Rearrange an array so that arr = i, using C++
We have an array of positive integer type, assuming it is arr[], its size can be given arbitrarily, and the elements in the array should be greater than 0 but less than the size of the array. The task is to rearrange the array such that if arr[i] is equal to 'i', then 'i' exists in the array, otherwise set the arr[i] element to -1 and print the final result.
Let us take a look at various input and output scenarios for this problem:
Input − int arr[] = {0, 8, 1, 5, 4, 3 , 2, 9 }
Output − The rearranged array is: 0 1 2 3 4 5 -1 -1
Explanation − We have an integer array of size 8, all elements in the array are less than 8. Now, we will rearrange the array i.e.
arr[0] = 0(present in an array) arr[1] = 1(present in an array) arr[2] = 2(present in an array) arr[3] = 3(present in an array) arr[4] = 4(present in an array) arr[5] = 5(present in an array) arr[6] = -1(NOT present in an array) arr[7] = -1(NOT present in an array)
input− int arr[] = {1, 2, 6, 9, 10}
output − Rearrange the array so that arr[i] = i is: -1 1 2 -1 -1
Explanation− We get an integer array of size 5, and All elements in the array have values less than or greater than 5. Now, we will rearrange the array i.e.
arr[0] = -1(NOT present in an array) arr[1] = 1(present in an array) arr[2] = 2(present in an array) arr[3] = -1(NOT present in an array) arr[4] = -1(NOT present in an array)
The method used in the program below is as follows:
Enter an array of integer type and calculate the size of the array.
Print the array before sorting and call the function Rearranging(arr, size)
Inside the function Rearranging(arr, size)
Declare an integer type variable, assuming it is ptr
Start looping from i to 0 until i is less than size. Inside the loop, another loop starts from j to 0 until j is less than size.
Inside the loop, check if arr[j] = i, then set ptr = arr[j], arr[j] = arr[i], arr[i] = ptr And break out of the loop.
Start looping from i to size. Inside the loop, check if arr[i]! = i, then set arr[i] to -1.
#Print an array after rearranging the array values.
Example
#include <iostream> using namespace std; void Rearranging(int arr[], int size){ int ptr; for(int i = 0; i < size; i++){ for(int j = 0; j < size; j++){ if(arr[j] == i){ ptr = arr[j]; arr[j] = arr[i]; arr[i] = ptr; break; } } } for(int i = 0; i < size; i++){ if(arr[i] != i){ arr[i] = -1; } } } int main(){ int arr[] = {0, 8, 1, 5, 4, 3, 2, 9 }; int size = sizeof(arr) / sizeof(arr[0]); //calling the function to rearrange an array such that arr[i] = i Rearranging(arr, size); //Printing the array cout<<"Rearrangement of an array such that arr[i] = i is: "; for(int i = 0; i < size; i++){ cout << arr[i] << " "; } }
Output
If we run the above code it will generate the following output
Rearrangement of an array such that arr[i] = i is: 0 1 2 3 4 5 -1 -1
The above is the detailed content of Rearrange an array so that arr = i, using C++. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
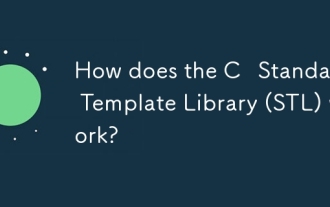
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
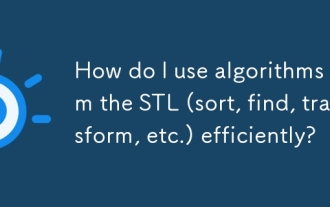
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
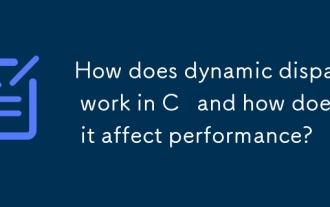
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
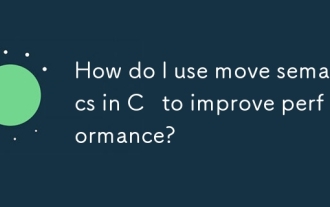
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
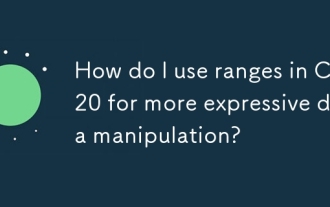
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
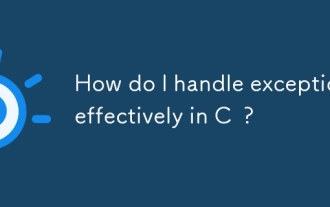
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
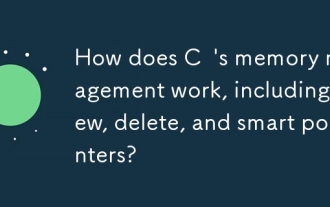
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
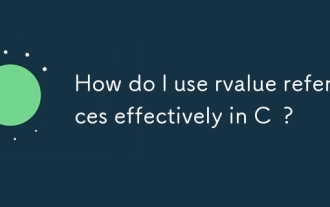
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
