Mapping CSV to JavaBeans using OpenCSV
CSV files are basically plain text files that store data in comma-separated columns. OpenCSV is the library for parsing these CSV files, which are otherwise difficult to process. It is a very easy-to-use library that supports multiple functions such as reading and writing CSV files with headers.
In this article, we will discuss the process of mapping CSV files to JavaBeans through OpenCSV. Additionally, OpenCSV is a helpful tool in this process.
Mapping CSV to JavaBean
The OpenCSV library provides some classes and mapping strategies for mapping CSV files to Java Beans. One of the classes is CSVToBean, which is used to map CSV files to JavaBeans. To parse these CSV files, the CSVToBean class needs a mapping strategy defined and passed to the CSVToBean class. One of the popular mapping strategies is HeaderColumnNameTranslateMappingStrategy, which maps column IDs to Java Bean properties.
grammar
The mapping of CSV files to Beans is completed through a series of steps. However, the syntax for creating a HashMap with a mapping between column IDs and beam properties is as follows -
HashMap map = new HashMap(); map.put("column_id", "bean_property");
First, we create a hashmap, and then use the put() function of HashMap to map the column id with the corresponding Java bean property.
algorithm
Step One - First add OpenCSV to your Java project using the following dependencies.
For Maven projects, add the following dependencies to the java project -
<dependency> <groupId>com.opencsv</groupId> <artifactId>opencsv</artifactId> <version>4.1</version> </dependency>
For a Gradle project, you need to add the following dependencies -
compile group: 'com.opencsv', name: 'opencsv', version: '4.1'
Step 2 - Now, let’s start the basic steps of mapping csv file to Java Bean.
Step 3 - Create a HashMap with a mapping between column IDs and bean properties.
Step 4 - Add all the column ids of the csv file corresponding to the bean properties.
Step 5 − Create HeaderColumnNameTranslateMappingStrategy object.
Step 6 - Now, pass the mapped hashmap to the setColumnMapping() method.
Step 7 - Call objects of CSVToBean and CSVReader classes.
Step 8 - Now, we will call the parse method of the CSVToBean class and pass it the HeaderColumnNameTranslateMappingStrategy and CSVReader objects.
Step 9 - Print the details of the Bean object.
method
Now, let us use OpenCSV to map the contents of the Employee.csv file to JavaBeans. The Employee.csv file contains data such as employee name, department, and salary.
The contents of the Employee.csv file are as follows:
Employee_Name, Department, Salary Naman, Human Resource, 45000 Nikita, Sales, 35000 Rocky, IT, 50000 Raman, Human Resource, 42000
Now, let us first create the Employee class and then create the main method that maps the contents of that csv file to JavaBeans.
Example: Employee.java
public class Employee { private static final long serialVersionUID = 1L; public String emp_name, department, salary; public String getName() { return emp_name; } public void setName(String n) { emp_name = n; } public String getSalary() { return salary; } public void setSalary(String s) { salary = s; } public String getDepartment() { return department; } public void setDepartment(String d) { d = department; } public String toString() { return "Employee [Name=" + emp_name + ", Department= " + department +", Salary = " + salary+ "]"; } }
The following is the program code of the CsvToBean.java file.
Example
import java.util.*; import com.opencsv.CSVReader; import com.opencsv.bean.CsvToBean; import com.opencsv.bean.HeaderColumnNameTranslateMappingStrategy; public class csvtobean { public static void main(String[] args) { Map<String, String> map = new HashMap<>(); map.put("Employee_Name", "emp_name"); map.put("Department", "department"); map.put("Salary", "salary"); HeaderColumnNameTranslateMappingStrategy<Employee> s = new HeaderColumnNameTranslateMappingStrategy<>(); s.setType(Employee.class); s.setColumnMapping(map); CSVReader csvReader = null; try { csvReader = new CSVReader(new FileReader ("D:\CSVFiles\Employee.csv")); } catch (FileNotFoundException e) { e.printStackTrace(); } CsvToBean csvToBean = new CsvToBean(); List<Employee> l = csvToBean.parse(s, csvReader); for (Employee x : l) { System.out.println(x); } } }
Output
Employee [Name=Naman, Department=Human Resource, Salary=45000] Employee [Name=Nikita, Department=Sales, Salary=35000] Employee [Name=Rocky, Department=IT, Salary=50000] Employee [Name=Raman, Department=Human Resource, Salary=42000]
As you can see in the above program code, we first create a hash map that maps column IDs to corresponding bean properties. We then implemented the HeaderColumnNameTranslateMappingStrategy strategy for the Employee class and passed it to the parse method of the CsvToBean class to map the CSV to JavaBean using OpenCSV.
in conclusion
In this article, we looked at how to map CSV files to JavaBeans using OpenCSV. The simple technique discussed to do this is to use the CsvToBean class and a mapping strategy for the objects passed to the CsvToBean class. We discussed the steps and program code to parse employee data in csv format into JavaBeans using OpenCSV.
The above is the detailed content of Mapping CSV to JavaBeans using OpenCSV. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


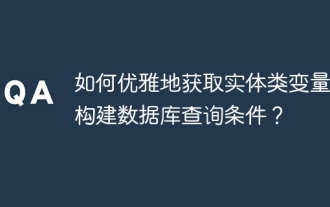
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
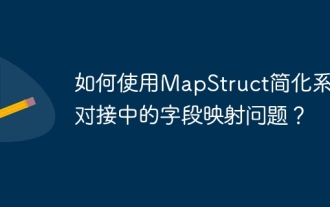
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
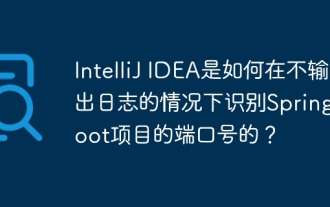
Start Spring using IntelliJIDEAUltimate version...
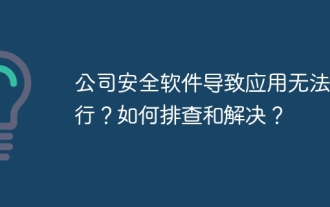
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
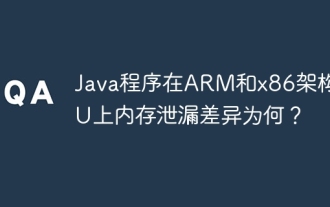
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
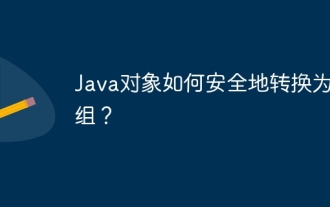
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
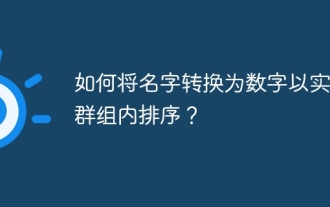
How to convert names to numbers to implement sorting within groups? When sorting users in groups, it is often necessary to convert the user's name into numbers so that it can be different...
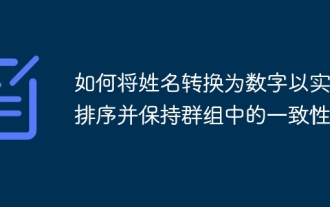
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
