Summary of PHP image cropping techniques
Summary of PHP image cropping techniques, specific code examples are required
In web development, the need to crop images is often involved. Whether it is to adapt to different layout needs or to improve page loading speed, image cropping is a very important technology. As a popular server-side scripting language, PHP provides a wealth of image processing functions and libraries, making image cropping easier and more efficient. This article will introduce some commonly used PHP image cropping techniques and provide specific code examples.
1. GD library to crop images
The GD library is an image processing library provided by PHP, which can crop, scale, rotate and other operations on images. The following is a simple example that demonstrates how to use the GD library to crop an image:
<?php // 设置原图片路径和目标图片路径 $src = 'original.jpg'; $dst = 'cropped.jpg'; // 获取原图片和目标图片的宽高 list($srcWidth, $srcHeight) = getimagesize($src); $dstWidth = 300; $dstHeight = 300; // 创建一个指定大小的目标图片 $dstImage = imagecreatetruecolor($dstWidth, $dstHeight); // 打开原图片 $srcImage = imagecreatefromjpeg($src); // 裁剪原图片到目标图片 imagecopyresampled($dstImage, $srcImage, 0, 0, 0, 0, $dstWidth, $dstHeight, $srcWidth, $srcHeight); // 保存目标图片 imagejpeg($dstImage, $dst); // 释放资源 imagedestroy($dstImage); imagedestroy($srcImage); ?>
The above code first uses the getimagesize
function to obtain the width and height of the original image, and then uses imagecreatetruecolor
The function creates a target image of the specified size. Then, use the imagecreatefromjpeg
function to open the original image, and use the imagecopyresampled
function to crop the original image into the target image. Finally, use the imagejpeg
function to save the target image, and use the imagedestroy
function to release the resources.
2. ImageMagick cropping pictures
ImageMagick is a powerful open source image processing software suite, and PHP provides extensions for interacting with ImageMagick. The following example demonstrates how to use ImageMagick to crop an image:
<?php // 设置原图片路径和目标图片路径 $src = 'original.jpg'; $dst = 'cropped.jpg'; // 初始化ImageMagick对象 $imagick = new Imagick($src); // 获取原图片的宽高 $srcWidth = $imagick->getImageWidth(); $srcHeight = $imagick->getImageHeight(); // 设置裁剪参数 $x = 0; $y = 0; $dstWidth = 300; $dstHeight = 300; // 裁剪图片 $imagick->cropImage($dstWidth, $dstHeight, $x, $y); // 保存目标图片 $imagick->writeImage($dst); // 释放资源 $imagick->destroy(); ?>
The above code first initializes an ImageMagick object using the Imagick
class, and uses getImageWidth
and getImageHeight
method obtains the width and height of the original image. Then, use the cropImage
method to crop the image and set the cropping parameters. Finally, use the writeImage
method to save the target image, and use the destroy
method to release the resources.
3. Use the third-party library Intervention Image
Intervention Image is a powerful and easy-to-use PHP image processing library that provides many fast image processing methods, including image cropping. The following example demonstrates how to use Intervention Image to crop an image:
<?php // 引入Intervention Image库 require 'vendor/autoload.php'; // 使用ImageManager创建Intervention Image对象 $img = InterventionImageImageManagerStatic::make('original.jpg'); // 设置裁剪参数 $x = 0; $y = 0; $dstWidth = 300; $dstHeight = 300; // 裁剪图片 $img->crop($dstWidth, $dstHeight, $x, $y); // 保存目标图片 $img->save('cropped.jpg'); ?>
The above code first uses the ImageManagerStatic::make
method to create an Intervention Image object and calls crop through the chain
Method to crop the image and set the cropping parameters. Finally, use the save
method to save the target image.
To sum up, the above are specific code examples of several commonly used PHP image cropping techniques. By using the GD library, ImageMagick or the third-party library Intervention Image, we can easily achieve image cropping under various needs. No matter which method you use, as long as you set the cropping parameters according to your needs, you can get the cropping effect that meets your requirements.
The above is the detailed content of Summary of PHP image cropping techniques. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


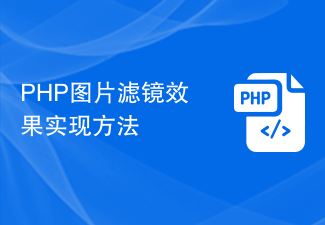
How to implement PHP image filter effects requires specific code examples. Introduction: In the process of web development, image filter effects are often used to enhance the vividness and visual effects of images. The PHP language provides a series of functions and methods to achieve various picture filter effects. This article will introduce some commonly used picture filter effects and their implementation methods, and provide specific code examples. 1. Brightness adjustment Brightness adjustment is a common picture filter effect, which can change the lightness and darkness of the picture. By using imagefilte in PHP

PHP is a programming language widely used in web development. It is highly readable and easy to learn. It also has high application value in the field of image processing. From PHP5.5 to PHP7.0 upgrade, PHP has made a series of optimizations and improvements in image processing, including more efficient memory management, faster execution speed, richer image processing functions, etc. This article will introduce in detail how to perform image processing in PHP7.0. 1. GD library image processing is an essential part of web development.
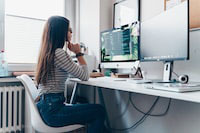
This article will explain in detail how to draw an ellipse in PHP. The editor thinks it is quite practical, so I share it with you as a reference. I hope you can gain something after reading this article. PHP Drawing Ellipses Preface The PHP language provides a rich function library, among which the GD library is specially used for image processing and can draw various shapes in PHP, including ellipses. Draw an ellipse 1. Load the GD library 2. Create an image
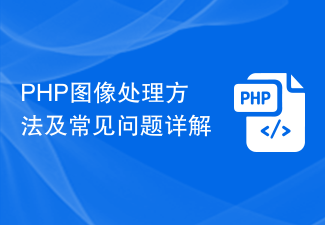
PHP is a very popular server-side scripting language that can handle a wide variety of web tasks, including image processing. This article will introduce some image processing methods in PHP and some common problems you may encounter. 1. How to process images in PHP 1. Use the GD library GD (GNU Image Processing Library) is an open source library for image processing. It allows PHP developers to create and manipulate images using scripts, including scaling, cropping, rotating, filtering, and drawing. Before using the GD library, you need to make sure
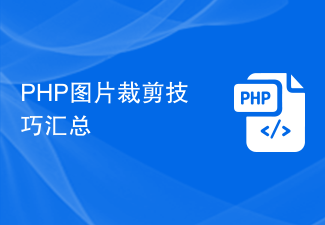
Summary of PHP image cropping techniques, specific code examples are required. In web development, the need to crop images is often involved. Whether it is to adapt to different layout needs or to improve page loading speed, image cropping is a very important technology. As a popular server-side scripting language, PHP provides a wealth of image processing functions and libraries, making image cropping easier and more efficient. This article will introduce some commonly used PHP image cropping techniques and provide specific code examples. 1. GD library to crop pictures GD
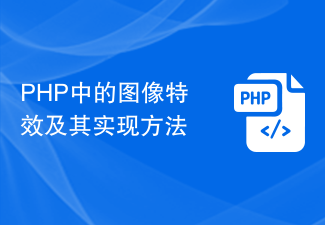
In website development, image special effects can increase the beauty of the page, attract users' attention, and provide users with a better experience. As a powerful back-end language, PHP also provides many methods to achieve image special effects. This article will introduce commonly used image effects in PHP and their implementation methods. Scaling images Scaling images is one of the common ways to implement responsive design on your website. The imagecopyresampled() function is provided in PHP to complete the operation of scaling images. The prototype of this function is as follows: boolim
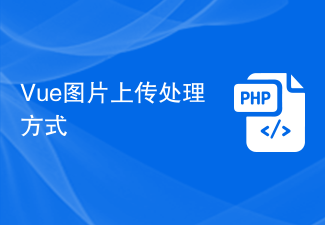
How to handle image uploading in Vue development. With the popularity of the Internet and the development of technology, image uploading has become one of the common functions in many websites and applications. For Vue developers, how to efficiently handle image uploading is an important skill. In this article, we will discuss how to handle image uploading in Vue development, and introduce some practical methods and techniques. First, let's look at the basic steps for handling image uploads in Vue. First, we need to add a file upload function to the web page

Image processing is a very important topic in PHP programming. With the development of web applications, more and more websites need to use images to attract users' attention. Therefore, it is very important for PHP developers to master some common image processing operations. This article will introduce some common image processing operations for reference by PHP developers. 1. Image scaling Image scaling is one of the most common operations in image processing. PHP provides two methods to resize images: ImageCopyResample()
