


How to optimize PHP code and use closures, generators and reflection techniques to improve performance
How to optimize PHP code and use closures, generators and reflection techniques to improve performance
When writing PHP code, optimizing the code can improve performance and maintainability. This article will introduce how to optimize PHP code by using closures, generators and reflection techniques. At the same time, we will also provide specific code examples to help readers better understand the optimization process.
A closure is a special anonymous function that can save the environment variables when it was created. Using closures can reduce the use of global variables and improve code readability and maintainability. Here is a simple closure example:
function multiplyBy($number) { return function($value) use ($number) { return $number * $value; }; } $triple = multiplyBy(3); echo $triple(5); // 输出 15
In the above example, we defined a multiplyBy
function that returns a closure. The closure saves the environment variable $number
when the function is created and applies it to the parameter $value
when it is called. In this way, we can implement the multiplication operation by calling the closure.
A generator is a special function that can be iterated multiple times and returns a value through the yield
keyword. Generators can significantly reduce memory usage, especially when processing large amounts of data. Here is a simple generator example:
function generateNumbers($start, $end) { for ($i = $start; $i <= $end; $i++) { yield $i; } } foreach (generateNumbers(1, 1000000) as $number) { echo $number . ' '; }
In the above example, we defined a generateNumbers
generator function. By using the yield
keyword, a function can generate and return numbers on demand. In the foreach
loop, we use the generator to generate and output all numbers from 1 to 1000000.
Reflection is a technology used to analyze and modify the structure of PHP code. Through reflection, we can dynamically obtain and modify classes, properties, methods, etc. at runtime. Here is a simple reflection example:
class MyClass { public $name = 'John'; public function greet() { echo 'Hello, ' . $this->name; } } $reflectionClass = new ReflectionClass('MyClass'); $reflectionProperty = $reflectionClass->getProperty('name'); $reflectionProperty->setAccessible(true); $reflectionProperty->setValue(new MyClass(), 'Jane'); $reflectionMethod = $reflectionClass->getMethod('greet'); $reflectionMethod->invoke(new MyClass());
In the above example, we first create a MyClass
class, which has a public property name
and a public Methodgreet
. Then, we obtained the name
attribute and greet
method through reflection, and modified the value of the name
attribute and called greet
through reflection. method.
By using closures, generators, and reflection techniques, we can improve performance and maintainability when writing PHP code. Closures can help us reduce the use of global variables, generators can reduce memory usage, and reflection can dynamically obtain and modify code structures at runtime. I hope this article will be helpful to you in optimizing your PHP code.
The above is the detailed content of How to optimize PHP code and use closures, generators and reflection techniques to improve performance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
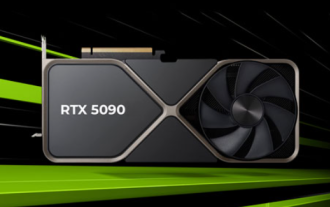
Many users are curious about the next-generation brand new RTX5090 graphics card. They don’t know how much the performance of this graphics card has been improved compared to the previous generation. Judging from the current information, the overall performance of this graphics card is still very good. Is the performance improvement of RTX5090 obvious? Answer: It is still very obvious. 1. This graphics card has an acceleration frequency beyond the limit, up to 3GHz, and is also equipped with 192 streaming multiprocessors (SM), which may even generate up to 520W of power. 2. According to the latest news from RedGamingTech, NVIDIARTX5090 is expected to exceed the 3GHz clock frequency, which will undoubtedly play a greater role in performing difficult graphics operations and calculations, providing smoother and more realistic games.
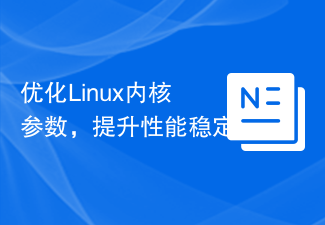
How to optimize and adjust the kernel parameters of Linux systems to improve performance and stability Summary: Linux is an operating system widely used in various servers and workstations, and the optimization of its performance and stability is crucial to providing efficient and reliable services. This article will introduce how to improve system performance and stability by optimizing and adjusting the kernel parameters of the Linux system. Keywords: Linux system, kernel parameters, performance optimization, stability Introduction: Linux, as an open source operating system, is widely used in various servers and work
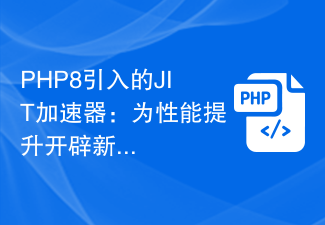
PHP8's JIT accelerator: ushering in a new era of performance improvement With the development of the Internet and the advancement of technology, the response speed of web pages has become one of the important indicators of user experience. As a widely used server-side scripting language, PHP has always been loved by developers for its simplicity, ease of learning and powerful functions. However, when processing large and complex business logic, PHP's performance often encounters bottlenecks. To solve this problem, PHP8 introduces a brand new feature: JIT (just in time compilation) accelerator. JIT accelerator is PHP8
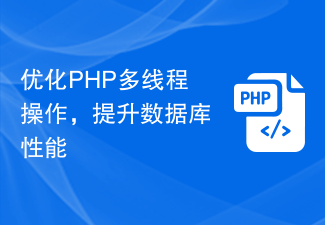
How to improve database read and write performance through PHP multi-threading. With the rapid development of the Internet, database read and write performance has become a key issue. When our application needs to frequently read and write to the database, using a single-threaded approach often leads to performance bottlenecks. The use of multi-threading can improve the efficiency of database reading and writing, thereby improving overall performance. As a commonly used server-side scripting language, PHP has flexible syntax and powerful database operation capabilities. This article will introduce how to use PHP multi-threading technology to improve
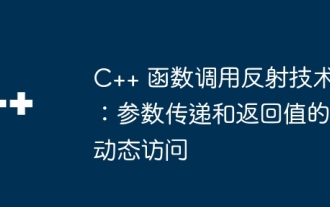
C++ function call reflection technology allows dynamically obtaining function parameter and return value information at runtime. Use typeid(decltype(...)) and decltype(...) expressions to obtain parameter and return value type information. Through reflection, we can dynamically call functions and select specific functions based on runtime input, enabling flexible and scalable code.
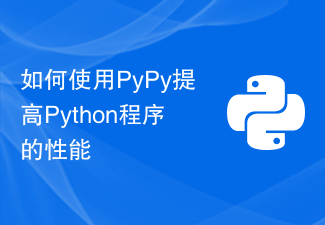
How to use PyPy to improve the performance of Python programs Introduction: Python, as a high-level programming language, is simple, easy to read, and easy to learn, so it has been widely used. However, Python also has the problem of slow running speed due to its interpreted execution characteristics. To solve this problem, PyPy came into being. This article will introduce how to use PyPy to improve the performance of Python programs. 1. What is PyPy? PyPy is a just-in-time compiled Python interpreter
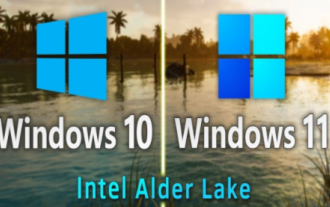
Presumably everyone's computer system has been updated to win11, so what are the advantages and disadvantages of win11 system compared to win10 system? This is what everyone wants to know. Let's take a look at the specific advantages and disadvantages together. What are the advantages of win11 over win10: 1. Smoothness. Win11 is better than win10 in terms of single-threaded and multi-threaded 3D operation. However, the response speed of win11 is relatively slow, and you need to wait for a while after clicking. 2. The performance of games is better than win10, and the average frame rate is also better than win10. However, the memory optimization is poor, the memory and CPU consumption are much higher than win10.3, and the operation interface uses too many rounded corners. Desktop ui mining
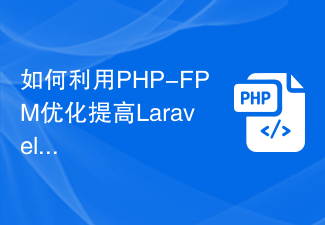
How to use PHP-FPM optimization to improve the performance of Laravel applications Overview: Laravel is a popular PHP framework that adopts modern design concepts and elegant syntax to enable developers to build web applications efficiently. However, performance issues may arise when handling a large number of concurrent requests. This article will introduce how to use PHP-FPM to optimize and improve the performance of Laravel applications. 1. What is PHP-FPM? PHP-FPM (FastCGIProce
