In C/C++, the strcmp() function is used to compare two strings
The function strcmp() is a built-in library function and it is declared in “string.h” header file. This function is used to compare the string arguments. It compares strings lexicographically which means it compares both the strings character by character. It starts comparing the very first character of strings until the characters of both strings are equal or NULL character is found.
If the first character of both strings are equal, it checks second character and so on. This process will be continued until NULL character is found or both characters are unequal.
Here is the syntax of strcmp() in C language,
int strcmp(const char *leftStr, const char *rightStr );
This function returns the following three different values based on the comparison.
1.Zero(0) − It returns zero if both strings are identical. All characters are same in both strings.
Here is an example of strcmp() when both strings are equal in C language,
Example
Live Demo
#include<stdio.h> #include<string.h> int main() { char str1[] = "Tom!"; char str2[] = "Tom!"; int result = strcmp(str1, str2); if (result==0) printf("Strings are equal"); else printf("Strings are unequal"); printf("\nValue returned by strcmp() is: %d" , result); return 0; }
Output
Strings are equal Value returned by strcmp() is: 0
2.大于零(>0) − 当左字符串的匹配字符的ASCII值大于右字符串的字符时,它返回一个大于零的值。
这里是C语言中strcmp()返回大于零值的一个例子,
示例
在线演示
#include<stdio.h> #include<string.h> int main() { char str1[] = "hello World!"; char str2[] = "Hello World!"; int result = strcmp(str1, str2); if (result==0) printf("Strings are equal"); else printf("Strings are unequal"); printf("\nValue returned by strcmp() is: %d" , result); return 0; }
Output
Strings are unequal Value returned by strcmp() is: 32
3.小于零(<0) − 当左字符串的匹配字符的ASCII值小于右字符串的字符时,它返回一个小于零的值。
下面是C语言中strcmp()的一个例子
例子
在线演示
#include<stdio.h> #include<string.h> int main() { char leftStr[] = "Hello World!"; char rightStr[] = "hello World!"; int result = strcmp(leftStr, rightStr); if (result==0) printf("Strings are equal"); else printf("Strings are unequal"); printf("\nValue returned by strcmp() is: %d" , result); return 0; }
Output
Strings are unequal Value returned by strcmp() is: -32
The above is the detailed content of In C/C++, the strcmp() function is used to compare two strings. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
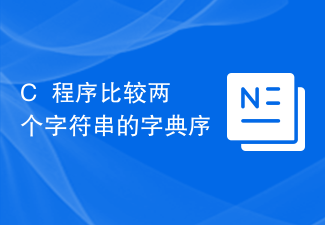
Lexicographic string comparison means that strings are compared in dictionary order. For example, if there are two strings 'apple' and 'appeal', the first string will come last because the first three characters of 'app' are the same. Then for the first string the character is 'l' and in the second string the fourth character is 'e'. Since 'e' is shorter than 'l', it will come first if we sort lexicographically. Strings are compared lexicographically before being arranged. In this article, we will see different techniques for lexicographically comparing two strings using C++. Using the compare() function in C++ strings The C++string object has a compare()
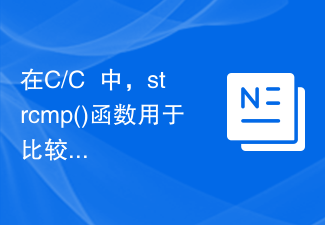
Thefunctionstrcmp()isabuilt-inlibraryfunctionanditisdeclaredin“string.h”headerfile.Thisfunctionisusedtocomparethestringarguments.Itcomparesstringslexicographicallywhichmeansitcomparesboththestringscharacterbycharacter.Itstartscomp
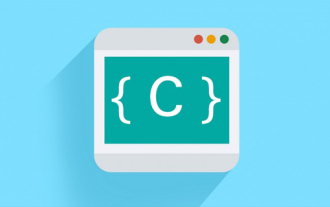
Comparison methods: 1. bcmp(), compares whether the first n bytes of a string are equal; 2. strcmp(), compares strings in a case-sensitive manner; 3. strictmp(), compares strings in a case-insensitive manner; 4. strncmp() or strnicmp(), case-sensitive comparison of the first n characters of a string.
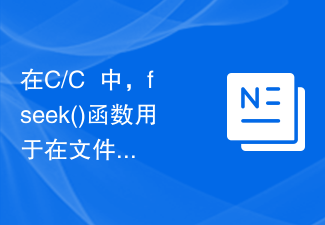
fseek() is used in C language to move the file pointer to a specific location. Offsets and streams are the targets of pointers, and they are given in function arguments. If successful, it returns zero. If unsuccessful, it returns a non-zero value. The following is the syntax of fseek() in C language: intfseek(FILE*stream,longintoffset,intwhence) Here are the parameters used in fseek(): stream− This is the pointer used to identify the stream. offset − This is the number of bytes from the position. whence−This is where the offset is added. whence is given by the following constants
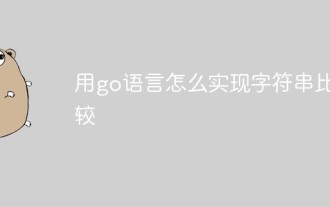
How to compare strings in Go language: 1. Use the "==" operator, the syntax "String 1 == String 2"; 2. Use the ToLower() function of the strings package; 3. Use the Compare() of the strings package Function that can compare two strings in dictionary order, the syntax is "strings.Compare(str1,str2)"; 4. Use the EqualFold() function of the strings package to compare strings that ignore case, and the return value is of bool type.
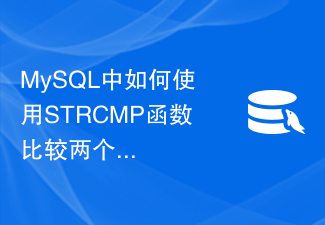
How to use the STRCMP function in MySQL to compare the sizes of two strings In MySQL, you can use the STRCMP function to compare the sizes of two strings. The STRCMP function compares two strings according to their lexicographic order and returns an integer value representing the comparison result. The syntax of the STRCMP function is as follows: STRCMP(str1,str2) where str1 and str2 are the two strings to be compared. The return values of the STRCMP function are as follows:
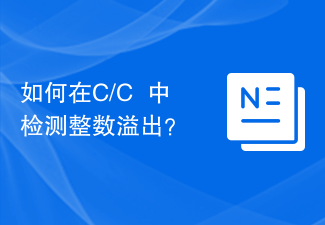
The only safe way is to check before the overflow occurs. There are some informal ways to check for integer overflow though. So, if your goal is to detect overflow when adding unsigned integers, you can check whether the result is actually smaller than the two added values. For example, the sample code unsignedintx,y;unsignedintvalue=x+y;booloverflow=value<x;//Alternatively "value<y" should also work. This is because if x and y are both unsigned integers, if they are added and overflow, their Values cannot be greater than any of them, as they require

The idea is to realize the fact that greedy methods provide the best solution to the fractional knapsack problem. To check if a specific node can provide us with a better solution, we calculate the best solution (by node) implementing a greedy approach. If the greedy method itself computes more solutions than the best solution so far, then we cannot get to a better solution through the nodes. The complete algorithm is as follows - All items are sorted in descending order of value per unit weight ratio so that the upper limit can be calculated using the greedy method. Initialize the maximum profit, for example maxProfit=0 to create an empty queue Q. Decision virtual nodes create trees and insert or queue them into Q. The profit and weight of the virtual node are 0. Perform the following operations when Q is not empty or empty. create
