


Analysis of advanced application methods of PHP closures, generators and reflection technology
Advanced application method analysis of PHP closure, generator and reflection technology
Overview:
In PHP programming, closure, generator and reflection are powerful features and technologies. They provide many advanced and flexible features to help us organize and manage our code better. This article will analyze the advanced application methods of these three technologies and provide specific code examples.
1. Advanced application methods of closures:
1.1 Use closures to implement "lazy evaluation":
Closures can be used to implement "lazy evaluation" The idea is to defer calculations and only perform calculations when needed. The following example shows how to use closures to implement lazy loading.
function lazySum($a, $b) { return function() use ($a, $b) { return $a + $b; }; } $sum = lazySum(3, 4); echo $sum(); // 输出7
In the above example, the lazySum function returns a closure instead of returning the calculation result directly. The result is actually calculated only when the closure is called. In this way, we can implement a simple lazy loading function.
1.2 Use closures to implement Currying:
Closures can also be used to implement Currying, that is, converting a multi-parameter function into multiple single-parameter functions. The following example shows how to use closures to implement currying.
function add($a) { return function($b) use ($a) { return $a + $b; }; } $addTwo = add(2); echo $addTwo(3); // 输出5
In the above example, the add function receives a parameter $a and returns a closure. The closure receives the parameter $b and adds $a and $b. In this way, we can use multiple function calls to implement a complex calculation process.
2. Advanced application methods of generators:
2.1 Use generators to achieve (infinite) sequences:
Generators are very suitable for generating (infinite) sequences. Rather than having to generate all elements at once. The following example shows how to use a generator to implement an infinite sequence.
function infiniteSequence() { $n = 0; while (true) { yield $n; $n++; } } $sequence = infiniteSequence(); foreach ($sequence as $number) { echo $number . " "; // 输出0 1 2 3 4 ... if ($number > 10) { break; } }
In the above example, the infiniteSequence function is a generator function. It uses the yield keyword to generate a sequence. By generating one element at a time and continuing to generate elements on the next iteration, an infinite sequence is generated.
2.2 Use generators to implement lazy reading:
Generators can also be used to implement lazy reading, that is, reading and processing a portion of the data at a time. The following example shows how to use generators to implement lazy reading.
function processFile($filename) { $file = fopen($filename, 'r'); while ($line = fgets($file)) { yield trim($line); } fclose($file); } $fileData = processFile('data.txt'); foreach ($fileData as $line) { echo $line . PHP_EOL; }
In the above example, the processFile function is a generator function. It reads the file line by line and produces the contents of each line using the yield keyword. This way we can process large files line by line and avoid reading the entire file at once.
3. Advanced application methods of Reflection:
3.1 Dynamically calling class methods:
Reflection can be used to dynamically call class methods, that is, based on the method name at runtime. Call the corresponding method. The following example shows how to use reflection to dynamically call class methods.
class Calculator { public function add($a, $b) { return $a + $b; } } $calculator = new Calculator(); $reflection = new ReflectionMethod('Calculator', 'add'); $result = $reflection->invokeArgs($calculator, [3, 4]); echo $result; // 输出7
In the above example, we use the reflection class ReflectionMethod to get the class method add, and use the invokeArgs method to call the method. In this way we can dynamically call class methods at runtime.
3.2 Dynamically create objects:
Reflection can also be used to dynamically create objects, that is, instantiate the class according to the class name at runtime. The following example shows how to use reflection to dynamically create objects.
class Person { public function __construct($name) { $this->name = $name; } public function sayHello() { echo "Hello, " . $this->name . "!"; } } $reflection = new ReflectionClass('Person'); $person = $reflection->newInstanceArgs(['John']); $person->sayHello(); // 输出Hello, John!
In the above example, we use the reflection class ReflectionClass to get the class and use the newInstanceArgs method to instantiate the class. This way we can create objects dynamically at runtime.
Conclusion:
This article introduces the advanced application methods of PHP closures, generators and reflection technology, and provides specific code examples. Closures can be used to implement "lazy evaluation" and Currying; generators can be used to implement (infinite) sequences and lazy reading; reflection can be used to dynamically call class methods and dynamically create objects. These technologies provide us with more flexibility and functional scalability, helping us better organize and manage code.
The above is the detailed content of Analysis of advanced application methods of PHP closures, generators and reflection technology. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


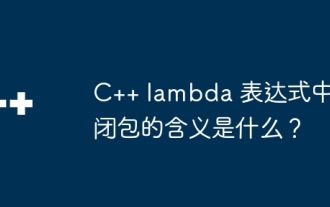
In C++, a closure is a lambda expression that can access external variables. To create a closure, capture the outer variable in the lambda expression. Closures provide advantages such as reusability, information hiding, and delayed evaluation. They are useful in real-world situations such as event handlers, where the closure can still access the outer variables even if they are destroyed.
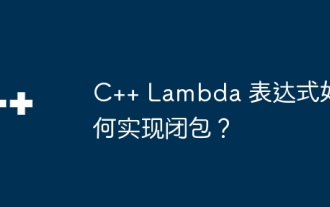
C++ Lambda expressions support closures, which save function scope variables and make them accessible to functions. The syntax is [capture-list](parameters)->return-type{function-body}. capture-list defines the variables to capture. You can use [=] to capture all local variables by value, [&] to capture all local variables by reference, or [variable1, variable2,...] to capture specific variables. Lambda expressions can only access captured variables but cannot modify the original value.
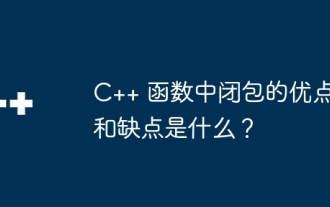
A closure is a nested function that can access variables in the scope of the outer function. Its advantages include data encapsulation, state retention, and flexibility. Disadvantages include memory consumption, performance impact, and debugging complexity. Additionally, closures can create anonymous functions and pass them to other functions as callbacks or arguments.
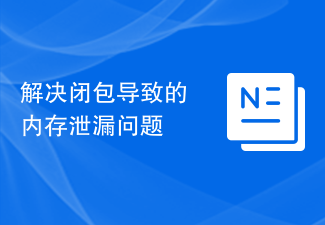
Title: Memory leaks caused by closures and solutions Introduction: Closures are a very common concept in JavaScript, which allow internal functions to access variables of external functions. However, closures can cause memory leaks if used incorrectly. This article will explore the memory leak problem caused by closures and provide solutions and specific code examples. 1. Memory leaks caused by closures The characteristic of closures is that internal functions can access variables of external functions, which means that variables referenced in closures will not be garbage collected. If used improperly,
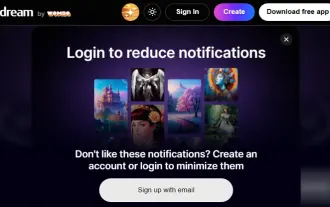
If you are eager to find the top free AI animation art generator, you can end your search. The world of anime art has been captivating audiences for decades with its unique character designs, captivating colors and captivating plots. However, creating anime art requires talent, skill, and a lot of time. However, with the continuous development of artificial intelligence (AI), you can now explore the world of animation art without having to delve into complex technologies with the help of the best free AI animation art generator. This will open up new possibilities for you to unleash your creativity. What is an AI anime art generator? The AI Animation Art Generator utilizes sophisticated algorithms and machine learning techniques to analyze an extensive database of animation works. Through these algorithms, the system learns and identifies different animation styles
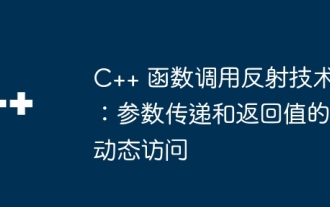
C++ function call reflection technology allows dynamically obtaining function parameter and return value information at runtime. Use typeid(decltype(...)) and decltype(...) expressions to obtain parameter and return value type information. Through reflection, we can dynamically call functions and select specific functions based on runtime input, enabling flexible and scalable code.
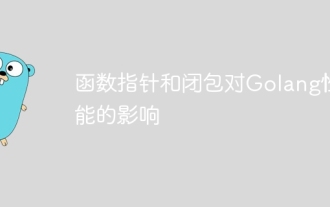
The impact of function pointers and closures on Go performance is as follows: Function pointers: Slightly slower than direct calls, but improves readability and reusability. Closures: Typically slower, but encapsulate data and behavior. Practical case: Function pointers can optimize sorting algorithms, and closures can create event handlers, but they will bring performance losses.
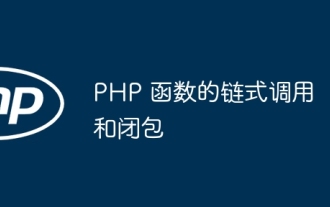
Yes, code simplicity and readability can be optimized through chained calls and closures: chained calls link function calls into a fluent interface. Closures create reusable blocks of code and access variables outside functions.
