Given a string in which letters represent numbers scrambled
In today's article, we will delve into a unique issue related to string manipulation in C. This question is "In the given string, the alphabetic expression is scrambled with numbers." This question can serve as a good exercise to improve your string manipulation and data structure skills in C.
Problem Statement
Given a string, the task is to identify numbers in which letter expressions are scrambled. For example, if the input string is "oentow", it has an alphabetical representation of the number 2 (t, w, o) and the number 1 (o, n, e) scrambled.
C Solution Method
To solve this problem, we will use a hash table or unordered map in C to store the frequency of letters in a string. We will then compare this frequency map to a predefined map of the alphabetical representation of each number. If a representation of a number can be formed from the input string, we will output that number.
The Chinese translation ofExample
is:Example
This is the C code to solve the problem −
#include <iostream> #include <unordered_map> #include <vector> // Array of digit representations std::string digitRepresentations[10] = {"zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"}; std::unordered_map<char, int> generateFrequencyMap(std::string str) { std::unordered_map<char, int> freqMap; for (char c : str) { freqMap[c]++; } return freqMap; } std::vector<int> findJumbledDigits(std::string str) { std::unordered_map<char, int> strFreqMap = generateFrequencyMap(str); std::vector<int> digits; for (int i = 0; i < 10; i++) { std::unordered_map<char, int> digitFreqMap = generateFrequencyMap(digitRepresentations[i]); bool canFormDigit = true; for (auto pair : digitFreqMap) { if (strFreqMap[pair.first] < pair.second) { canFormDigit = false; break; } } if (canFormDigit) { digits.push_back(i); } } return digits; } int main() { std::string input = "oentow"; std::vector<int> digits = findJumbledDigits(input); std::cout << "The jumbled digits in the string are: "; for (int digit : digits) { std::cout << digit << " "; } return 0; }
Output
The jumbled digits in the string are: 1 2
Explanation with a Test Case
is translated as:Explanation with a Test Case
Let's consider the string "oentow".
When this string is passed to the findJumbledDigits function, it first generates a frequency map for the string: {'o': 2, 'e': 1, 'n': 1, 't': 1, ' w': 1}.
Then, for each number from 0 to 9, it generates a frequency map of the alphabetical representation of the number and checks whether this map can be formed from the frequency map of the string.
The representation "one" of the number 1 has the frequency mapping {'o': 1, 'n': 1, 'e': 1}, while the representation "two" of the number 2 has the frequency mapping {'t' : 1, 'w': 1, 'o': 1}.
These two can be generated by a frequency map of strings, so we add these numbers to the result.
Finally, it outputs the result: "The jumbled digits in the string are: 1 2".
in conclusion
This question shows how we can use frequency mapping to solve complex string manipulation problems in C. This is a great question to practice your string and data structure handling skills. Keep practicing questions like this to improve your C coding skills.
The above is the detailed content of Given a string in which letters represent numbers scrambled. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
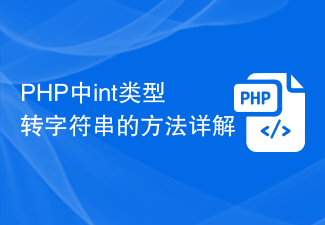
Detailed explanation of the method of converting int type to string in PHP In PHP development, we often encounter the need to convert int type to string type. This conversion can be achieved in a variety of ways. This article will introduce several common methods in detail, with specific code examples to help readers better understand. 1. Use PHP’s built-in function strval(). PHP provides a built-in function strval() that can convert variables of different types into string types. When we need to convert int type to string type,
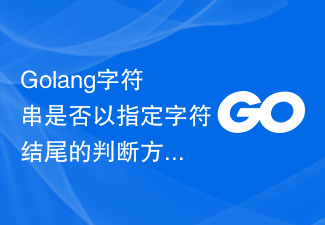
Title: How to determine whether a string ends with a specific character in Golang. In the Go language, sometimes we need to determine whether a string ends with a specific character. This is very common when processing strings. This article will introduce how to use the Go language to implement this function, and provide code examples for your reference. First, let's take a look at how to determine whether a string ends with a specified character in Golang. The characters in a string in Golang can be obtained through indexing, and the length of the string can be
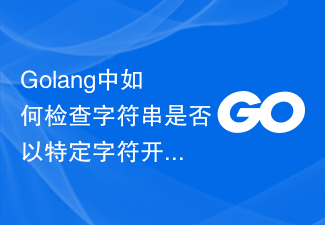
How to check if a string starts with a specific character in Golang? When programming in Golang, you often encounter situations where you need to check whether a string begins with a specific character. To meet this requirement, we can use the functions provided by the strings package in Golang to achieve this. Next, we will introduce in detail how to use Golang to check whether a string starts with a specific character, with specific code examples. In Golang, we can use HasPrefix from the strings package
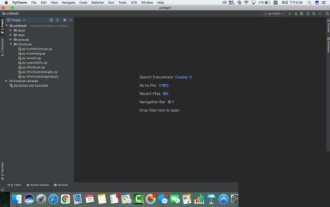
1. First open pycharm and enter the pycharm homepage. 2. Then create a new python script, right-click - click new - click pythonfile. 3. Enter a string, code: s="-". 4. Then you need to repeat the symbols in the string 20 times, code: s1=s*20. 5. Enter the print output code, code: print(s1). 6. Finally run the script and you will see our return value at the bottom: - repeated 20 times.
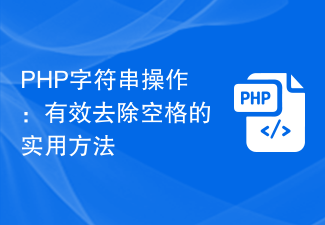
PHP String Operation: A Practical Method to Effectively Remove Spaces In PHP development, you often encounter situations where you need to remove spaces from a string. Removing spaces can make the string cleaner and facilitate subsequent data processing and display. This article will introduce several effective and practical methods for removing spaces, and attach specific code examples. Method 1: Use the PHP built-in function trim(). The PHP built-in function trim() can remove spaces at both ends of the string (including spaces, tabs, newlines, etc.). It is very convenient and easy to use.
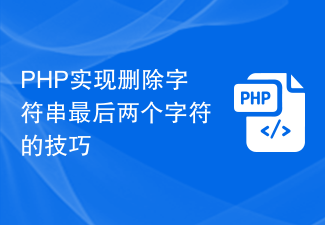
As a scripting language widely used to develop web applications, PHP has very powerful string processing functions. In daily development, we often encounter operations that require deleting a string, especially the last two characters of the string. This article will introduce two PHP techniques for deleting the last two characters of a string and provide specific code examples. Tip 1: Use the substr function The substr function in PHP is used to return a part of a string. We can easily remove characters by specifying the string and starting position
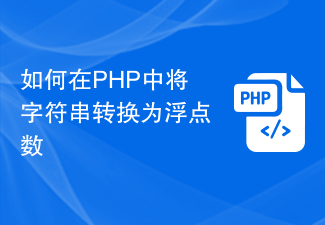
Converting a string to a floating point number is a common operation in PHP and can be accomplished through built-in methods. First make sure that the string is in a legal floating point format before it can be successfully converted to a floating point number. The following will detail how to convert a string to a floating point number in PHP and provide specific code examples. 1. Use (float) cast In PHP, the simplest way to convert a string into a floating point number is to use cast. The way to force conversion is to add (float) before the string, and PHP will automatically convert it
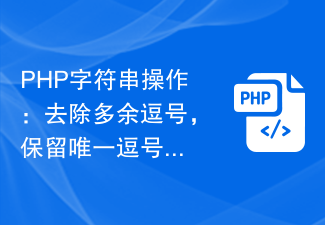
PHP String Operation: Remove Extra Commas and Keep Only Commas Implementation Tips In PHP development, string processing is a very common requirement. Sometimes we need to process the string to remove extra commas and retain the only commas. In this article, I'll introduce an implementation technique and provide concrete code examples. First, let's look at a common requirement: Suppose we have a string containing multiple commas, and we need to remove the extra commas and keep only the unique comma. For example, replace "apple,ba
