How to use array variables in PHP
How to use array variables in PHP
In PHP, an array is a very commonly used data structure that can be used to store and operate multiple values. This article will explain how to use array variables in PHP and provide some concrete code examples.
- Creating array variables
In PHP, you can create array variables in two ways: using the array() function or directly declaring an empty array. Here are two sample codes for creating array variables:
// 使用array()函数创建数组 $fruits = array("apple", "banana", "orange"); // 直接声明一个空数组 $numbers = [];
- Accessing Array Elements
You can access array elements using the index of the array. First of all, you need to understand that PHP's array index starts at 0 by default and can be an integer or a string. Here are some sample codes:
// 访问数组元素 echo $fruits[0]; // 输出 "apple" echo $fruits[1]; // 输出 "banana" echo $fruits[2]; // 输出 "orange" // 修改数组元素的值 $fruits[1] = "grape"; echo $fruits[1]; // 输出 "grape"
- Determine whether an element exists in the array
You can use the array_key_exists() function or in_array() function to determine whether an element exists in the array. The following are examples of usage of these two functions:
// 使用array_key_exists()函数判断 $fruits = array("apple", "banana", "orange"); if (array_key_exists(2, $fruits)) { echo "存在"; } else { echo "不存在"; } // 使用in_array()函数判断 if (in_array("apple", $fruits)) { echo "存在"; } else { echo "不存在"; }
- Traverse the array
You can use a foreach loop to traverse all elements in the array. The following is a sample code:
$fruits = array("apple", "banana", "orange"); foreach ($fruits as $fruit) { echo $fruit . " "; } // 输出 "apple banana orange"
- Array operation function
PHP provides a wealth of array operation functions, which can sort, search, merge and other operations on arrays. The following are some examples of commonly used array operation functions:
// 数组排序 $numbers = [3, 1, 2]; sort($numbers); // 排序 print_r($numbers); // 输出 [1, 2, 3] // 数组查找 $fruits = array("apple", "banana", "orange"); $key = array_search("banana", $fruits); // 查找 "banana" echo $key; // 输出 1 // 数组合并 $fruits1 = array("apple", "banana"); $fruits2 = array("orange", "grape"); $merged = array_merge($fruits1, $fruits2); // 合并数组 print_r($merged); // 输出 ["apple", "banana", "orange", "grape"]
The above are some basic operations and functions about using array variables in PHP. By mastering the use of arrays, you can improve the flexibility and functionality of your PHP program.
The above is the detailed content of How to use array variables in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


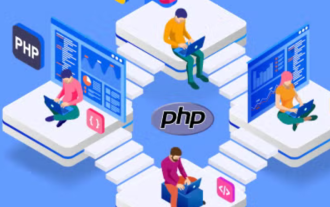
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
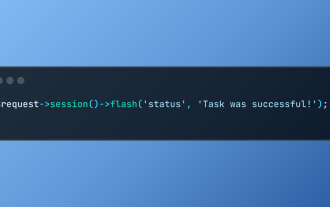
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
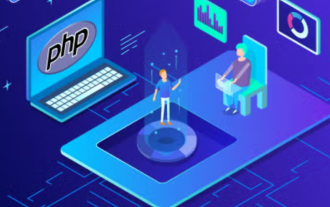
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
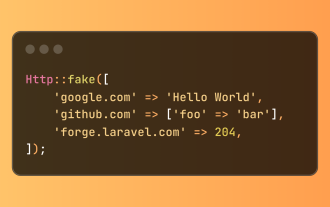
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
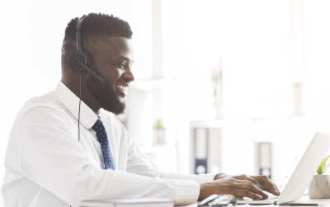
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
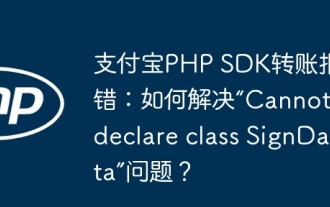
Alipay PHP...
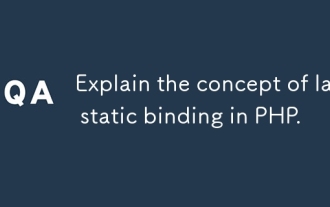
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
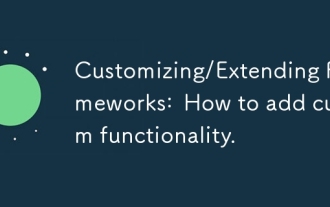
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
