Reactive programming with JavaScript and RxJS
Reactive programming is a programming paradigm that handles asynchronous data flows. It's a way of writing code that responds to changes more quickly and handles events and data flow more efficiently.
In reactive programming, data is represented as a stream of events. These events can be anything from user input to network requests to database updates. The program then subscribes to these events and reacts when they occur.
This programming method has many advantages. First, it makes working with asynchronous data easier. In traditional programming, asynchronous data can be difficult to handle because it is difficult to know when the data will be available. Reactive programming, on the other hand, handles asynchronous data in a more natural way by treating it as a stream of events.
Secondly, reactive programming helps improve the performance of your code. By subscribing to events, your code can be notified as soon as new data is available, so it doesn't have to poll for data or wait for an event to occur.
Finally, reactive programming can help your code be more maintainable. By treating data as a stream of events, your code becomes more declarative and it is easier to understand how different parts of the code interact with each other.
RxJS
RxJS is a JavaScript library that provides a reactive programming API. It is a popular library used by many popular JavaScript frameworks such as Angular and React.
RxJS provides many features that make it ideal for reactive programming. These features include -
Observable objects− Observables are the basic building blocks of RxJS. They represent streams of events and can be used to represent any type of data, including numbers, strings, objects, and arrays.
Operators− Operators are functions that can be used to transform, filter, and combine Observables. There are a large number of operators available in RxJS, which make it possible to perform various operations with Observables.
Scheduler− The scheduler is used to control the time of Observables. They can be used to cause Observables to fire at specific times, or to delay the emission of events.
Install RxJS
To start using RxJS, we need to install it. Open a terminal and run the following command -
npm install rxjs
After the installation is complete, we can start exploring the power of RxJS reactive programming.
Create observable objects
Observables are at the heart of RxJS. They represent a stream of data that subscribers can observe.
Let's first create a simple Observable that emits a sequence of numbers -
Example
import { Observable } from 'rxjs'; const numberObservable = new Observable((observer) => { let count = 0; const interval = setInterval(() => { observer.next(count); count++; if (count > 5) { clearInterval(interval); observer.complete(); } }, 1000); }); numberObservable.subscribe((number) => { console.log(number); });
illustrate
In the above code, we create an Observable using the Observable class in RxJS. Inside the constructor, we define the logic for emitting values. In this example, we use setInterval to emit a number every second. Once the count reaches 5, we stop the interval and call observer.complete() to signal the end of the stream.
To observe the values emitted by the Observable, we call the subscribe method and provide a callback function. In this case, the callback function simply logs the emitted number to the console.
Output
0 1 2 3 4 5
Operators in RxJS
RxJS provides a wide range of operators that allow us to transform, filter, combine and manipulate the data emitted by Observables. Let's look at some common operators.
Map Operator
The map operator allows us to transform the values emitted by an Observable. For example, let's modify the previous example to double the number emitted -
Example
import { Observable } from 'rxjs'; import { map } from 'rxjs/operators'; const numberObservable = new Observable((observer) => { let count = 0; const interval = setInterval(() => { observer.next(count); count++; if (count > 5) { clearInterval(interval); observer.complete(); } }, 1000); }); numberObservable .pipe(map((number) => number * 2)) .subscribe((number) => { console.log(number); });
illustrate
In this code, we use the pipeline method to link the mapping operator to our Observable. The mapping operator takes a callback function that converts each emitted number by doubling it. The resulting value is then passed to the subscriber's callback function.
Output
0 2 4 6 8 10
Filter Operator
The filter operator allows us to selectively filter out the values emitted by an Observable based on conditions. Let's add a filter to the previous example to emit only even numbers -
示例
import { Observable } from 'rxjs'; import { filter } from 'rxjs/operators'; const numberObservable = new Observable((observer) => { let count = 0; const interval = setInterval(() => { observer.next(count); count++; if (count > 5) { clearInterval(interval); observer.complete(); } }, 1000); }); numberObservable .pipe(filter((number) => number % 2 === 0)) .subscribe((number) => { console.log(number); });
说明
在提供的代码中,我们创建了一个名为 numberObservable 的 Observable,它发出一系列数字。 Observable 使用 setInterval 发出从 0 开始的数字,每秒递增 1。发出数字 5 后,间隔被清除,Observable 使用observer.complete() 发出完成信号。
接下来,我们使用管道方法将过滤运算符应用于 numberObservable。过滤器运算符采用定义条件的回调函数。它过滤掉不满足条件的值,只允许偶数通过。
最后,我们订阅过滤后的 Observable,并使用订阅者的回调函数将每个发出的数字记录到控制台。
输出
0 2 4
结论
总之,使用 JavaScript 和 RxJS 进行响应式编程提供了一种强大而有效的方法来处理异步数据流和构建响应式应用程序。通过拥抱 Observables 的概念并利用 RxJS 提供的丰富的运算符集,开发人员可以以声明式且优雅的方式轻松操作、转换和组合数据流。
通过本文讨论的示例,我们了解了如何创建 Observables、应用映射和过滤器等运算符来转换和过滤发出的值,以及订阅 Observables 来接收和处理数据。 RxJS 通过提供一致且可组合的方法简化了复杂异步流的管理。
The above is the detailed content of Reactive programming with JavaScript and RxJS. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


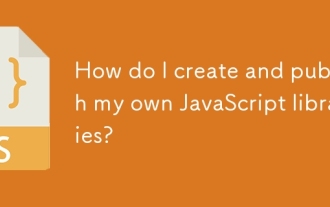
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
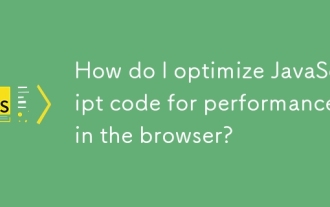
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
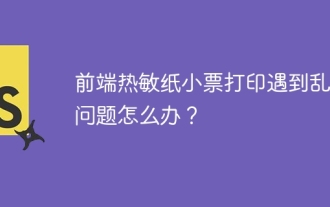
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
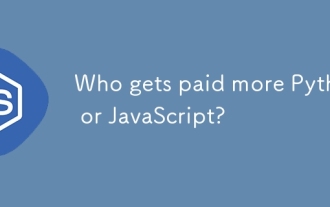
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
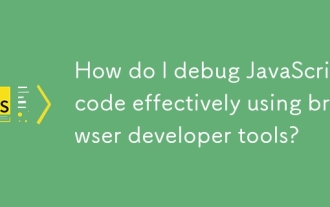
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
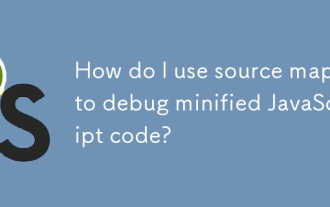
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
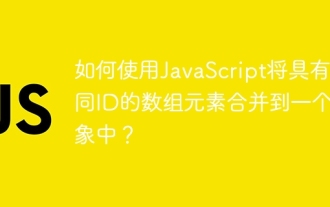
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
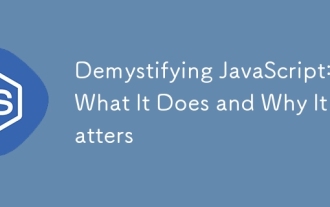
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
