


From Beginner to Mastery: A Complete Guide to PHP WebSocket Development and Implementation
From Beginner to Mastery: A Complete Guide to PHP WebSocket Development and Implementation Functions
Introduction:
WebSocket is an emerging network communication protocol that allows web applications The program conducts real-time two-way communication with the server without relying on the traditional HTTP request-response model. PHP is a popular server-side programming language that can be used to develop high-performance, real-time web applications. This article will introduce the basic knowledge and skills of PHP WebSocket development, and provide a complete guide to help readers from getting started to mastering WebSocket development.
1. Understanding the WebSocket protocol
First of all, we need to understand the basic principles and characteristics of the WebSocket protocol. WebSocket uses full-duplex communication, allowing the server to actively push data to the client, achieving higher real-time communication. Compared with the traditional HTTP protocol, WebSocket maintains a long connection after establishing a connection, thus avoiding the overhead of re-establishing the connection for each communication.
2. Build a WebSocket server
Before starting PHP WebSocket development, we need to build a WebSocket server. PHP does not natively support WebSocket, but we can use third-party libraries to implement WebSocket functionality. Commonly used WebSocket libraries include Ratchet and Swoole. This article uses Ratchet as an example to explain.
First, we need to install Ratchet through Composer. Execute the following command in the command line:
$ composer require cboden/ratchet
Then, create a PHP script, such as server.php
:
<?php require 'vendor/autoload.php'; use RatchetMessageComponentInterface; use RatchetConnectionInterface; use RatchetServerIoServer; use RatchetHttpHttpServer; use RatchetWebSocketWsServer; class MyChat implements MessageComponentInterface { public function onOpen(ConnectionInterface $conn) { // 新连接建立时触发 } public function onMessage(ConnectionInterface $from, $msg) { // 收到消息时触发 } public function onClose(ConnectionInterface $conn) { // 连接关闭时触发 } public function onError(ConnectionInterface $conn, Exception $e) { // 发生错误时触发 } } $server = IoServer::factory( new HttpServer( new WsServer( new MyChat() ) ), 8080 ); $server->run();
The above code creates a file named The class of MyChat
and implements the MessageComponentInterface
interface. In the MyChat
class, we can define specific logic to handle operations such as connection establishment, message reception, and connection closing.
In the final code, we create a WebSocket server through the class provided by Ratchet. The port number defined in the configuration file is 8080, which can be modified according to needs.
3. Implement the WebSocket function
After completing the construction of the server, we can start to implement the specific WebSocket function. In the MyChat
class, we can define different operations according to our needs.
For example, we can implement the logic when establishing a new connection in the onOpen
method, such as sending a welcome message to other clients:
public function onOpen(ConnectionInterface $conn) { echo "New connection! ({$conn->resourceId}) "; $conn->send("Welcome! ({$conn->resourceId})"); // 向其他客户端发送消息 foreach ($this->clients as $client) { if ($conn !== $client) { $client->send("New connection! ({$conn->resourceId})"); } } $this->clients->attach($conn); }
In onMessage
In the method, we can implement the logic after receiving the message, such as broadcasting the message to other clients:
public function onMessage(ConnectionInterface $from, $msg) { echo "Received message: {$msg} "; // 向其他客户端广播消息 foreach ($this->clients as $client) { if ($from !== $client) { $client->send("Message from {$from->resourceId}: {$msg}"); } } }
In the onClose
method, we can implement the logic when the connection is closed, Such as sending leave messages to other clients:
public function onClose(ConnectionInterface $conn) { echo "Connection {$conn->resourceId} has disconnected "; // 向其他客户端发送消息 foreach ($this->clients as $client) { if ($conn !== $client) { $client->send("Connection {$conn->resourceId} has disconnected"); } } $this->clients->detach($conn); }
Through the above method, we can implement basic WebSocket functions. Depending on specific needs, we can also handle error conditions in the onError
method.
4. Using the WebSocket protocol
After completing the construction of the server and the implementation of the functions, we can use the WebSocket protocol for communication.
On the client side, we can use JavaScript to create a WebSocket object and establish a connection with the server:
var conn = new WebSocket('ws://localhost:8080'); conn.onopen = function() { console.log('Connected'); conn.send('Hello, server!'); }; conn.onmessage = function(e) { console.log('Received message: ' + e.data); }; conn.onclose = function() { console.log('Connection closed'); }; conn.onerror = function() { console.log('Error occurred'); };
On the server side, we can use the methods provided by Ratchet to handle the connection and message reception :
public function onOpen(ConnectionInterface $conn) { // 新连接建立时触发 } public function onMessage(ConnectionInterface $from, $msg) { // 收到消息时触发 } public function onClose(ConnectionInterface $conn) { // 连接关闭时触发 } public function onError(ConnectionInterface $conn, Exception $e) { // 发生错误时触发 }
Through the above code, we can implement basic two-way communication functions and realize web applications with higher real-time performance.
Summary:
This article introduces the basic knowledge and skills of PHP WebSocket development, and provides a complete guide from entry to mastery. By understanding the WebSocket protocol, building a WebSocket server and implementing WebSocket functions, we can quickly get started and develop high-performance, real-time Web applications. I hope this article can help readers go from getting started to becoming proficient in WebSocket development and play a greater role in actual projects.
The above is the detailed content of From Beginner to Mastery: A Complete Guide to PHP WebSocket Development and Implementation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



How to use Laravel to implement image processing functions requires specific code examples. Nowadays, with the development of the Internet, image processing has become an indispensable part of website development. Laravel is a popular PHP framework that provides us with many convenient tools to process images. This article will introduce how to use Laravel to implement image processing functions, and give specific code examples. Install LaravelInterventionImageInterven
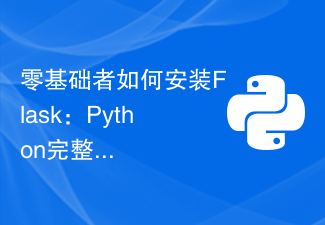
Starting from Scratch: A Complete Guide to Installing Flask in Python Introduction Flask is a lightweight Python web framework that is widely used to develop simple and flexible web applications. This article will provide you with a complete guide on how to install Flask from scratch and provide some commonly used code examples. Installing Python First, you need to install Python. You can download it from the Python official website (https://www.python.org)
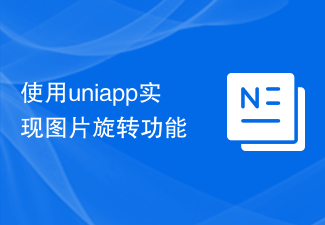
Using uniapp to implement image rotation function In mobile application development, we often encounter scenarios where images need to be rotated. For example, the angle needs to be adjusted after taking a photo, or an effect similar to the rotation of a camera after taking a photo is achieved. This article will introduce how to use the uniapp framework to implement the image rotation function and provide specific code examples. uniapp is a cross-platform development framework based on Vue.js, which can simultaneously develop and publish applications for iOS, Android, H5 and other platforms. Implemented in uniapp
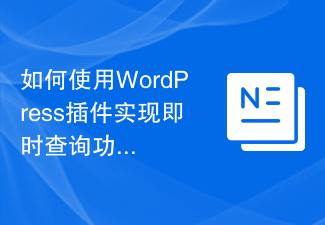
How to use WordPress plug-ins to achieve instant query function WordPress is a powerful blog and website building platform. Using WordPress plug-ins can further expand the functions of the website. In many cases, users need to perform real-time queries to obtain the latest data. Next, we will introduce how to use WordPress plug-ins to implement instant query functions and provide some code samples for reference. First, we need to choose a suitable WordPress plug-in to achieve instant query
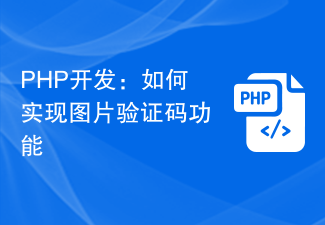
PHP development: How to implement the image verification code function In WEB development, in order to prevent robots or malicious attacks, it is often necessary to use verification codes to verify the user's identity. Among them, picture verification code is a common type of verification code, which can not only effectively identify users, but also improve user experience. This article will introduce how to use PHP to implement the image verification code function and provide specific code examples. 1. Generate verification code images First, we need to generate verification code images with random characters. PHP provides the GD library to easily generate images. the following
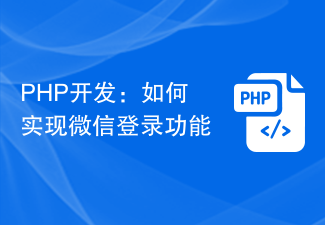
PHP development: How to implement the WeChat login function, specific code examples are required Introduction: With the rapid development of the mobile Internet, WeChat, as one of China's largest social media platforms, plays an important role in application development. WeChat login is a common login method in many applications and websites, providing a convenient, fast and secure authentication method. This article will introduce how to use PHP to implement the WeChat login function and provide specific code examples. Step 1: Apply for a WeChat open platform account and create an application. Before starting, we need to apply first
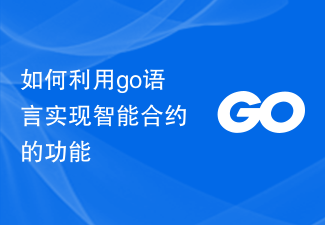
How to use Go language to implement the functions of smart contracts. Smart contracts are a form of contract based on blockchain technology. It runs on the blockchain and can automatically execute the agreements in it. In recent years, smart contracts have received widespread attention and application and can be used to implement automated business logic in a variety of scenarios. This article will introduce how to use Go language to implement smart contract functions and provide corresponding code examples. 1. Blockchain development library in Go language. Before using Go language to develop smart contracts, we need to choose a suitable blockchain development library. Head
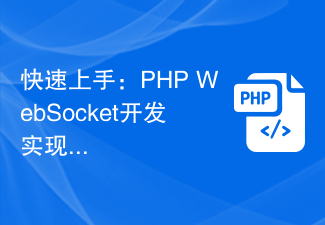
Get started quickly: Tutorial on PHP WebSocket development and implementation Introduction: WebSocket is an open standard protocol for real-time communication. It can establish a persistent connection between the browser and the server to achieve two-way communication. In Web development, WebSocket is widely used in real-time chat, real-time notification, multi-person collaboration and other scenarios. This article will introduce how to use PHP to develop WebSocket applications and quickly implement functions. 1. What is WebSocket? WebSoc
