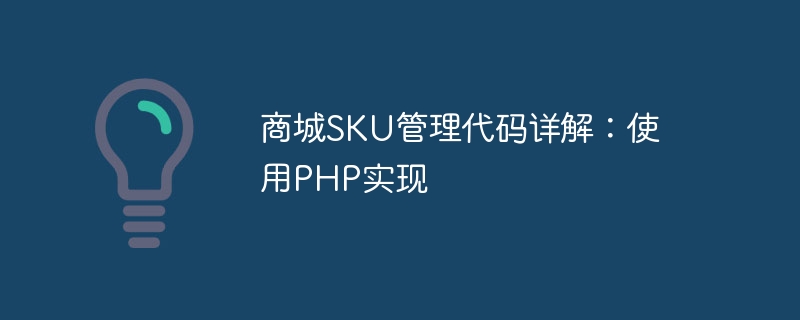
Mall SKU management is a very important function in the e-commerce platform. It is mainly used to manage the inventory, price, attributes, etc. of goods. In actual development, in order to facilitate SKU management, a specific code implementation is usually used. In this article, I will introduce in detail how to use PHP code to manage SKUs in the mall.
- The concept of SKU
SKU is the abbreviation of Stock Keeping Unit, that is, the inventory unit. It is used to uniquely identify each product in the mall, and each SKU corresponds to a different combination of product attributes. Product attributes include color, size, version, etc. Different attribute combinations correspond to different SKUs.
- Database Design
In order to implement SKU management, you first need to design the database table structure. Generally speaking, at least two tables are needed. One is the product table, which is used to store basic information about the product, such as product ID, name, description, etc.; the other is the SKU table, which is used to store the attribute information of the product, such as SKU ID, Product ID, attribute combination, inventory, price, etc.
- Commodity attribute combination
The attribute combination of the product is the key to SKU management. Each product can have multiple attributes, and each attribute can have multiple options. For example, a T-shirt has two attributes: color and size. The color has three options: red, blue, and black. The size has three options: S, M, and L. Then this T-shirt has 3 color options and 3 size options, totaling 9 SKUs.
- SKU coding rules
In order to facilitate management and query, each SKU needs to have a unique code. The encoding generally consists of IDs of attribute options, separated by commas. For example, the code for a red T-shirt is "1,4", which means red and M code.
- SKU management code implementation
In PHP code, SKU management can be achieved through multi-dimensional arrays. First, read and parse product attribute information and attribute option information, and organize them into multi-dimensional arrays. Then, all possible SKU codes are generated based on the attribute combination of the product and stored in the database.
- SKU query and update
An important function of using SKU management is to query and update SKU information. When querying SKU information, you can obtain all corresponding SKU information based on the product ID. When updating SKU information, you can update inventory, price and other information based on the SKU ID.
- Sample code
The following is a simple sample code that demonstrates how to implement SKU management:
// 获取商品属性信息和属性选项信息
$properties = [
['id' => 1, 'name' => '颜色', 'options' => ['红色', '蓝色', '黑色']],
['id' => 2, 'name' => '尺寸', 'options' => ['S码', 'M码', 'L码']]
];
// 生成所有可能的SKU编码
$skus = [];
foreach ($properties[0]['options'] as $color) {
foreach ($properties[1]['options'] as $size) {
$code = $properties[0]['id'] . ',' . $properties[1]['id'];
$sku = [
'code' => $code,
'color' => $color,
'size' => $size,
'stock' => 100,
'price' => 99.99
];
$skus[] = $sku;
}
}
// 将SKU信息存储到数据库中
foreach ($skus as $sku) {
$sql = "INSERT INTO sku (code, color, size, stock, price)
VALUES ('{$sku['code']}', '{$sku['color']}', '{$sku['size']}', {$sku['stock']}, {$sku['price']})";
// 执行SQL语句
// ...
}
// 查询SKU信息
$productId = 1; // 商品ID
$sql = "SELECT * FROM sku WHERE product_id = {$productId}";
// 执行SQL语句
// ...
// 更新SKU信息
$skuId = 1; // SKU ID
$newStock = 50; // 新的库存量
$sql = "UPDATE sku SET stock = {$newStock} WHERE sku_id = {$skuId}";
// 执行SQL语句
// ...
Copy after login
Through the above sample code, basic mall SKU management functions can be achieved. Of course, there may be more details and complexities in actual development, such as dynamic addition of product attributes, SKU image management, etc. But the core ideas are the same and can be expanded and optimized according to actual needs.
Summary:
Mall SKU management is one of the important functions in the e-commerce platform. SKU management can be easily achieved using PHP code. By designing the database table structure, generating SKU codes, and implementing SKU query and update operations, you can effectively manage product attributes and inventory information.
The above is the detailed content of Detailed explanation of mall SKU management code: implemented using PHP. For more information, please follow other related articles on the PHP Chinese website!