How to implement push messages using Slack Webhooks in PHP
How to use Slack Webhooks to implement message push in PHP
Introduction:
Slack is a tool widely used for team collaboration, and Slack Webhooks is Slack An API provided that can push messages to the Slack channel through HTTP requests. This article will introduce how to use Slack Webhooks to implement message push in PHP, and give specific code examples.
Step 1: Get the Slack Webhook URL
First, you need to create a Webhook on Slack to receive your push messages. In Slack, open the channel you want to push messages to, find "Add apps and integrations" in "Settings", then search for "Webhooks" and add a new webhook. When you create a webhook, you will be given a unique URL that you need to push messages.
Step 2: Send a message to Slack
In PHP, you can use the cURL library to send HTTP requests. To send messages to Slack, you need to use the curl_init()
, curl_setopt()
, and curl_exec()
functions of the cURL library.
Here is a basic PHP code example showing how to send a message to Slack:
<?php // 设置Slack Webhook URL $webhookUrl = 'https://hooks.slack.com/services/your-webhook-url'; // 准备要发送的消息内容 $message = array( 'text' => '这是一条来自PHP的Slack消息', ); // 将消息内容转化为JSON格式 $jsonPayload = json_encode($message); // 设置cURL请求 $ch = curl_init($webhookUrl); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST"); curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonPayload); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); // 发送请求并获取返回结果 $result = curl_exec($ch); // 检查请求是否成功 if ($result === false) { echo '发送消息到Slack失败: ' . curl_error($ch); } else { echo '消息已成功发送到Slack'; } // 关闭cURL资源 curl_close($ch); ?>
Please note that you need to change the in the
$webhookUrl variable Replace your-webhook-url
with the webhook URL you created on Slack.
In this code example, we first prepare the message content to be sent and convert it into JSON format. We then set up a POST request using the cURL library to send the JSON data as the request body to the Slack webhook URL. Finally, we send the request through curl_exec()
and get the return result.
Summary:
The process of pushing messages to Slack in PHP is relatively simple. By using Slack Webhooks, you can easily send messages to Slack channels for instant communication and collaboration with your team. I hope this article can help you understand and implement the message push function using Slack Webhooks in PHP.
The above is the detailed content of How to implement push messages using Slack Webhooks in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


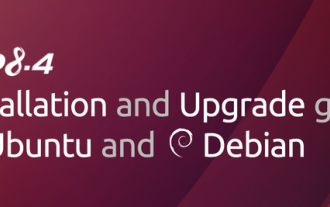
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
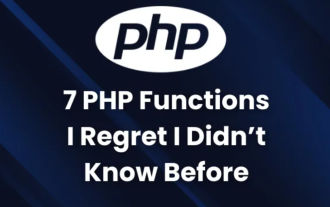
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
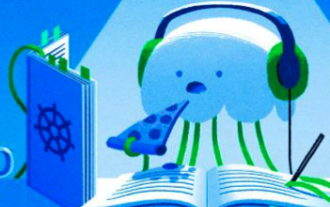
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
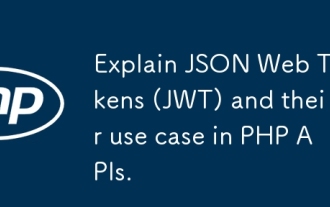
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
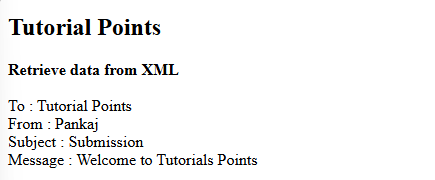
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
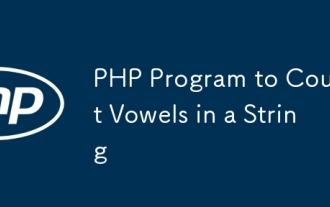
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
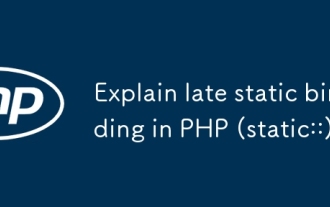
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
