Authorization'); if (!validateToken($token)) { $response = new Response(401, ['Content-Type' => 'application/json'], json_encode(['error' => 'Unauthorized'])); $connection->send($response); } else { // 处理请求逻辑 } } else { $response = new Response(404, ['Content-Type' => 'text/html'], 'Not found'); $connection->send($response); } }; Worker::runAll(); function generateToken() { // 生成Token逻辑 } function validateToken($token) { // 验证Token逻辑 }
- Permission control
Permission control refers to controlling the user's access to system resources. In the online chat system Permission control is usually carried out in the form of roles and permissions. The specific steps are as follows:
Step 1: Define the role and permission list and store it in the database.
Step 2: After the user logs in, the server obtains the permission list corresponding to the role based on the user's role.
Step 3: When the server processes the request, it determines whether the user has the permission to perform the operation based on the permissions required by the request. If it has permission, continue processing the request; otherwise, return an error message indicating insufficient permissions.
The following is a sample code that uses Workerman to implement permission control:
require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; use WorkermanConnectionAsyncTcpConnection; use WorkermanProtocolsHttpRequest; use WorkermanProtocolsHttpResponse; $worker = new Worker('http://0.0.0.0:8080'); $roles = [ 'admin' => ['create', 'read', 'update', 'delete'], 'user' => ['read'] ]; $worker->onMessage = function ($connection, Request $request) use ($roles) { $path = $request->path(); $role = getUserRole(); // 根据Token获取用户角色 if (!isset($roles[$role])) { $response = new Response(401, ['Content-Type' => 'application/json'], json_encode(['error' => 'Unauthorized'])); $connection->send($response); return; } $allowedPermissions = $roles[$role]; $requiredPermission = extractRequiredPermission($path); // 根据请求路径提取所需权限 if (!in_array($requiredPermission, $allowedPermissions)) { $response = new Response(403, ['Content-Type' => 'application/json'], json_encode(['error' => 'Forbidden'])); $connection->send($response); return; } // 处理请求逻辑 }; Worker::runAll(); function getUserRole() { // 根据Token获取用户角色的逻辑 } function extractRequiredPermission($path) { // 从请求路径中提取所需权限的逻辑 }
Through the above sample code, we can see that it is very simple to implement user authentication and permission control in Workerman. Through reasonable authentication and authorization mechanisms, the security of the online chat system and user rights can be effectively protected. Hope this article can be helpful to you.
The above is the detailed content of Use Workerman to realize user authentication and authority control of online chat system. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


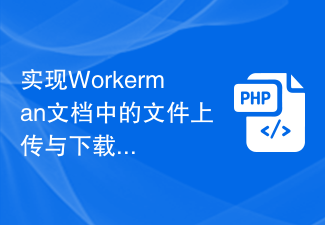
To implement file upload and download in Workerman documents, specific code examples are required. Introduction: Workerman is a high-performance PHP asynchronous network communication framework that is simple, efficient, and easy to use. In actual development, file uploading and downloading are common functional requirements. This article will introduce how to use the Workerman framework to implement file uploading and downloading, and give specific code examples. 1. File upload: File upload refers to the operation of transferring files on the local computer to the server. The following is used
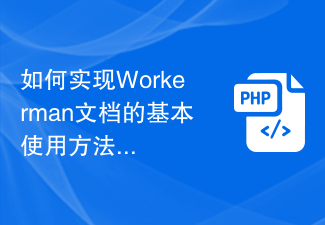
Introduction to how to implement the basic usage of Workerman documents: Workerman is a high-performance PHP development framework that can help developers easily build high-concurrency network applications. This article will introduce the basic usage of Workerman, including installation and configuration, creating services and listening ports, handling client requests, etc. And give corresponding code examples. 1. Install and configure Workerman. Enter the following command on the command line to install Workerman: c
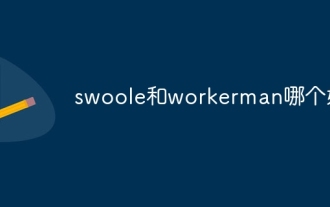
Swoole and Workerman are both high-performance PHP server frameworks. Known for its asynchronous processing, excellent performance, and scalability, Swoole is suitable for projects that need to handle a large number of concurrent requests and high throughput. Workerman offers the flexibility of both asynchronous and synchronous modes, with an intuitive API that is better suited for ease of use and projects that handle lower concurrency volumes.
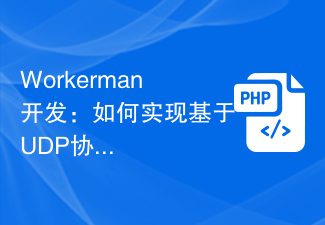
Workerman development: real-time video call based on UDP protocol Summary: This article will introduce how to use the Workerman framework to implement real-time video call function based on UDP protocol. We will have an in-depth understanding of the characteristics of the UDP protocol and show how to build a simple but complete real-time video call application through code examples. Introduction: In network communication, real-time video calling is a very important function. The traditional TCP protocol may have problems such as transmission delays when implementing high-real-time video calls. And UDP
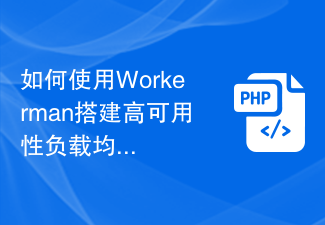
How to use Workerman to build a high-availability load balancing system requires specific code examples. In the field of modern technology, with the rapid development of the Internet, more and more websites and applications need to handle a large number of concurrent requests. In order to achieve high availability and high performance, the load balancing system has become one of the essential components. This article will introduce how to use the PHP open source framework Workerman to build a high-availability load balancing system and provide specific code examples. 1. Introduction to Workerman Worke
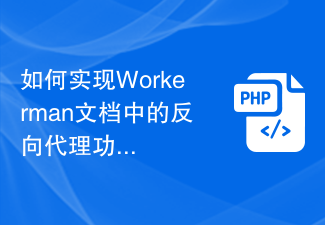
How to implement the reverse proxy function in the Workerman document requires specific code examples. Introduction: Workerman is a high-performance PHP multi-process network communication framework that provides rich functions and powerful performance and is widely used in Web real-time communication and long connections. Service scenarios. Among them, Workerman also supports the reverse proxy function, which can realize load balancing and static resource caching when the server provides external services. This article will introduce how to use Workerman to implement the reverse proxy function.
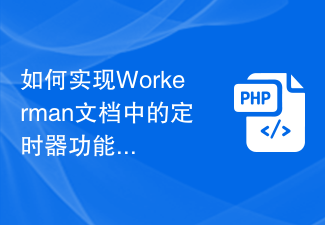
How to implement the timer function in the Workerman document Workerman is a powerful PHP asynchronous network communication framework that provides a wealth of functions, including the timer function. Use timers to execute code within specified time intervals, which is very suitable for application scenarios such as scheduled tasks and polling. Next, I will introduce in detail how to implement the timer function in Workerman and provide specific code examples. Step 1: Install Workerman First, we need to install Worker
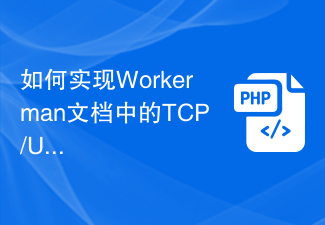
How to implement TCP/UDP communication in the Workerman document requires specific code examples. Workerman is a high-performance PHP asynchronous event-driven framework that is widely used to implement TCP and UDP communication. This article will introduce how to use Workerman to implement TCP and UDP-based communication and provide corresponding code examples. 1. Create a TCP server for TCP communication. It is very simple to create a TCP server using Workerman. You only need to write the following code: <?ph
