


How to use php Elasticsearch to implement intelligent recommendation function?
How to use PHP Elasticsearch to implement intelligent recommendation function?
Smart recommendations are one of the common and important features in modern applications. It can automatically recommend relevant content or products based on user preferences, behavior and historical data to improve user experience and increase interactivity. In this article, we will explore how to use PHP Elasticsearch to implement intelligent recommendation functions and provide specific code examples.
- Installing and configuring Elasticsearch
First, we need to install and configure Elasticsearch in the local environment. You can download the latest stable version from the official website of Elasticsearch and install and configure it according to the guidelines of the official documentation. After the installation is complete, ensure that Elasticsearch is running successfully and can be accessed at http://localhost:9200.
- Creating indexes and mappings
Before we start writing code, we need to create an index and define the corresponding mapping. In this example, assuming we want to implement a product recommendation function, we can create an index named "products". The following is sample code for creating indexes and mappings:
PUT /products { "mappings": { "properties": { "title": { "type": "text" }, "category": { "type": "keyword" }, "tags": { "type": "keyword" }, "price": { "type": "float" } } } }
According to actual needs, you can adjust the field types and attributes in the mapping.
- Add data to the index
In actual use, we need to add product data to the index so that Elasticsearch can search and recommend. The following is a sample code for adding data:
require 'vendor/autoload.php'; use ElasticsearchClientBuilder; $client = ClientBuilder::create()->build(); $params = [ 'index' => 'products', 'body' => [ ['index' => ['_index' => 'products']], ['title' => 'Product 1', 'category' => 'Category 1', 'tags' => ['tag1', 'tag2'], 'price' => 10.99], ['index' => ['_index' => 'products']], ['title' => 'Product 2', 'category' => 'Category 2', 'tags' => ['tag3', 'tag4'], 'price' => 20.99], // 添加更多商品数据... ] ]; $response = $client->bulk($params); // 检查添加是否成功 if ($response['errors']) { foreach($response['items'] as $item) { if ($item['index']['status'] !== 201) { echo "Failed to add product: " . $item['index']['error']['reason']; } } } else { echo "Products added successfully."; }
In the above sample code, we use the PHP client library (Elasticsearch-PHP) provided by Elasticsearch to interact with Elasticsearch. First, we create an Elasticsearch client instance using ClientBuilder
. Then, we add the product data to the index in batches through the bulk
method.
- Implementing the Intelligent Recommendation Algorithm
Once the data is successfully added to the index, we can start implementing the intelligent recommendation algorithm.
First, we need to determine the product categories, tags or other attributes that the target user of the collection (or the current user) is interested in. We can then use Elasticsearch's query capabilities to search for and return related items. Here is a sample code snippet for searching for items that match a user’s tags:
$params = [ 'index' => 'products', 'body' => [ 'query' => [ 'terms' => [ 'tags' => ['user_tag_1', 'user_tag_2'] ] ] ] ]; $response = $client->search($params); // 处理搜索结果 if ($response['hits']['total']['value'] > 0) { foreach ($response['hits']['hits'] as $hit) { echo $hit['_source']['title'] . ', ' . $hit['_source']['price'] . PHP_EOL; } } else { echo "No products found."; }
In the above sample code, we use Elasticsearch’s terms
query to search for items that match a user’s tags . $params
The array specifies the search conditions and index name. We use the search
method to perform the search and process the returned results.
According to the actual needs of users, you can use more complex query conditions, such as multi-field matching, range query, etc. Elasticsearch provides rich query syntax and functions that can be adjusted according to actual needs.
- Full Example
The following is a complete example that shows how to use PHP Elasticsearch to implement intelligent recommendation functionality:
require 'vendor/autoload.php'; use ElasticsearchClientBuilder; $client = ClientBuilder::create()->build(); // 创建索引和映射 $params = [ 'index' => 'products', 'body' => [ "mappings" => [ "properties" => [ "title" => [ "type" => "text" ], "category" => [ "type" => "keyword" ], "tags" => [ "type" => "keyword" ], "price" => [ "type" => "float" ] ] ] ] ]; $client->indices()->create($params); // 添加数据到索引 $params = [ 'index' => 'products', 'body' => [ ['index' => ['_index' => 'products']], ['title' => 'Product 1', 'category' => 'Category 1', 'tags' => ['tag1', 'tag2'], 'price' => 10.99], ['index' => ['_index' => 'products']], ['title' => 'Product 2', 'category' => 'Category 2', 'tags' => ['tag3', 'tag4'], 'price' => 20.99], // 添加更多商品数据... ] ]; $client->bulk($params); // 执行智能推荐算法 $params = [ 'index' => 'products', 'body' => [ 'query' => [ 'terms' => [ 'tags' => ['user_tag_1', 'user_tag_2'] ] ] ] ]; $response = $client->search($params); // 处理搜索结果 if ($response['hits']['total']['value'] > 0) { foreach ($response['hits']['hits'] as $hit) { echo $hit['_source']['title'] . ', ' . $hit['_source']['price'] . PHP_EOL; } } else { echo "No products found."; }
In the above example, We first created an index called "products" and defined the corresponding mapping. Then we added some sample product data to the index. Finally, we implement an intelligent recommendation algorithm to search and return relevant products based on user tags.
Please adjust the code according to actual needs, and perform more detailed configuration and tuning according to the instructions in the document. I hope this article will help you understand how to use PHP Elasticsearch to implement intelligent recommendation functions!
The above is the detailed content of How to use php Elasticsearch to implement intelligent recommendation function?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


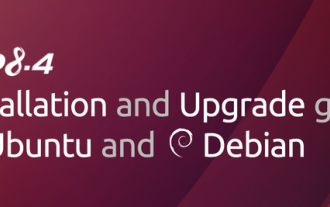
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
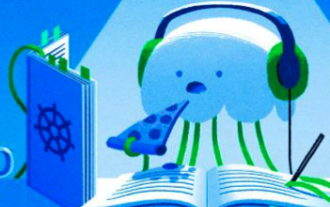
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
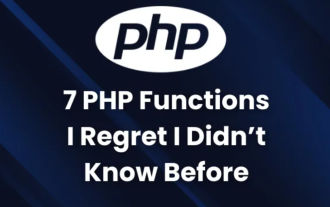
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
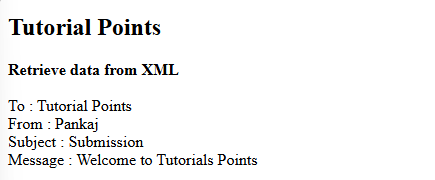
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
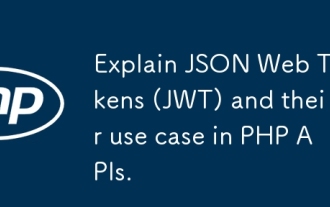
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
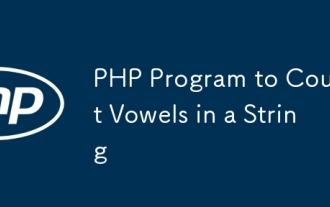
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
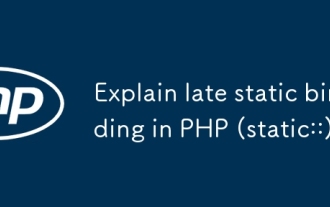
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
