


How to use PHP database connection to implement search function
How to use PHP database connection to implement search function
In modern websites and applications, search function is a common and important function. It enables users to quickly find the information they want and provides a good user experience. This article will introduce how to use PHP database connection to implement search functionality.
Before starting to implement the search function, you first need to establish a database and create a table to store the data to be searched. Suppose we want to create a simple employee information table that includes the employee's name, position, and salary. The following is the SQL statement to create the table:
CREATE TABLE employees ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255), position VARCHAR(255), salary DECIMAL(10, 2) );
Next, we need an HTML form to receive the user's search query. Here is a simple search form:
<form action="search.php" method="GET"> <input type="text" name="query" placeholder="输入员工姓名"> <input type="submit" value="搜索"> </form>
After submitting the form, we need to write a PHP script to handle the search query and retrieve relevant data from the database. Here is an example of a simple search.php script:
<?php // 提取用户搜索查询 $query = $_GET['query']; // 建立数据库连接 $host = 'localhost'; $username = 'root'; $password = 'password'; $dbname = 'my_database'; $connection = new mysqli($host, $username, $password, $dbname); // 检查连接是否成功 if ($connection->connect_error) { die('数据库连接失败: ' . $connection->connect_error); } // 构建SQL查询语句 $sql = "SELECT * FROM employees WHERE name LIKE '%$query%'"; // 执行查询 $result = $connection->query($sql); // 检查查询结果 if ($result->num_rows > 0) { // 输出每一行数据 while ($row = $result->fetch_assoc()) { echo "姓名: " . $row['name'] . ", 职位: " . $row['position'] . ", 薪水: $" . $row['salary'] . "<br>"; } } else { echo "没有找到相关员工信息"; } // 关闭数据库连接 $connection->close(); ?>
In the above script, we first extract the user search query and store it in the variable $query. We then establish a database connection and execute the SQL query. The LIKE statement is used here to fuzzy match employee names. If there are matching records in the query results, we will output the data of each row, otherwise we will output "No relevant employee information found". Finally, we close the database connection.
The above is a simple example of how to use PHP database connection to implement the search function. According to actual needs, you can make appropriate modifications and extensions according to your own database table structure and search requirements. I hope this article will help you implement the search function!
The above is the detailed content of How to use PHP database connection to implement search function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


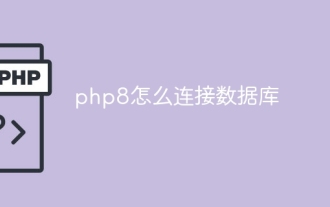
PHP8 can use mysqli and PDO to connect to the database. Detailed introduction: 1. Use mysqli to connect to the database by passing in the database server name, user name, password and database name to connect. Then, use the `connect_error` attribute to check whether the connection is successful and output an error message if the connection fails. Finally, close the connection by calling the `close()` method; 2. Use PDO to connect to the database, and connect by passing in the database server name, password and database name, etc.
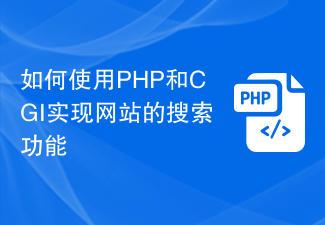
How to use PHP and CGI to implement the search function of the website In the modern Internet era, the search function of the website is indispensable. Users can quickly find the information they need through the search function, which improves user experience. This article will introduce how to use PHP and CGI to implement the search function of the website. 1. The concept of PHP and CGI PHP (full name PHP: Hypertext Preprocessor) is a widely used open source scripting language that can be embedded into HTML for processing
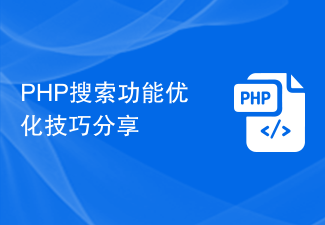
PHP search function has always been a very important part of website development, because users often use the search box to find the information they need. However, many websites have problems such as low efficiency and inaccurate search results when implementing search functions. In order to help you optimize PHP search function, this article will share some tips and provide specific code examples. 1. Use full-text search engines. Traditional SQL databases are less efficient when processing large amounts of text content. Therefore, it is recommended to use full-text search engines, such as Elasticsearch, Solr, etc.
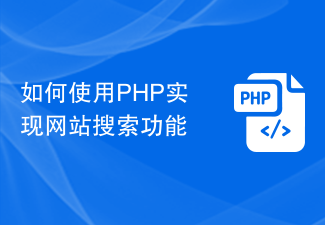
How to use PHP to implement website search function With the development of the Internet, various websites have emerged. Search functionality is an essential part of most websites. In this article, I will introduce how to use PHP language to implement a simple website search function and provide specific code examples. First, we need a database to store the content of the website. We can use MySQL database to store articles, products or other information for the website. In this example, we will create a table called "articles" where
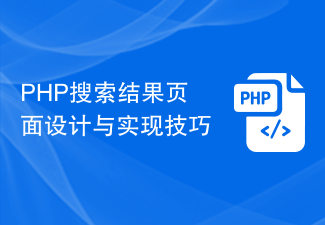
With the explosive growth of information on the Internet, search functionality has become a vital part of websites and applications. As a popular server-side scripting language, PHP is widely used in website development. This article will discuss the design and implementation techniques of PHP search results pages, combined with specific code examples, to help developers better implement search functions. 1. Page design 1. Search box design The search box is the core component of the search page, usually located at the top of the page. Design a concise and clear search box where users can enter keywords to search
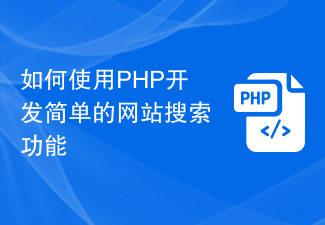
How to use PHP to develop a simple website search function. The rapid development of the Internet has caused the amount of information to explode. In order to allow users to find the information they need more conveniently, the website search function has become a basic element of modern websites. In this article, we will introduce how to use PHP to develop a simple website search function and provide specific code examples. 1. Database preparation First, we need to prepare a database to store the content data of the website. Let's assume that there is a table named "articles" in the database,
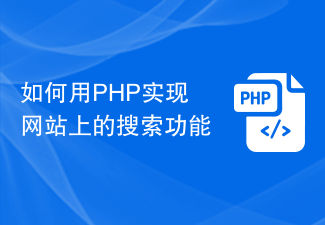
With the popularity and development of the Internet, websites have become one of the important channels for people to obtain information and services. The search function on the website is one of the important tools for users to quickly obtain the information they need. This article gives a brief description of how to use PHP to implement the search function on the website. 1. Basic principles of the search function To implement the search function on the website, the following four basic steps need to be completed: 1. The user enters keywords to search; 2. The website searches the database based on the keywords; 3. The website extracts data that meets the search conditions; 4. The website will match the search criteria
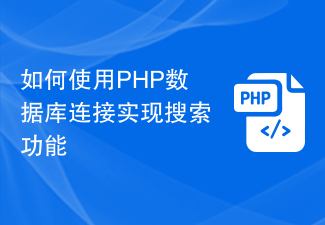
How to use PHP database connection to implement search function In modern websites and applications, search function is a common and important function. It enables users to quickly find the information they want and provides a good user experience. This article will introduce how to use PHP database connection to implement search functionality. Before starting to implement the search function, you first need to set up a database and create a table to store the data to be searched. Suppose we want to create a simple employee information table that includes the employee's name, position, and salary. Below is the S that creates the table
