


Written in C++, find the number of segments where all elements are greater than X
In this article, we need to find the number of segments or sub-arrays in a given sequence that are greater than a given number X.
We can only count overlapping segments once, two adjacent elements or segments should not be counted separately. So here is a basic example of the given problem −
Input : arr[ ] = { 9, 6, 7, 11, 5, 7, 8, 10, 3}, X = 7 Output : 3 Explanation : { 9 }, { 11 } and { 8, 10 } are the segments greater than 7 Input : arr[ ] = { 9, 6, 12, 2, 11, 14, 8, 14 }, X = 8 Output : 4 Explanation : { 9 }, { 12 }, { 11, 14 } and { 14 } are the segments greater than 8
Ways to find the solution
Naive way
In this problem we initialize the variables with 0state and start processing the given array, when an element greater than X is found, change the state to 1 and continue processing elements; when a number less than or equal to X is found, change the state back to 0, each time When the status changes to 1 and returns, count is increased by 1 to 0.
Example
#include <bits/stdc++.h> using namespace std; int main (){ int a[] = { 9, 6, 12, 2, 11, 14, 8, 14 }; int n = sizeof (a) / sizeof (a[0]); int X = 8; int state = 0; int count = 0; // traverse the array for (int i = 0; i < n; i++){ // checking whether element is greater than X if (a[i] > X){ state = 1; } else{ // if flag is true if (state) count += 1; state = 0; } } // checking for the last segment if (state) count += 1; cout << "Number of segments where all elements are greater than X: " << count; return 0; }
Output
Number of segments where all elements are greater than X: 4
Description of the above program
In the above program, we use the state as a switch when a number greater than X is found Set it to 1, when we find a number greater than X we set it to 0 when we find a number less than or equal to Finally, print the results stored in the count.
Conclusion h2>
In this article, we solved the problem of finding the number of segments where all elements are greater than X by applying a method of setting the state to 1 and 0 whenever a segment is found. We can write this program in any other programming language like C, Java, Python, etc.
The above is the detailed content of Written in C++, find the number of segments where all elements are greater than X. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
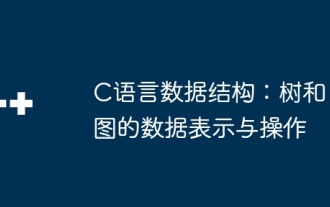
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
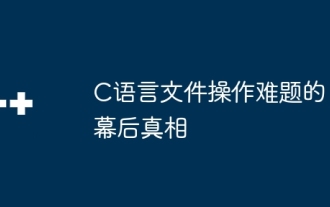
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
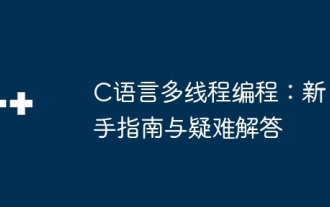
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
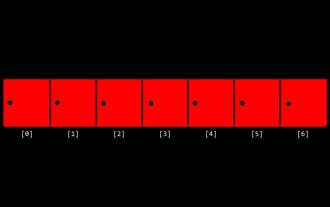
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
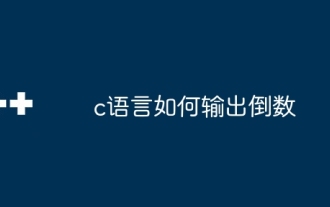
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
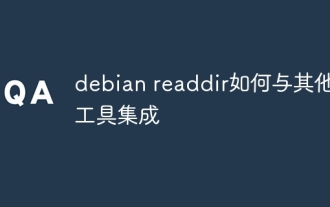
The readdir function in the Debian system is a system call used to read directory contents and is often used in C programming. This article will explain how to integrate readdir with other tools to enhance its functionality. Method 1: Combining C language program and pipeline First, write a C program to call the readdir function and output the result: #include#include#include#includeintmain(intargc,char*argv[]){DIR*dir;structdirent*entry;if(argc!=2){
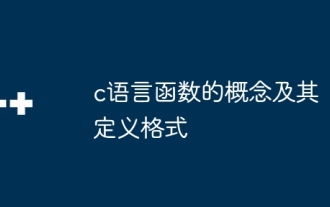
C language functions are reusable code blocks, receive parameters for processing, and return results. It is similar to the Swiss Army Knife, powerful and requires careful use. Functions include elements such as defining formats, parameters, return values, and function bodies. Advanced usage includes function pointers, recursive functions, and callback functions. Common errors are type mismatch and forgetting to declare prototypes. Debugging skills include printing variables and using a debugger. Performance optimization uses inline functions. Function design should follow the principle of single responsibility. Proficiency in C language functions can significantly improve programming efficiency and code quality.
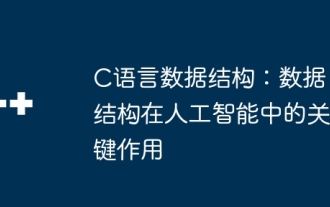
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
