


php Elasticsearch: How to handle load balancing of search requests?
php Elasticsearch: How to handle load balancing of search requests?
- Introduction
Load balancing is an important step in handling high concurrent search requests. When using Elasticsearch for search, failure to adopt appropriate load balancing strategies may result in reduced search performance or system crashes. This article will introduce how to use PHP and Elasticsearch to implement load balancing of search requests, and demonstrate the specific implementation process through sample code.
- Elasticsearch load balancing strategy
Elasticsearch provides a variety of load balancing strategies, and we can choose the appropriate strategy according to actual needs. The following are common load balancing strategies:
- Round Robin: Distribute requests to each node in order, suitable for situations where the number of nodes is close to equal.
- Least Connection: Distribute requests to the nodes with the fewest connections, suitable for situations where node load is uneven.
- IP Hash: Calculate the hash value based on the client’s IP address and send the request to the corresponding node. Ensure that each client request is sent to the same node, suitable for stateful search requests.
- Weighted Round Robin: Distribute requests according to the weight of the node. Nodes with higher weights will receive more requests, which is suitable for situations where node performance is unbalanced.
- Use PHP to implement Elasticsearch load balancing
First, make sure that the PHP Elasticsearch extension has been installed and enabled. Next, we will use the official client library provided by Elasticsearch, which provides load balancing functionality.
Suppose we have three Elasticsearch nodes, namely "http://node1:9200", "http://node2:9200" and "http://node3:9200". The following is a sample code using the load balancing strategy:
<?php require 'vendor/autoload.php'; use ElasticsearchClientBuilder; $nodes = [ 'http://node1:9200', 'http://node2:9200', 'http://node3:9200' ]; $client = ClientBuilder::create() ->setHosts($nodes) ->build(); $params = [ 'index' => 'my_index', 'type' => 'my_type', 'body' => [ 'query' => [ 'match' => [ 'title' => 'Elasticsearch' ] ] ] ]; $response = $client->search($params); print_r($response); ?>
In the above code, we create an Elasticsearch client through ClientBuilder
and set the node list. Search requests will be sent to different nodes according to the load balancing policy.
- Advanced configuration
In addition to simple load balancing strategies, we can also perform some advanced configurations to meet special needs. For example:
- Custom node weight: By specifying a weight for each node in the node list, the load balancing among nodes can be dynamically adjusted based on performance.
- Health check: Regularly check the health status of each node. If the node is abnormal, the request will be forwarded to other healthy nodes.
- Slow query log: Record the slow query log for subsequent analysis and optimization.
These advanced configurations are beyond the scope of this article. Interested readers can consult the Elasticsearch official documentation or other related materials.
- Summary
By using PHP and Elasticsearch, we can easily achieve load balancing of search requests. Choosing an appropriate load balancing strategy, combined with advanced configuration, can further improve search performance and system stability. I hope this article can help you understand and solve Elasticsearch load balancing problems.
Reference:
- Elasticsearch Documentation: https://www.elastic.co/guide/en/elasticsearch/reference/current/index.html
The above is an article about how to handle the load balancing problem of search requests. I hope it will be helpful to you.
The above is the detailed content of php Elasticsearch: How to handle load balancing of search requests?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


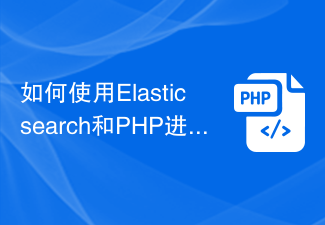
How to use Elasticsearch and PHP for product search and recommendation Introduction: In today's e-commerce field, a good search and recommendation system is very important for users. Elasticsearch is a powerful and flexible open source search engine. Combined with PHP as a back-end development language, it can provide efficient product search and personalized recommendation functions for e-commerce websites. This article will introduce how to use Elasticsearch and PHP to implement product search and recommendation functions, and attach
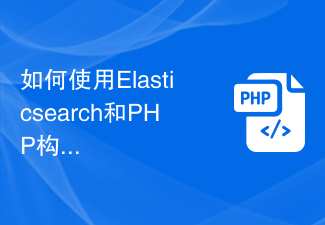
How to use Elasticsearch and PHP to build a user login and permission management system Introduction: In the current Internet era, user login and permission management are one of the necessary functions for every website or application. Elasticsearch is a powerful and flexible full-text search engine, while PHP is a widely used server-side scripting language. This article will introduce how to combine Elasticsearch and PHP to build a simple user login and permission management system
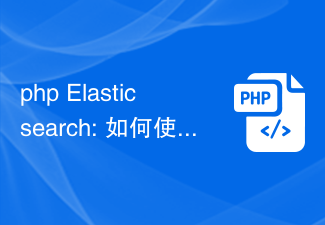
PHPElasticsearch: How to use dynamic mapping to achieve flexible search capabilities? Introduction: Search functionality is an integral part of developing modern applications. Elasticsearch is a powerful search and analysis engine that provides rich functionality and flexible data modeling. In this article, we will focus on how to use dynamic mapping to achieve flexible search capabilities. 1. Introduction to dynamic mapping In Elasticsearch, mapping (mapp
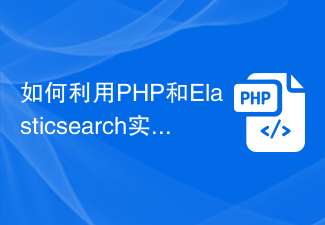
How to use PHP and Elasticsearch to achieve highlighted search results Introduction: In the modern Internet world, search engines have become the main way for people to obtain information. In order to improve the readability and user experience of search results, highlighting search keywords has become a common requirement. This article will introduce how to use PHP and Elasticsearch to achieve highlighted search results. 1. Preparation Before starting, we need to ensure that PHP and Elasticsearch have been installed and configured correctly.
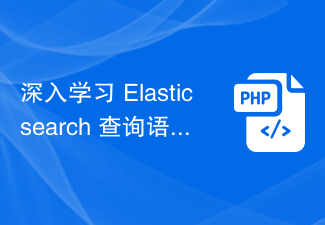
In-depth study of Elasticsearch query syntax and practical introduction: Elasticsearch is an open source search engine based on Lucene. It is mainly used for distributed search and analysis. It is widely used in full-text search of large-scale data, log analysis, recommendation systems and other scenarios. When using Elasticsearch for data query, flexible use of query syntax is the key to improving query efficiency. This article will delve into the Elasticsearch query syntax and give it based on actual cases.
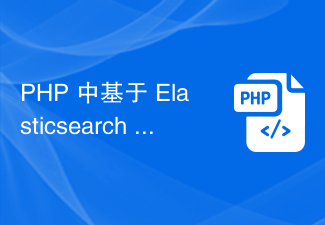
Summary of log analysis and exception monitoring based on Elasticsearch in PHP: This article will introduce how to use the Elasticsearch database for log analysis and exception monitoring. Through concise PHP code examples, it shows how to connect to the Elasticsearch database, write log data to the database, and use Elasticsearch's powerful query function to analyze and monitor anomalies in the logs. Introduction: Log analysis and exception monitoring are
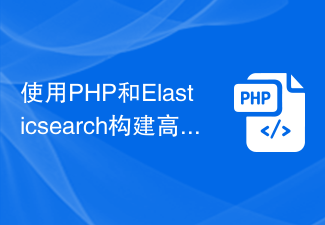
Use PHP and Elasticsearch to build an efficient search engine Introduction: In today's Internet era, search engines are people's first choice for obtaining information. In order to provide fast and accurate search results, developers need to build efficient search engines. This article will introduce how to use PHP and Elasticsearch to build an efficient search engine, and give corresponding code examples. 1. What is Elasticsearch? Elasticsearch is a distributed open source search and analytics
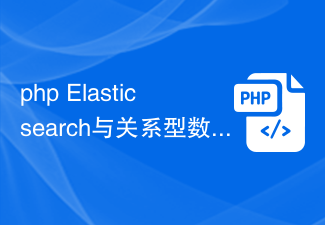
Introduction to the Practical Guide for the Integration of PHPElasticsearch and Relational Databases: With the advent of the Internet and big data era, data storage and processing methods are also constantly evolving. Traditional relational databases have gradually shown some shortcomings when faced with scenarios such as massive data, high concurrent reading and writing, and full-text search. As a real-time distributed search and analysis engine, Elasticsearch has gradually attracted the attention and use of the industry through its high-performance full-text search, real-time analysis and data visualization functions. Ran
