自己动手做一个SQL解释器_PHP
自己动手做一个SQL解释器
在一些小型的应用中,完全没有必要使用大型数据库软件。自己做一个SQL解释器就能用数据库的方式来管理了。
这个解释器,能解释常用的SQL命令。你可以自行添加其他功能。
class DB_text {
var $conn;
var $classname = "db_text";
var $database;
function on_create() {
}
function connect($database_name) {
$this->database = $database_name;
if(! file_exists($database_name)) {
$this->conn = array();
$this->_close();
}
$fp = fopen($this->database,"r");
$this->conn = unserialize(fread($fp,filesize($this->database)));
fclose($fp);
}
function &query($query) {
if(eregi("select ",$query)) return $this->_select($query);
if(eregi("insert ",$query)) return $this->_insert($query);
if(eregi("delete ",$query)) return $this->_delete($query);
if(eregi("update ",$query)) return $this->_update($query);
return array();
}
function fetch_row(&$result) {
if(list($key,$value) = each($result))
return $value;
return false;
}
function num_rows($result) {
return count($result);
}
/**
* query的辅助函数
*/
function _select($query) {
if(eregi("(order by (. ))",$query,$regs)) {
$order = $regs[2];
$query = eregi_replace($regs[1],"",$query);
}
if(eregi("(group by (. ))",$query,$regs)) {
$group = $regs[2];
$query = eregi_replace($regs[1],"",$query);
}
eregi("select .* from ([0-9a-z_] ) *(where (. ))?",$query,$regs);
if($regs[3] != "") {
$keys = $this->_where($regs[3],"\$this->conn[$regs[1]]");
while(list($key,$value) = each($keys)) {
$rs[] = $this->conn[$regs[1]][$value];
}
}else {
$rs = $this->conn[$regs[1]];
}
if($order) {
sscanf($order,"%s %s",$key,$type);
if(empty($type)) $type = "asc";
$this->_sort($rs,$key,$type);
}
return $rs;
}
function _insert($query) {
eregi("insert into ([0-9a-z_] ) *(. ) *values? *(. )",$query,$regs);
eval("\$key=array$regs[2];");
eval("\$value=array$regs[3];");
for($i=0;$i
$this->conn[$regs[1]][] = $rs;
$this->_close();
}
function _update($query) {
eregi("update ([0-9a-z_] ) set *(,?.*=.*) ( where (. ))",$query,$regs);
$regs[2] = eregi_replace(",","=",$regs[2]);
$v = split("=",$regs[2]);
$keys = $this->_where($regs[4],"\$this->conn[$regs[1]]");
while(list($key,$value) = each($keys)) {
for($i=0;$i
}
$this->_close();
}
function _delete($query) {
eregi("delete from ([0-9a-z_] ) *(where (. ))?",$query,$regs);
$keys = $this->_where($regs[3],"\$this->conn[$regs[1]]");
while(list($key,$value) = each($keys)) {
unset($this->conn[$regs[1]][$value]);
}
reset($this->conn[$regs[1]]);
while(list($key,$value) = each($this->conn[$regs[1]])) {
$ch[] = $value;
}
$this->conn[$regs[1]] = $ch;
$this->_close();
}
function _where($search,$table) {
$search = eregi_replace("\("," ( ",$search);
$search = eregi_replace("\)"," ) ",$search);
$search = eregi_replace("\ "," ",$search);
$search = eregi_replace("\*"," * ",$search);
while(eregi("[^ ]([*/>
$search = eregi_replace($regs[1]," $regs[1] ",$search);
}
while(eregi("([>
$search = eregi_replace($regs[1],eregi_replace(" ","",$regs[1]),$search);
}
$search = eregi_replace(" "," ",trim($search));
$search = eregi_replace(" and "," && ",$search);
$search = eregi_replace(" or "," || ",$search);
$search = eregi_replace(" = "," == ",$search);
$ar = split(" ",$search);
eval("\$t=$table;");
for($i=0;$i
$ar[$i] = "\$value[".$ar][$i]."]";
}
$expr = "\$expl=(".join(" ",$ar).");";
while(list($key,$value) = each($t)) {
eval($expr);
if($expl)
$keys[] = $key;
}
return $keys;
}
function _sort(&$ar,$key=0,$mode="desc") {
global $cmp_key;
$cmp_key = $key;
if($mode == "asc")
usort($ar,_cmp_asc);
else
usort($ar,_cmp_desc);
}
function _close() {
$fp = fopen($this->database,"w");
fwrite($fp,serialize($this->conn));
fclose($fp);
}
}
/** 排序键
*/
$cmp_key = "";
/** 排序用工作函数(降序 由usort()调用)
*/
function _cmp_desc($a,$b) {
global $cmp_key;
if ($a[$cmp_key] == $b[$cmp_key]) return 0;
return ($a[$cmp_key] > $b[$cmp_key]) ? -1 : 1;
}
/** 排序用工作函数(升序 由usort()调用)
*/
function _cmp_asc($a,$b) {
global $cmp_key;
if ($a[$cmp_key] == $b[$cmp_key]) return 0;
return ($a[$cmp_key] > $b[$cmp_key]) ? 1 : -1;
}
?>
测试例:
<br>
<?php <br>
//require_once "db_text.php";<br>
<br>
$conn = new DB_text;<br>
$conn->connect("text1.txt");<br>
<br>
$conn->query("insert into manage (id,title) values (10,'abcd')");<br>
$conn->query("insert into manage (id,title) values (2,'43d')");<br>
$conn->query("insert into manage (id,title) values (20,'tuu')");<br>
$conn->query("update manage set id=101,test='a' where id=10");<br>
//$conn->query("delete from manage where id='10'");<br>
//$conn->query("delete from manage where id=10 or table='code'");<br>
<br>
<br>
//$rt = $conn->query("select * from manage where id=101 or table='code' group by 1 order by 1 asc");<br>
$rt = $conn->query("select * from manage group by 1 order by id desc");<br>
<br>
print_r($rt);<br>
<br>
?><br>

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
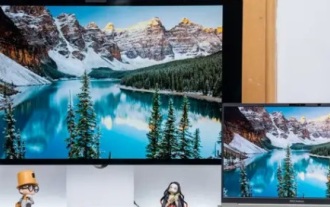
We often see the introduction of how many K screens we have when buying TVs, computers or mobile phones, such as 2.8K screens. At this time, there will be friends who don’t know much about electronic devices and will be curious about what this 2.8K screen means and what the resolution is. What does 2.8k screen mean? Answer: 2.8k screen means that the screen resolution is 2880*18002K, which means the number of horizontal pixels is greater than 2000. For the same size screen, the higher the resolution, the better the picture quality. Introduction to resolution 1. Since the points, lines and surfaces on the screen are all composed of pixels, the more pixels the monitor can display, the finer the picture, and the more information can be displayed in the same screen area. 2. The higher the resolution, the greater the number of pixels, and the sharper the sensed image.
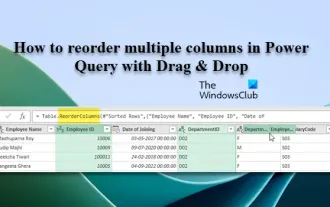
In this article, we will show you how to reorder multiple columns in PowerQuery by dragging and dropping. Often, when importing data from various sources, columns may not be in the desired order. Reordering columns not only allows you to arrange them in a logical order that suits your analysis or reporting needs, it also improves the readability of your data and speeds up tasks such as filtering, sorting, and performing calculations. How to rearrange multiple columns in Excel? There are many ways to rearrange columns in Excel. You can simply select the column header and drag it to the desired location. However, this approach can become cumbersome when dealing with large tables with many columns. To rearrange columns more efficiently, you can use the enhanced query editor. Enhancing the query
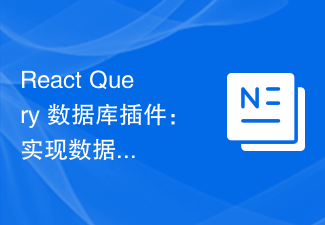
ReactQuery database plug-in: Methods to implement data import and export, specific code examples are required. With the widespread application of ReactQuery in front-end development, more and more developers are beginning to use it to manage data. In actual development, we often need to export data to local files or import data from local files into the database. In order to implement these functions more conveniently, you can use the ReactQuery database plug-in. The ReactQuery database plugin provides a series of methods
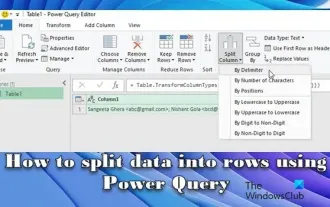
This article will introduce how to use PowerQuery to split data into rows. When exporting data from other systems or sources, it is common to encounter situations where the data is stored in cells combining multiple values. With PowerQuery, we can easily split such data into rows, making the data easier to process and analyze. This can happen if the user doesn't understand Excel's rules and accidentally enters multiple data into a cell, or if the data is not formatted correctly when copying/pasting it from other sources. Processing this data requires additional steps to extract and organize the information for analysis or reporting. How to split data in PowerQuery? PowerQuery transformations can be based on a variety of different factors such as word
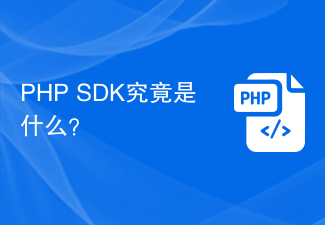
PHPSDK is a software development toolkit used to assist developers to quickly and easily integrate third-party services or API interfaces in the PHP language. The full name of SDK is Software Development Kit, which is software development kit. It provides a series of functions, classes, methods and tools to make it easier for developers to interact with external services. In PHP development, SDK usually contains encapsulation of specific services to simplify the process of developers writing related code. PHPSD
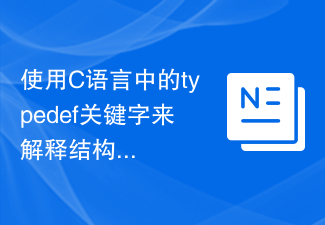
Typedef 'C' allows new data type names to be defined using the 'typedef' keyword. Using ‘typedef’ we cannot create a new data type, but define a new name for an already existing type. The Chinese translation of Syntaxtypedefdatatypenewname;Example is: example typedefintbhanu;inta;bhanua;%dThisstatementtellsthecompilertorec
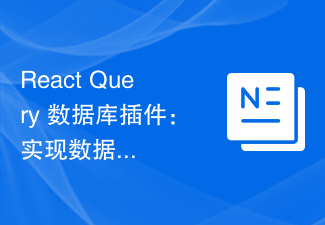
ReactQuery database plug-in: Strategies to implement data backup and restoration, specific code examples are required Introduction: In modern web development, data backup and restoration is a very important task. Especially when using state management tools like ReactQuery, we need to ensure data security and reliability. This article will introduce a database plug-in based on ReactQuery to implement data backup and restore strategies, and provide specific code examples. ReactQu
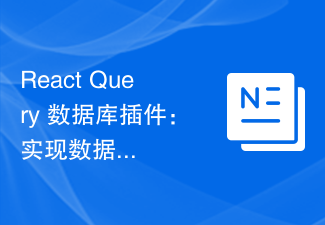
ReactQuery database plugin: Tips for implementing data compression and decompression, specific code examples required Introduction: In modern web application development, processing large amounts of data queries is a common task. ReactQuery is a powerful library that provides a simple and intuitive way to manage data queries and state. Although ReactQuery itself is already very good, when dealing with large amounts of data, we may need to consider some additional tricks to improve performance and optimize storage space. This article will introduce
